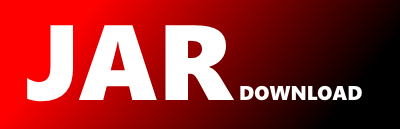
org.etlunit.ProcessDescription Maven / Gradle / Ivy
package org.etlunit;
import java.io.File;
import java.util.*;
/*Replacement for Process Builder. This one is to allow for better
* testing.
*/
public final class ProcessDescription
{
private final String command;
private final List arguments = new ArrayList();
private File workingDirectory;
private File outputBuffer;
// This hashmap was changed to a treemap to make the output
// stable. This was done for debugging and testing, but shouldn't
// have an impact on performance.
private final Map environment = new TreeMap(System.getenv());
public ProcessDescription(String command)
{
this.command = command;
}
public ProcessDescription argument(String arg)
{
arguments.add(arg);
return this;
}
public ProcessDescription output(File arg)
{
outputBuffer = arg;
return this;
}
public File getOutputFile()
{
return outputBuffer;
}
public ProcessDescription arguments(List args)
{
arguments.addAll(args);
return this;
}
public String getCommandName()
{
File f = new File(command);
return f.getName();
}
public ProcessDescription workingDirectory(File file)
{
this.workingDirectory = file;
return this;
}
public ProcessDescription environment(String var, String value)
{
environment.put(var, value);
return this;
}
public String getCommand()
{
return command;
}
public List getArguments()
{
return arguments;
}
public boolean hasArguments()
{
return arguments.size() != 0;
}
public File getWorkingDirectory()
{
return workingDirectory;
}
public Map getEnvironment()
{
return environment;
}
public String debugString()
{
return
new StringBuilder("Command[")
.append(command)
.append("]Arguments[")
.append(arguments.toString())
.append("]Env[")
.append(deltaEnvironment())
.append("]Cwd[")
.append(workingDirectory)
.append("]")
.append("]Output[")
.append(outputBuffer)
.append("]")
.toString();
}
public Map deltaEnvironment()
{
Map deltaMap = new TreeMap(getEnvironment());
Map sysMap = System.getenv();
Set> set = sysMap.entrySet();
Iterator> it = set.iterator();
while (it.hasNext())
{
Map.Entry entry = it.next();
String key = entry.getKey();
String value = entry.getValue();
String envValue = deltaMap.get(key);
if (envValue != null && (envValue == value || envValue.equals(value)))
{
deltaMap.remove(key);
}
}
return deltaMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy