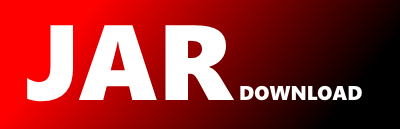
org.etlunit.util.TestHistory Maven / Gradle / Ivy
package org.etlunit.util;
import org.etlunit.parser.ETLTestMethod;
import java.io.*;
import java.util.*;
public class TestHistory
{
private int currentRunId;
private HistoryRun getRunList(boolean newRunId)
{
if (newRunId || runList.isEmpty())
{
runList.add(new HistoryRun());
}
// if list is getting too long remove the oldest (first) element
if (runList.size() > 25)
{
runList.remove(0);
}
return runList.get(runList.size() - 1);
}
public enum test_result
{
passed,
failed,
errored
}
public interface TestMethodHistory
{
test_result getTestResult();
String getTestClassName();
String getTestMethodName();
}
final class TestMethodHistoryImpl implements TestMethodHistory
{
private final String testClassName;
private final String testMethodName;
private final test_result testResult;
public TestMethodHistoryImpl(String testClassName, String testMethodName, test_result testResult)
{
this.testClassName = testClassName;
this.testMethodName = testMethodName;
this.testResult = testResult;
}
public test_result getTestResult()
{
return testResult;
}
public String getTestClassName()
{
return testClassName;
}
public String getTestMethodName()
{
return testMethodName;
}
}
private final class HistoryRun
{
final List passedList = new ArrayList();
final List failedList = new ArrayList();
final List erroredList = new ArrayList();
final List badList = new ArrayList();
final Map badMap = new HashMap();
}
private final List runList = new ArrayList();
private final File historyFile;
public TestHistory(File hist)
{
historyFile = hist;
}
public int getCurrentRunId()
{
return currentRunId;
}
public void load()
{
if (historyFile.exists())
{
try
{
BufferedReader br = new BufferedReader(new FileReader(historyFile));
try
{
String line = null;
while ((line = br.readLine()) != null)
{
StringTokenizer stk = new StringTokenizer(line, " ");
if (stk.countTokens() != 3)
{
// this line is corrupted. Skip.
throw new IllegalStateException();
}
String runId = stk.nextToken();
boolean newRunId = false;
try
{
int iRunId = Integer.parseInt(runId);
if (iRunId > currentRunId)
{
throw new IllegalStateException();
}
if (iRunId == currentRunId)
{
currentRunId = iRunId + 1;
newRunId = true;
}
}
catch (NumberFormatException exc)
{
// bad number - abort line.
throw new IllegalStateException();
}
HistoryRun run = getRunList(newRunId);
String method = stk.nextToken();
int index = method.lastIndexOf('.');
String tclass = method.substring(0, index);
method = method.substring(index + 1);
String status = stk.nextToken();
TestMethodHistoryImpl
impl =
new TestMethodHistoryImpl(tclass,
method,
(status.equals("pass")
? test_result.passed
: (status.equals("fail") ? test_result.failed : test_result.errored)));
switch (impl.getTestResult())
{
case passed:
run.passedList.add(impl);
break;
case failed:
run.failedList.add(impl);
run.badList.add(impl);
run.badMap.put(tclass, tclass);
break;
case errored:
run.erroredList.add(impl);
run.badList.add(impl);
run.badMap.put(tclass, tclass);
break;
}
}
}
finally
{
br.close();
}
}
catch (IOException exc)
{
throw new IllegalStateException(exc);
}
catch (IllegalStateException exc)
{
reset();
}
}
}
public void reset()
{
try
{
RandomAccessFile raf = new RandomAccessFile(historyFile, "rw");
raf.setLength(0);
raf.close();
}
catch (IOException exc)
{
throw new IllegalStateException(exc);
}
}
public void addMethod(ETLTestMethod method, test_result res) throws IOException
{
FileWriter fw = new FileWriter(historyFile, true);
try
{
fw.write(currentRunId + " " + method.getTestClass().getName() + "." + method.getName() + " " + (res
== test_result.passed ? "pass" : (res == test_result.failed ? "fail" : "error")) + "\n");
}
finally
{
fw.close();
}
}
public List getFailedTests(int runId)
{
if (runId >= currentRunId || runId < 0)
{
throw new IllegalArgumentException("Run does not exist: " + runId);
}
return runList.get(
runList.size() - (currentRunId - runId)
).failedList;
}
public List getFailedTests()
{
return getFailedTests(currentRunId - 1);
}
public List getErroredTests(int runId)
{
if (runId >= currentRunId || runId < 0)
{
throw new IllegalArgumentException("Run does not exist: " + runId);
}
return runList.get(
runList.size() - (currentRunId - runId)
).erroredList;
}
public List getErroredTests()
{
return getErroredTests(currentRunId - 1);
}
public List getPassedTests(int runId)
{
if (runId >= currentRunId || runId < 0)
{
throw new IllegalArgumentException("Run does not exist: " + runId);
}
return runList.get(
runList.size() - (currentRunId - runId)
).passedList;
}
public List getPassedTests()
{
return getPassedTests(currentRunId - 1);
}
public boolean isTestClassFailed(String name, int runId)
{
if (runId >= currentRunId || runId < 0)
{
throw new IllegalArgumentException("Run does not exist: " + runId);
}
return runList.get(
runList.size() - (currentRunId - runId)
).badMap.containsKey(name);
}
public boolean isTestClassFailed(String name)
{
return isTestClassFailed(name, currentRunId - 1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy