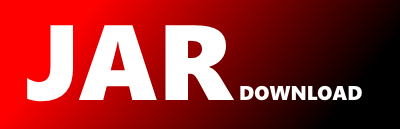
org.etlunit.parser.ETLTestClassImpl Maven / Gradle / Ivy
package org.etlunit.parser;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
public class ETLTestClassImpl extends ETLTestAnnotatedImpl implements ETLTestClass
{
private List classVariables = new ArrayList();
private final String _package;
private String className;
private List methodNames = new ArrayList();
private List suiteMemberships = new ArrayList();
private List suiteMembershipsPub = Collections.unmodifiableList(suiteMemberships);
private List testMethods = new ArrayList();
private List testMethodsPub = Collections.unmodifiableList(testMethods);
private List beforeClassMethods = new ArrayList();
private List beforeClassMethodsPub = Collections.unmodifiableList(beforeClassMethods);
private List afterClassMethods = new ArrayList();
private List afterClassMethodsPub = Collections.unmodifiableList(afterClassMethods);
private List beforeTestMethods = new ArrayList();
private List beforeTestMethodsPub = Collections.unmodifiableList(beforeTestMethods);
private List afterTestMethods = new ArrayList();
private List afterTestMethodsPub = Collections.unmodifiableList(afterTestMethods);
public ETLTestClassImpl(String _package)
{
this._package = _package;
}
public ETLTestClassImpl(String _package, Token t)
{
super(t);
this._package = _package;
}
public void addSuiteMembership(String s)
{
suiteMemberships.add(s.toLowerCase());
}
@Override
public List getClassVariables()
{
return classVariables;
}
@Override
public String getPackage()
{
return _package;
}
public void setName(String className)
{
this.className = className;
}
@Override
public String getName()
{
return className;
}
@Override
public String getQualifiedName()
{
return _package.equals("") ? (className) : (_package + "." + className);
}
public List getSuiteMemberships()
{
if (suiteMemberships.size() == 0 && hasAnnotation("@JoinSuite"))
{
List annList = getAnnotations("@JoinSuite");
Iterator annIt = annList.iterator();
while (annIt.hasNext())
{
ETLTestAnnotation ann = annIt.next();
suiteMemberships.add(ann.getValue().getValueAsMap().get("name").getValueAsString());
}
}
return suiteMembershipsPub;
}
public List getTestMethods()
{
return testMethodsPub;
}
public void addClassVariable(ETLTestVariable var)
{
classVariables.add(var);
}
public void addMethod(ETLTestMethod method)
{
if (methodNames.contains(method.getName()))
{
throw new IllegalArgumentException("method name "
+ getName()
+ "."
+ method.getName()
+ " specified more than once");
}
if (!method.requiresPurge() && testMethods.size() == 0)
{
throw new IllegalArgumentException("First test method does not support @DoNotPurge annotation");
}
methodNames.add(method.getName());
if (method.hasAnnotation("@BeforeClass"))
{
beforeClassMethods.add(method);
}
if (method.hasAnnotation("@AfterClass"))
{
afterClassMethods.add(method);
}
if (method.hasAnnotation("@BeforeTest"))
{
beforeTestMethods.add(method);
}
if (method.hasAnnotation("@AfterTest"))
{
afterTestMethods.add(method);
}
if (method.hasAnnotation("@Test"))
{
testMethods.add(method);
}
}
public List getBeforeClassMethods()
{
return beforeClassMethodsPub;
}
public List getAfterClassMethods()
{
return afterClassMethodsPub;
}
public List getBeforeTestMethods()
{
return beforeTestMethodsPub;
}
public List getAfterTestMethods()
{
return afterTestMethodsPub;
}
/**
* Ordering is specified as the alpha comparison of the package name first (null package is first),
* then the alpha of the class name
* This won't necessarily match the file names, but will make the test ordering predictable
*
* @param o
* @return
*/
@Override
public int compareTo(ETLTestClass o)
{
// check null package first
if (getPackage() == null && o.getPackage() != null)
{
return -1;
}
else if (getPackage() != null && o.getPackage() == null)
{
return 1;
}
// both packages not null. Check package names
int packComp = getPackage().compareTo(o.getPackage());
if (packComp != 0)
{
// return package ordering
return packComp;
}
// defer to the class name
return getName().compareTo(o.getName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy