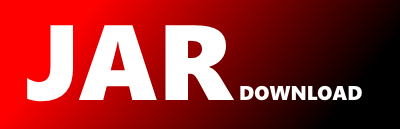
org.etlunit.feature.extend.ExtensibleFeatureModule Maven / Gradle / Ivy
package org.etlunit.feature.extend;
import org.etlunit.*;
import org.etlunit.context.VariableContext;
import org.etlunit.feature.*;
import org.etlunit.json.validator.JsonSchema;
import org.etlunit.parser.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
@FeatureModule
public abstract class ExtensibleFeatureModule extends AbstractFeature
{
private final
List
extenders =
new ArrayList();
@Override
public final ClassListener getListener()
{
return new ClassListener()
{
private final ClassListener classListener = getDelegateListener();
@Override
public void begin(ETLTestClass cl, final VariableContext context)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
if (classListener != null)
{
classListener.begin(cl, context);
}
}
@Override
public void declare(ETLTestVariable var, final VariableContext context)
{
if (classListener != null)
{
classListener.declare(var, context);
}
}
@Override
public void begin(ETLTestMethod mt, final VariableContext context)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
if (classListener != null)
{
classListener.begin(mt, context);
}
}
@Override
public void begin(ETLTestOperation op, ETLTestValueObject parameters, VariableContext vcontext, ExecutionContext econtext)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
if (classListener != null)
{
classListener.begin(op, parameters, vcontext, econtext);
}
}
@Override
public action_code process(ETLTestOperation op, ETLTestValueObject obj, VariableContext context, ExecutionContext econtext)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
if (handles(op, obj))
{
return FeatureListenerProxy.processListenerList(extenders, op, obj, context, econtext, applicationLog);
}
return action_code.defer;
}
@Override
public void end(ETLTestOperation op, ETLTestValueObject parameters, VariableContext vcontext, ExecutionContext econtext)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
if (classListener != null)
{
classListener.end(op, parameters, vcontext, econtext);
}
}
@Override
public void end(ETLTestMethod mt, final VariableContext context)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
if (classListener != null)
{
classListener.end(mt, context);
}
}
@Override
public void end(ETLTestClass cl, final VariableContext context)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
if (classListener != null)
{
classListener.end(cl, context);
}
}
};
}
@Override
public FeatureMetaInfo getMetaInfo()
{
final FeatureMetaInfo meta = super.getMetaInfo();
return new FeatureMetaInfo()
{
@Override
public String getFeatureConfiguration()
{
return meta.getFeatureConfiguration();
}
@Override
public JsonSchema getFeatureConfigurationValidator()
{
return meta.getFeatureConfigurationValidator();
}
@Override
public Map getExportedOperations()
{
return meta.getExportedOperations();
}
@Override
public Map getExportedAnnotations()
{
return meta.getExportedAnnotations();
}
public List getOperationValidator(String operation)
{
List list = new ArrayList();
// check with each validator
for (FeatureListenerProxy.FeatureOperationProcessor extender : extenders)
{
Feature feature = extender.getFeature();
if (feature != ExtensibleFeatureModule.this)
{
List schema = feature.getMetaInfo().getExportedOperations().get(operation).getValidator();
if (schema != null)
{
list.addAll(schema);
}
}
}
return list;
}
@Override
public boolean isInternalFeature()
{
return meta.isInternalFeature();
}
@Override
public String getFeatureUsage()
{
return meta.getFeatureUsage();
}
@Override
public List getOptions()
{
return Collections.emptyList();
}
};
}
public final void extend(final Extender exe)
{
extenders.add(new FeatureListenerProxy.FeatureOperationProcessor()
{
@Override
public boolean handlesRequest(ETLTestOperation operation, ETLTestValueObject operands, VariableContext context, ExecutionContext econtext)
{
return exe.canHandleRequest(operation, operands, context, econtext);
}
@Override
public Feature getFeature()
{
return exe.getFeature();
}
@Override
public OperationProcessor getOperationProcessor()
{
return exe;
}
});
}
protected abstract boolean handles(ETLTestOperation op, ETLTestValueObject obj);
protected ClassListener getDelegateListener()
{
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy