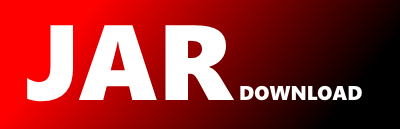
org.etlunit.Configuration Maven / Gradle / Ivy
package org.etlunit;
import org.etlunit.parser.ETLTestParser;
import org.etlunit.parser.ETLTestValueObject;
import org.etlunit.parser.ParseException;
import org.etlunit.util.IOUtils;
import org.etlunit.util.Incomplete;
import org.etlunit.util.NeedsTest;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.util.Enumeration;
@Incomplete
@NeedsTest
public class Configuration
{
private final ETLTestValueObject mapping;
public Configuration(String config)
{
this(init(config));
}
public Configuration(ETLTestValueObject pMapping)
{
if (pMapping.getValueType() != ETLTestValueObject.value_type.object)
{
throw new IllegalArgumentException("Configuration must be an object value type");
}
mapping = pMapping;
}
private static ETLTestValueObject init(String confObject)
{
try
{
String str2 = confObject.trim();
if (str2.length() > 0 && (str2.charAt(0) == '{') && (str2.charAt(str2.length() - 1) == '}'))
{
return ETLTestParser.loadObject(confObject);
}
else
{
return ETLTestParser.loadBareObject(confObject);
}
}
catch (ParseException e)
{
throw new IllegalArgumentException(e);
}
}
public ETLTestValueObject getRoot()
{
return mapping;
}
public ETLTestValueObject query(String path)
{
return mapping.query(path);
}
public static Configuration loadFromEnvironment(File configDir) throws IOException, ParseException
{
return loadFromEnvironment(configDir, null);
}
public static Configuration loadFromEnvironment(File configDir, String override) throws IOException, ParseException
{
return loadFromEnvironment(configDir, override, null);
}
public static Configuration loadFromEnvironment(File configDir, String override, ClassLoader clsLdr)
throws IOException, ParseException
{
return loadFromEnvironment(configDir, override, clsLdr, null);
}
public static Configuration loadFromEnvironment(File configDir, String override, ClassLoader clsLdr, ETLTestValueObject superOverride)
throws IOException, ParseException
{
if (clsLdr == null)
{
clsLdr = Configuration.class.getClassLoader();
}
ETLTestValueObject configObject = null;
// check the classpath for base configuration resources
Enumeration enu = clsLdr.getResources("config/etlunit.json");
while (enu.hasMoreElements())
{
URL url = enu.nextElement();
ETLTestValueObject remoteConfigObjectBase = ETLTestParser.loadObject(IOUtils.readURLToString(url));
if (configObject != null)
{
configObject = configObject.merge(remoteConfigObjectBase, ETLTestValueObject.merge_type.left_merge);
}
else
{
configObject = remoteConfigObjectBase;
}
}
// check the classpath for override configuration resources
if (override != null)
{
enu = clsLdr.getResources("config/" + override + ".json");
while (enu.hasMoreElements())
{
URL url = enu.nextElement();
ETLTestValueObject remoteConfigObjectBase = ETLTestParser.loadObject(IOUtils.readURLToString(url));
if (configObject != null)
{
configObject = configObject.merge(remoteConfigObjectBase, ETLTestValueObject.merge_type.right_merge);
}
else
{
configObject = remoteConfigObjectBase;
}
}
}
// look for a a config file in the local config directory
if (configDir != null)
{
File configBase = new File(configDir, "etlunit.json");
if (configBase.exists())
{
ETLTestValueObject baseObject = ETLTestParser.loadObject(IOUtils.readFileToString(configBase));
if (configObject != null)
{
configObject = configObject.merge(baseObject, ETLTestValueObject.merge_type.right_merge);
}
else
{
configObject = baseObject;
}
}
if (override != null)
{
File overrideBase = new File(configDir, override + ".json");
if (overrideBase.exists())
{
ETLTestValueObject overrideObject = ETLTestParser.loadObject(IOUtils.readFileToString(overrideBase));
if (configObject != null)
{
configObject = configObject.merge(overrideObject, ETLTestValueObject.merge_type.right_merge);
}
else
{
configObject = overrideObject;
}
}
}
}
if (configObject == null)
{
// can't survive this
throw new IllegalArgumentException("No configuration found");
}
if (superOverride != null)
{
configObject = configObject.merge(superOverride, ETLTestValueObject.merge_type.right_merge);
}
return new Configuration(configObject);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy