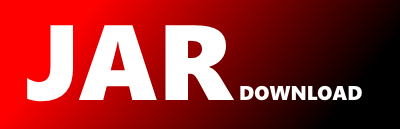
org.etlunit.util.Hex Maven / Gradle / Ivy
package org.etlunit.util;
public class Hex
{
public static String hexEncode(byte[] bytes)
{
StringBuffer buffer = new StringBuffer();
for (int i = 0; i < bytes.length; i++)
{
int b = (int) bytes[i] & 0xFF;
buffer.append(makeHex(b / 0X10));
buffer.append(makeHex(b % 0X10));
}
return buffer.toString();
}
public static char makeHex(int b)
{
switch (b)
{
case 0x0:
return '0';
case 0x1:
return '1';
case 0x2:
return '2';
case 0x3:
return '3';
case 0x4:
return '4';
case 0x5:
return '5';
case 0x6:
return '6';
case 0x7:
return '7';
case 0x8:
return '8';
case 0x9:
return '9';
case 0xa:
return 'A';
case 0xb:
return 'B';
case 0xc:
return 'C';
case 0xd:
return 'D';
case 0xe:
return 'E';
case 0xf:
return 'F';
default:
throw new IllegalArgumentException("Bad char: " + b);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy