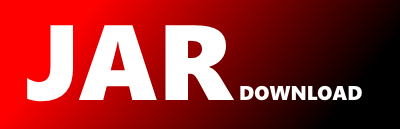
org.etlunit.util.StringUtils Maven / Gradle / Ivy
package org.etlunit.util;
import java.util.StringTokenizer;
public class StringUtils
{
public interface Justifier
{
String justify(String str, int length);
}
public static boolean isAsciiPrintable(char ch)
{
return ch >= 32 && ch < 127;
}
public static String encodeNonPrintable(String source)
{
StringBuilder strb = new StringBuilder();
for (char ch : source.toCharArray())
{
if (
isAsciiPrintable(ch)
)
{
strb.append((char) ch);
}
else
{
switch (ch)
{
case '\\':
strb.append("\\\\");
break;
case '\r':
strb.append("\\r");
break;
case '\n':
strb.append("\\n");
break;
case '\t':
strb.append("\\t");
break;
case '\0':
strb.append("\\0");
break;
case '\f':
strb.append("\\f");
break;
case '\b':
strb.append("\\b");
break;
default:
{
strb.append("0x");
strb.append(Integer.toHexString(ch));
}
}
}
}
return strb.toString();
}
public static String stripSpaces(String source)
{
StringBuilder strb = new StringBuilder();
for (int ch : source.toCharArray())
{
if (ch == ' ')
{
continue;
}
strb.append((char) ch);
}
return strb.toString();
}
private static class RightJustifier implements Justifier
{
@Override
public String justify(String str, int length)
{
if (str.length() < length)
{
StringBuilder sb = new StringBuilder();
int spacesRequired = length - str.length();
for (int i = 0; i < spacesRequired; i++)
{
sb.append(' ');
}
sb.append(str);
return sb.toString();
}
return str;
}
}
private static class LeftJustifier implements Justifier
{
@Override
public String justify(String str, int length)
{
if (str.length() < length)
{
StringBuilder sb = new StringBuilder(str);
int spacesRequired = length - str.length();
for (int i = 0; i < spacesRequired; i++)
{
sb.append(' ');
}
return sb.toString();
}
return str;
}
}
public static Justifier RIGHT_JUSTIFIED = new RightJustifier();
public static Justifier LEFT_JUSTIFIED = new LeftJustifier();
public static String wrapToLength(String text, int width, String lineToken, String lineDelimiter)
{
return wrapToLength(text, width, lineToken, lineDelimiter, LEFT_JUSTIFIED);
}
public static String wrapToLength(String text, int width, String lineToken, String lineDelimiter, Justifier justifier)
{
StringBuilder sb = new StringBuilder();
StringBuilder lineBuilder = new StringBuilder();
StringTokenizer st = new StringTokenizer(text, " ");
while (st.hasMoreTokens())
{
String line = st.nextToken();
if (lineBuilder.length() > 0 && (lineBuilder.length() + line.length() + 1) >= width)
{
if (lineToken != null)
{
sb.append(lineToken);
}
sb.append(justifier.justify(lineBuilder.toString(), width));
sb.append(lineDelimiter);
lineBuilder.setLength(0);
}
if (lineBuilder.length() != 0)
{
lineBuilder.append(' ');
}
lineBuilder.append(line);
}
if (lineBuilder.length() != 0)
{
if (lineToken != null)
{
sb.append(lineToken);
}
sb.append(justifier.justify(lineBuilder.toString(), width));
}
return sb.toString();
}
public static String sanitize(String input, char replacement)
{
return sanitize(input, "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789_", replacement);
}
public static String sanitize(String input, String allowedChars, char replacement)
{
StringBuffer sb = new StringBuffer(input.length());
for (int offset = 0; offset < input.length(); offset++)
{
char c = input.charAt(offset);
if (allowedChars.indexOf(c) == -1)
{
sb.append(replacement);
}
else
{
sb.append(c);
}
}
return sb.toString();
}
public static String prepareForLog(String text, String header)
{
return header + " " + text.replaceAll("\r|\n|\r\n", "$0" + header + " ");
}
public static String makeProperPropertyName(String property)
{
String base = makePropertyName(property);
base = Character.toUpperCase(base.charAt(0)) + base.substring(1);
return base;
}
public static String makePropertyName(String property)
{
StringBuffer buffer = new StringBuffer();
char[] array = property.toCharArray();
boolean capNext = false;
for (char ch : array)
{
if (ch == '-' || ch == '_' || ch == '.')
{
capNext = true;
}
else
{
if (capNext)
{
buffer.append(Character.toUpperCase(ch));
capNext = false;
}
else
{
buffer.append(ch);
}
}
}
return buffer.toString();
}
public static String convertToRelativePath(String absolutePath, String relativeTo)
{
StringBuilder relativePath = null;
// Thanks to:
// http://mrpmorris.blogspot.com/2007/05/convert-absolute-path-to-relative-path.html
absolutePath = absolutePath.replaceAll("\\\\", "/");
relativeTo = relativeTo.replaceAll("\\\\", "/");
if (!absolutePath.equals(relativeTo))
{
String[] absoluteDirectories = absolutePath.split("/");
String[] relativeDirectories = relativeTo.split("/");
//Get the shortest of the two paths
int length = absoluteDirectories.length < relativeDirectories.length ?
absoluteDirectories.length : relativeDirectories.length;
//Use to determine where in the loop we exited
int lastCommonRoot = -1;
int index;
//Find common root
for (index = 0; index < length; index++)
{
if (absoluteDirectories[index].equals(relativeDirectories[index]))
{
lastCommonRoot = index;
}
else
{
break;
//If we didn't find a common prefix then throw
}
}
if (lastCommonRoot != -1)
{
//Build up the relative path
relativePath = new StringBuilder();
//Add on the ..
for (index = lastCommonRoot + 1; index < absoluteDirectories.length; index++)
{
if (absoluteDirectories[index].length() > 0)
{
relativePath.append("../");
}
}
for (index = lastCommonRoot + 1; index < relativeDirectories.length - 1; index++)
{
relativePath.append(relativeDirectories[index]).append("/");
}
relativePath.append(relativeDirectories[relativeDirectories.length - 1]);
}
}
return relativePath == null ? null : relativePath.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy