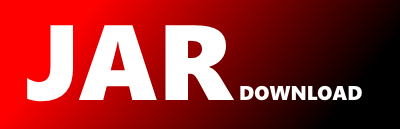
org.etlunit.io.FileBuilder Maven / Gradle / Ivy
package org.etlunit.io;
import java.io.File;
public class FileBuilder
{
private File path;
public FileBuilder(File path)
{
this.path = path;
}
public FileBuilder(String path)
{
this.path = new File(path);
}
public FileBuilder()
{
this.path = new File("");
}
public FileBuilder subdir(String name)
{
path = new File(path, name);
return this;
}
public FileBuilder name(String name)
{
path = new File(path, name);
return this;
}
public FileBuilder mkdirs()
{
if (!path.exists())
{
if (!path.mkdirs())
{
throw new RuntimeException("Could not make directory: " + path.getAbsolutePath());
}
}
else if (!path.isDirectory())
{
throw new RuntimeException("Path is not a directory: " + path.getAbsolutePath());
}
return this;
}
public FileBuilder mkdirsToFile()
{
new FileBuilder(path.getParentFile()).mkdirs();
return this;
}
public File file()
{
return path;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy