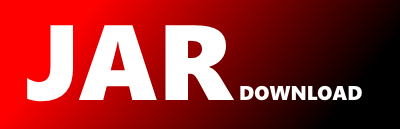
org.etlunit.util.IOUtils Maven / Gradle / Ivy
package org.etlunit.util;
import org.etlunit.TestAssertionFailure;
import org.etlunit.TestContext;
import java.io.*;
import java.net.URL;
import java.util.Arrays;
import java.util.Map;
import java.util.concurrent.Semaphore;
import java.util.zip.CRC32;
import java.util.zip.CheckedInputStream;
public class IOUtils
{
public static String hashToLength(String s, int i)
{
if (s.length() < i)
{
return s;
}
s = s.replaceAll("a|e|i|o|u|A|E|I|O|U", "");
if (s.length() < i)
{
return s;
}
s = s.replaceAll("_|-", "");
return s;
}
public static interface LineVisitor
{
String visit(String line, int lineNo);
}
public static void copyFiles(File dsrc, File gtrg) throws IOException
{
copyFiles(dsrc, gtrg, false);
}
public static void copyFiles(File dsrc, File gtrg, boolean append) throws IOException
{
BufferedInputStream bin = new BufferedInputStream(new FileInputStream(dsrc));
try
{
BufferedOutputStream bout = new BufferedOutputStream(new FileOutputStream(gtrg, append));
try
{
copyStream(bin, bout);
}
finally
{
bout.close();
}
}
finally
{
bin.close();
}
}
public static void copyStream(InputStream in, OutputStream out) throws IOException
{
byte[] buff = new byte[1024 * 1024];
int i = 0;
while ((i = in.read(buff)) != -1)
{
if (out != null)
{
out.write(buff, 0, i);
}
}
}
public static String readFileToString(File f) throws IOException
{
StringWriter swriter = new StringWriter();
Reader reader = new BufferedReader(new FileReader(f));
try
{
char[] buff = new char[1024 * 1024];
int res = 0;
while ((res = reader.read(buff)) != -1)
{
swriter.write(buff, 0, res);
}
}
finally
{
reader.close();
}
return swriter.toString();
}
public static void compareFiles(File dsrc, File gtrg) throws Exception
{
// run through line by line and compare results
BufferedReader srcReader = new BufferedReader(new FileReader(dsrc), 65536);
try
{
BufferedReader trgReader = new BufferedReader(new FileReader(gtrg), 65536);
try
{
String srcLine = null;
String trgLine = null;
while (true)
{
srcLine = srcReader.readLine();
trgLine = trgReader.readLine();
if (srcLine == null && trgLine == null)
{
break;
}
else if (srcLine == null)
{
throw new TestAssertionFailure("Files do not match. Target has more rows. First extra row in target: "
+ trgLine);
}
else if (trgLine == null)
{
throw new TestAssertionFailure("Files do not match. Source has more rows. First extra row in source: "
+ srcLine);
}
else if (!srcLine.equals(trgLine))
{
throw new TestAssertionFailure(
"Files do not match. Input rows do not match. First non-matching row in source: >>"
+ srcLine
+ "<< target: >>"
+ trgLine
+ "<<");
}
}
}
finally
{
trgReader.close();
}
}
finally
{
srcReader.close();
}
}
public static boolean checksumFiles(File dsrc, File gtrg) throws Exception
{
return checkSumFile(dsrc).getValue() == checkSumFile(gtrg).getValue();
}
public static CRC32 checkSumFile(File dsrc) throws Exception
{
InputStream in = new BufferedInputStream(new FileInputStream(dsrc), 1024 * 1024);
try
{
return checkSumStream(in);
}
finally
{
in.close();
}
}
public static CRC32 checkSumStream(InputStream inst) throws Exception
{
CRC32 crc = new CRC32();
CheckedInputStream cis = new CheckedInputStream(inst, crc);
copyStream(cis, null);
return crc;
}
public static int exec(String[] args, File root, TestContext context) throws Exception
{
return exec(args, null, root, context);
}
public static int exec(String[] args, File root, TestContext context, StringBuffer stdoutBuffer) throws Exception
{
return exec(args, null, root, context, stdoutBuffer);
}
public static int exec(String[] args, Map env, File root, TestContext context) throws Exception
{
return exec(args, env, root, context, null);
}
public static int exec(String[] args, Map env, File root, TestContext context, StringBuffer stdoutBuffer)
throws Exception
{
context.writeToProcessLog("Executing " + Arrays.asList(args));
if (context != null)
{
context.writeToProcessLog(args);
}
ProcessBuilder pb = new ProcessBuilder();
pb.redirectErrorStream(true);
pb.command(args).directory(root);
if (env != null)
{
pb.environment().putAll(env);
}
Process p = pb.start();
context.writeToProcessLog("Process started");
Streamer s = new Streamer(p.getInputStream(), context, stdoutBuffer);
s.start();
int rc = p.waitFor();
s.waitForFlush();
return rc;
}
public static void writeBufferToFile(File file, StringBuffer buff) throws IOException
{
writeStringToFile(file, buff.toString(), false);
}
public static void writeStringToFile(File file, String buff) throws IOException
{
writeStringToFile(file, buff, false);
}
public static void writeBufferToFile(File file, StringBuffer buff, boolean append) throws IOException
{
writeStringToFile(file, buff.toString(), append);
}
public static void writeStringToFile(File file, String buff, boolean append) throws IOException
{
FileWriter writer = new FileWriter(file, append);
try
{
writer.write(buff);
}
finally
{
writer.close();
}
}
public static File createTempFile(File root)
{
return new File(root, "IUnit_TEMP" + System.currentTimeMillis());
}
public static void replace(File in, File out, final String match, final String replace) throws IOException
{
visitLines(in, out, new LineVisitor()
{
@Override
public String visit(String line, int lineNo)
{
return line.replaceAll(match, replace);
}
});
}
public static void visitLines(File in, LineVisitor lvisitor) throws IOException
{
BufferedReader breader = new BufferedReader(new FileReader(in));
try
{
int lineNo = 0;
String line = null;
while ((line = breader.readLine()) != null)
{
lvisitor.visit(line, lineNo++);
}
}
finally
{
breader.close();
}
}
public static void visitLines(File in, File out, LineVisitor lvisitor) throws IOException
{
BufferedReader breader = new BufferedReader(new FileReader(in));
try
{
BufferedWriter bwriter = new BufferedWriter(new FileWriter(out));
int lineNo = 0;
try
{
String line = null;
while ((line = breader.readLine()) != null)
{
bwriter.write(lvisitor.visit(line, lineNo++));
bwriter.write("\n");
}
}
finally
{
bwriter.close();
}
}
finally
{
breader.close();
}
}
public static boolean rmdir(File root)
{
purge(root, true);
return root.delete();
}
public static void purge(File root)
{
purge(root, false);
}
public static void purge(File root, boolean killDirs)
{
File[] f = root.listFiles();
if (f != null)
{
for (int i = 0; i < f.length; i++)
{
if (f[i].isDirectory())
{
purge(f[i], killDirs);
if (killDirs)
{
f[i].delete();
}
}
else
{
f[i].delete();
}
}
}
}
public static String readURLToString(URL url) throws IOException
{
ByteArrayOutputStream bout = new ByteArrayOutputStream();
try
{
InputStream urlIn = url.openStream();
try
{
copyStream(urlIn, bout);
}
finally
{
urlIn.close();
}
}
finally
{
bout.close();
}
return bout.toString();
}
public static void writeURLToFile(URL url, File destination) throws IOException
{
BufferedOutputStream bout = new BufferedOutputStream(new FileOutputStream(destination));
try
{
copyStream(url.openStream(), bout);
}
finally
{
bout.close();
}
}
public static String readLineSystemIn(String prompt) throws IOException
{
System.out.print(prompt);
System.out.flush();
String in = new BufferedReader(new InputStreamReader(System.in)).readLine();
in = in.trim();
return in;
}
public static String removeExtension(File dataFile)
{
return removeExtension(dataFile.getName());
}
public static String removeExtension(String name)
{
int ind = name.lastIndexOf(".");
if (ind != -1)
{
return name.substring(0, ind);
}
return name;
}
public static String getExtension(File dataFile)
{
return getExtension(dataFile.getName());
}
public static String getExtension(String name)
{
int ind = name.lastIndexOf(".");
if (ind != -1)
{
return name.substring(ind + 1);
}
return null;
}
public static boolean fileHasExtension(File file, String ext)
{
return fileHasExtension(file.getName(), ext);
}
public static boolean fileHasExtension(String file, String ext)
{
String ex = getExtension(file);
return ex != null && ex.equals(ext);
}
}
class Streamer
{
private final InputStream in;
private final TestContext context;
private final StringBuffer dataBuffer;
private final Semaphore semaphore = new Semaphore(1);
Streamer(InputStream i, TestContext tc, StringBuffer dBuffer)
{
in = i;
context = tc;
dataBuffer = dBuffer;
semaphore.acquireUninterruptibly();
}
void start()
{
new Thread(new Runnable()
{
public void run()
{
try
{
run1();
}
catch (IOException exc)
{
System.out.println(exc.toString());
}
}
public void run1() throws IOException
{
byte[] buff = new byte[1024 * 1024];
int read = 0;
while ((read = in.read(buff)) != -1)
{
if (context != null)
{
context.writeToProcessLog(buff, 0, read);
}
else
{
System.out.write(buff, 0, read);
System.out.flush();
}
if (dataBuffer != null)
{
dataBuffer.append(new String(buff, 0, read));
}
}
semaphore.release();
}
}).start();
}
public void waitForFlush()
{
semaphore.acquireUninterruptibly();
}
public static void writeFile(File file, StringBuffer buff)
{
writeFile(file, buff, false);
}
public static void writeFile(File file, StringBuffer buff, boolean append)
{
try
{
FileWriter writer = new FileWriter(file, append);
writer.write(buff.toString());
writer.close();
}
catch (Exception exc)
{
throw new RuntimeException("Could not write file", exc);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy