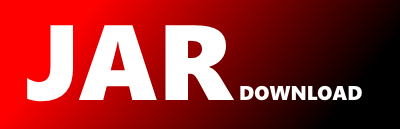
org.etlunit.io.NfurcatingOutputStream Maven / Gradle / Ivy
package org.etlunit.io;
import java.io.IOException;
import java.io.OutputStream;
public class NfurcatingOutputStream extends OutputStream
{
private final OutputStream stream;
private final Object LOCK = new Object();
private abstract class LockRunnable
{
public void run() throws IOException
{
synchronized (LOCK)
{
runLocked(stream);
}
}
protected abstract void runLocked(OutputStream stream) throws IOException;
}
public NfurcatingOutputStream(OutputStream stream)
{
this.stream = stream;
}
@Override
public void write(final int b) throws IOException
{
new LockRunnable()
{
@Override
protected void runLocked(OutputStream stream1) throws IOException
{
stream1.write(b);
}
}.run();
}
@Override
public void write(final byte[] b) throws IOException
{
new LockRunnable()
{
@Override
protected void runLocked(OutputStream stream1) throws IOException
{
stream1.write(b);
}
}.run();
}
@Override
public void write(final byte[] b, final int off, final int len) throws IOException
{
new LockRunnable()
{
@Override
protected void runLocked(OutputStream stream1) throws IOException
{
stream1.write(b, off, len);
}
}.run();
}
@Override
public void flush() throws IOException
{
new LockRunnable()
{
@Override
protected void runLocked(OutputStream stream1) throws IOException
{
stream1.flush();
}
}.run();
}
@Override
public void close() throws IOException
{
new LockRunnable()
{
@Override
protected void runLocked(OutputStream stream1) throws IOException
{
stream1.close();
}
}.run();
}
public OutputStream bifurcate(final OutputStream target)
{
return new BifurcatingOutputStream(this, target)
{
@Override
public void close() throws IOException
{
target.close();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy