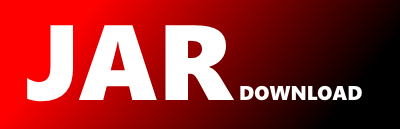
org.etlunit.parser.ETLTestOperationImpl Maven / Gradle / Ivy
package org.etlunit.parser;
public class ETLTestOperationImpl extends ETLTestDebugTraceableImpl implements ETLTestOperation
{
private final String operationName;
private final int ordinal;
private int siblingOrdinal = 1;
private final ETLTestValueObject operands;
private final ETLTestMethod method;
public ETLTestOperationImpl(ETLTestMethod pMethod, String name, int ordinal)
{
this(pMethod, name, ordinal, null, null);
}
public ETLTestOperationImpl(ETLTestMethod pMethod, String name, int ordinal, Token t)
{
this(pMethod, name, ordinal, null, t);
}
public ETLTestOperationImpl(ETLTestMethod pMethod, String name, int ordinal, ETLTestValueObject op)
{
method = pMethod;
operationName = name;
operands = op;
this.ordinal = ordinal;
}
public ETLTestOperationImpl(ETLTestMethod pMethod, String name, int ordinal, ETLTestValueObject op, Token t)
{
super(t);
method = pMethod;
operationName = name;
operands = op;
this.ordinal = ordinal;
}
public String getOperationName()
{
return operationName;
}
@Override
public ETLTestValueObject getOperands()
{
return operands;
}
private String list()
{
return String.valueOf(operands);
}
public String description()
{
return method.getName() + "." + operationName + "(" + list() + ")";
}
@Override
public ETLTestMethod getTestMethod()
{
return method;
}
@Override
public ETLTestClass getTestClass()
{
return getTestMethod().getTestClass();
}
@Override
public ETLTestOperation createSibling(String name)
{
return new ETLTestOperationImpl(getTestMethod(), name, (siblingOrdinal++ * 10000) + ordinal, token);
}
@Override
public ETLTestOperation createSibling(String name, ETLTestValueObject parameters)
{
return new ETLTestOperationImpl(getTestMethod(), name, (siblingOrdinal++ * 10000) + ordinal, parameters, token);
}
@Override
public String getQualifiedName()
{
return method.getQualifiedName() + "." + getOperationName() + "." + getOrdinal();
}
@Override
public int getOrdinal()
{
return ordinal;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy