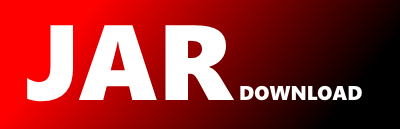
org.etlunit.feature.logging.LoggingFeatureModule Maven / Gradle / Ivy
package org.etlunit.feature.logging;
import org.etlunit.*;
import org.etlunit.context.VariableContext;
import org.etlunit.feature.AbstractFeature;
import org.etlunit.feature.Feature;
import org.etlunit.feature.FeatureLogListenerProxy;
import org.etlunit.feature.FeatureModule;
import org.etlunit.io.FileBuilder;
import org.etlunit.parser.ETLTestClass;
import org.etlunit.parser.ETLTestMethod;
import org.etlunit.parser.ETLTestOperation;
import org.etlunit.parser.ETLTestValueObject;
import org.etlunit.util.IOUtils;
import org.etlunit.util.StringUtils;
import java.io.*;
import java.util.List;
@FeatureModule
public class LoggingFeatureModule extends AbstractFeature
{
private RuntimeSupport runtimeSupport;
private Log applicationLog = new PrintWriterLog();
private final LogListenerLogProxy logListenerLogProxy;
private final boolean echo;
public LoggingFeatureModule(RuntimeSupport runtimeSupport, List features)
{
this.runtimeSupport = runtimeSupport;
// before logging anything, clear the log folder
IOUtils.purge(getLogDirectory());
logListenerLogProxy = new LogListenerLogProxy(new FeatureLogListenerProxy(features));
echo = System.getProperty("org.etlunit.feature.logging.echo") != null;
}
public LogListenerLogProxy getLog()
{
return logListenerLogProxy;
}
@Override
public ClassListener getListener()
{
return new NullClassListener()
{
@Override
public void begin(ETLTestClass cl, VariableContext context)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
logListenerLogProxy.begin(cl, context);
}
@Override
public void begin(ETLTestMethod mt, VariableContext context)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
logListenerLogProxy.begin(mt, context);
}
@Override
public void begin(ETLTestOperation op, ETLTestValueObject parameters, VariableContext vcontext, ExecutionContext econtext)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
logListenerLogProxy.begin(op, parameters, vcontext, econtext);
}
@Override
public void end(ETLTestOperation op, ETLTestValueObject parameters, VariableContext vcontext, ExecutionContext econtext)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
logListenerLogProxy.end(op, parameters, vcontext, econtext);
}
@Override
public void end(ETLTestMethod mt, VariableContext context)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
logListenerLogProxy.end(mt, context);
}
@Override
public void end(ETLTestClass cl, VariableContext context)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
logListenerLogProxy.end(cl, context);
}
@Override
public action_code process(ETLTestOperation op, ETLTestValueObject obj, final VariableContext context, ExecutionContext econtext)
throws TestAssertionFailure, TestExecutionError, TestWarning
{
if (op.getOperationName().equals("log"))
{
applicationLog.info(obj.getValueAsMap().get("message").getValueAsString());
return action_code.handled;
}
return action_code.defer;
}
};
}
public File getLogDirectory()
{
return new FileBuilder(runtimeSupport.getTempDirectory()).subdir("log").mkdirs().file();
}
@Override
public LogListener getLogListener()
{
return new LogListener()
{
@Override
public void log(message_type type, String text, ETLTestClass etlClass, ETLTestMethod method, ETLTestOperation operation)
{
log(type, null, text, etlClass, method, operation);
}
@Override
public void log(message_type type, Throwable error, String text, ETLTestClass etlClass, ETLTestMethod method, ETLTestOperation operation)
{
String name = "application.log";
if (etlClass != null)
{
name = etlClass.getQualifiedName() + ".txt";
}
File log = new FileBuilder(getLogDirectory()).mkdirs().name(name).file();
StringBuilder header = new StringBuilder("[");
switch (type)
{
case debug:
header.append("DBUG");
break;
case info:
header.append("INFO");
break;
case severe:
header.append("SEVR");
break;
}
if (etlClass != null)
{
header.append(".");
header.append(etlClass.getQualifiedName());
if (method != null)
{
header.append(".");
header.append(method.getName());
if (operation != null)
{
header.append(".");
header.append(operation.getOperationName());
}
}
}
header.append("]");
StringBuilder tbuilder = new StringBuilder(text);
if (error != null)
{
StringWriter swriter = new StringWriter();
PrintWriter pwriter = new PrintWriter(swriter);
error.printStackTrace(pwriter);
pwriter.flush();
pwriter.close();
tbuilder.append("\n").append(swriter.toString());
}
String
logEntry =
StringUtils.prepareForLog(tbuilder.toString().replace("\r\n", "\n").replace("\r", "\n"), header.toString());
if (echo)
{
System.out.println(logEntry);
}
try
{
PrintWriter writer = new PrintWriter(new FileWriter(log, true), true);
try
{
writer.println(logEntry);
}
finally
{
writer.close();
}
}
catch (IOException exc)
{
exc.printStackTrace();
}
}
};
}
@Override
public String getFeatureName()
{
return "logging";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy