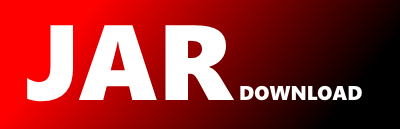
org.etlunit.HtmlDiffManagerImpl Maven / Gradle / Ivy
package org.etlunit;
import org.etlunit.feature.logging.LogFileManager;
import org.etlunit.parser.ETLTestMethod;
import org.etlunit.parser.ETLTestOperation;
import org.etlunit.util.IOUtils;
import org.etlunit.util.StringUtils;
import org.etlunit.util.VelocityUtil;
import javax.inject.Inject;
import java.io.File;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class HtmlDiffManagerImpl implements DiffManagerImpl
{
File reportsDir;
private LogFileManager logFileManager;
@Inject
public void receiveLogFileManager(LogFileManager logFileManager)
{
this.logFileManager = logFileManager;
}
public class MethodDiff
{
private final ETLTestMethod method;
private final List diffList = new ArrayList();
public MethodDiff(ETLTestMethod method)
{
this.method = method;
}
public ETLTestMethod getMethod()
{
return method;
}
public List getDiffList()
{
return diffList;
}
}
public class OperationDiffGrid
{
private final ETLTestOperation operation;
private final HtmlDiffGridImpl diffGrid;
private final String failureId;
public OperationDiffGrid(ETLTestOperation method, HtmlDiffGridImpl list, String failureId)
{
this.operation = method;
diffGrid = list;
this.failureId = failureId;
}
public ETLTestOperation getOperation()
{
return operation;
}
public String getFailureId()
{
return failureId;
}
public HtmlDiffGridImpl getDiffGrid()
{
return diffGrid;
}
}
private final Map diffMap = new HashMap();
@Override
public void setOutputDirectory(File file)
{
reportsDir = file;
}
@Override
public void dispose()
{
// purge reports dir before proceeding
IOUtils.purge(reportsDir);
try
{
// grab the velocity template for the report
URL url = getClass().getClassLoader().getResource("htmlDiffReport.vm");
String template = IOUtils.readURLToString(url);
// for each method, prepare a diff html file and save in the reports folder
for (Map.Entry entry : diffMap.entrySet())
{
ETLTestMethod method = entry.getKey();
// open the report file
File target = new File(reportsDir, method.getQualifiedName() + "_diff_report.html");
// process the template with the grid list
String reportText = VelocityUtil.writeTemplate(template, entry.getValue());
// persist the report
IOUtils.writeBufferToFile(target, new StringBuffer(reportText));
}
}
catch (Exception e)
{
throw new RuntimeException("Error writing diff report files", e);
}
}
@Override
public DiffGrid reportDiff(ETLTestOperation operation, String failureId)
{
HtmlDiffGridImpl htmlDiffGrid = new HtmlDiffGridImpl(operation.getTestMethod());
MethodDiff methodDiff = diffMap.get(operation.getTestMethod());
if (methodDiff == null)
{
methodDiff = new MethodDiff(operation.getTestMethod());
diffMap.put(operation.getTestMethod(), methodDiff);
}
OperationDiffGrid odg = new OperationDiffGrid(operation, htmlDiffGrid, failureId);
methodDiff.diffList.add(odg);
return htmlDiffGrid;
}
@Override
public DataSetGrid reportDataSet(ETLTestOperation method, List columns, String failureId)
{
throw new UnsupportedOperationException();
}
public final class HtmlDiffGridImpl implements DiffGrid
{
private final ETLTestMethod method;
private final List rows = new ArrayList();
public HtmlDiffGridImpl(ETLTestMethod method)
{
this.method = method;
}
@Override
public DiffGridRow addRow(int sourceLine, int targetLine, line_type type)
{
HtmlDiffGridRowImpl gridRow = new HtmlDiffGridRowImpl(sourceLine, targetLine, type);
rows.add(gridRow);
return gridRow;
}
public List getRows()
{
return rows;
}
@Override
public void done()
{
}
}
public final class HtmlDiffGridRowImpl implements DiffGridRow
{
private final int sourceRecordNum;
private final int targetRecordNum;
private final DiffGrid.line_type lineType;
private String columnName;
private String sourceValue;
private String targetValue;
private String orderKey;
public HtmlDiffGridRowImpl(int sourceRecordNum, int targetRecordNum, DiffGrid.line_type lineType)
{
this.sourceRecordNum = sourceRecordNum;
this.targetRecordNum = targetRecordNum;
this.lineType = lineType;
}
@Override
public void setColumnName(String col)
{
columnName = col;
}
@Override
public void setOrderKey(String key)
{
orderKey = key;
}
@Override
public void setSourceValue(String value)
{
sourceValue = value;
}
@Override
public void setTargetValue(String value)
{
targetValue = value;
}
public int getSourceRecordNum()
{
return sourceRecordNum;
}
public int getTargetRecordNum()
{
return targetRecordNum;
}
public DiffGrid.line_type getLineType()
{
return lineType;
}
public String getOrderKey()
{
return orderKey;
}
public String getOrderKeyEncoded()
{
return StringUtils.encodeNonPrintable(getOrderKey());
}
public String getColumnName()
{
return columnName;
}
public String getSourceValue()
{
return sourceValue;
}
public String getTargetValue()
{
return targetValue;
}
public String getSourceValueEncoded()
{
return StringUtils.encodeNonPrintable(getSourceValue());
}
public String getTargetValueEncoded()
{
return StringUtils.encodeNonPrintable(getTargetValue());
}
@Override
public void done()
{
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy