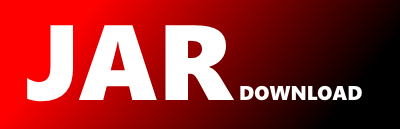
org.etlunit.io.file.SchemaColumn Maven / Gradle / Ivy
package org.etlunit.io.file;
import org.codehaus.jackson.JsonNode;
class SchemaColumn implements DataFileSchema.Column
{
private final String id;
private String type;
private basic_type basicType = basic_type.string;
private int length;
private final DataFileManager dataFileManager;
private int offset = -1;
public SchemaColumn(String id, int length, DataFileManager dataFileManager)
{
this(id, null, length, null, dataFileManager);
}
public SchemaColumn(String id, String type, DataFileManager dataFileManager)
{
this(id, type, -1, null, dataFileManager);
}
public SchemaColumn(String id, String type, int length, FlatFileType validator, DataFileManager dataFileManager)
{
this.id = id;
this.type = type;
this.length = length;
this.dataFileManager = dataFileManager;
}
public SchemaColumn(JsonNode node, DataFileManager dataFileManager)
{
this.dataFileManager = dataFileManager;
id = node.get("id").asText();
if (node.has("type"))
{
JsonNode type1 = node.get("type");
if (!type1.isNull())
{
type = type1.asText();
}
else
{
type = null;
}
}
else
{
type = null;
}
if (node.has("length"))
{
JsonNode length1 = node.get("length");
if (!length1.isNull())
{
length = length1.asInt();
}
else
{
length = -1;
}
}
else
{
length = -1;
}
if (node.has("basic-type"))
{
JsonNode length1 = node.get("basic-type");
if (!length1.isNull())
{
String bt = length1.asText();
if (bt.equals(basic_type.integer.name()))
{
basicType = basic_type.integer;
}
else if (bt.equals(basic_type.string.name()))
{
basicType = basic_type.string;
}
else if (bt.equals(basic_type.numeric.name()))
{
basicType = basic_type.numeric;
}
}
else
{
basicType = basic_type.string;
}
}
else
{
basicType = basic_type.string;
}
}
public boolean validateText(String text)
{
if (!hasType())
{
return true;
}
DataFormatValidator validator = dataFileManager.resolveValidatorById(type);
if (validator == null)
{
throw new IllegalArgumentException("Type " + type + " not resolved to a registered expression");
}
return validator.getPattern().matcher(text).matches();
}
public void setOffset(int offset)
{
this.offset = offset;
}
public String getId()
{
return id;
}
public boolean hasType()
{
return type != null;
}
public String getType()
{
return type;
}
@Override
public void setType(String type)
{
this.type = type;
}
@Override
public void setLength(int length)
{
if (this.length != -1)
{
throw new IllegalArgumentException("Cannot change the length of a column");
}
this.length = length;
}
public int getOffset()
{
return offset;
}
public int getLength()
{
return length;
}
public basic_type getBasicType()
{
return basicType;
}
public void setBasicType(basic_type basicType)
{
if (basicType == null)
{
throw new IllegalArgumentException("Basic type cannot be null");
}
this.basicType = basicType;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy