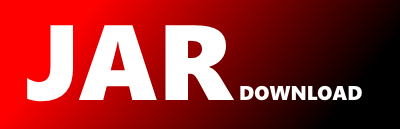
org.etlunit.feature.AnnotationValidationFeature Maven / Gradle / Ivy
package org.etlunit.feature;
import com.google.inject.name.Named;
import org.etlunit.ClassDirector;
import org.etlunit.Log;
import org.etlunit.NullClassDirector;
import org.etlunit.TestConstants;
import org.etlunit.json.validator.JsonSchema;
import org.etlunit.json.validator.JsonSchemaValidationException;
import org.etlunit.json.validator.JsonValidator;
import org.etlunit.parser.ETLTestAnnotation;
import org.etlunit.parser.ETLTestClass;
import org.etlunit.parser.ETLTestMethod;
import javax.inject.Inject;
import java.util.List;
import java.util.Map;
public class AnnotationValidationFeature extends AbstractFeature
{
private Log applicationLog;
private Log userLog;
private List featureList;
@Override
public long getPriorityLevel()
{
return Long.MIN_VALUE;
}
@Inject
public void setFeatureList(List features)
{
featureList = features;
}
@Inject
public void setApplicationLog(@Named("applicationLog") Log log)
{
this.applicationLog = log;
}
@Inject
public void setUserLog(@Named("userLog") Log log)
{
this.userLog = log;
}
@Override
public String getFeatureName()
{
return "annotation-validator";
}
// this director will decline any tests or classes which have invalid annotations
@Override
public ClassDirector getDirector()
{
return new NullClassDirector()
{
@Override
public response_code accept(ETLTestClass cl)
{
for (ETLTestAnnotation annotation : cl.getAnnotations())
{
validateAnnotation(cl.getQualifiedName(), annotation);
}
return response_code.defer;
}
@Override
public response_code accept(ETLTestMethod mt)
{
for (ETLTestAnnotation annotation : mt.getAnnotations())
{
validateAnnotation(mt.getQualifiedName(), annotation);
}
return response_code.defer;
}
};
}
private void validateAnnotation(String qualifiedName, ETLTestAnnotation annotation)
{
for (Feature feature : featureList)
{
FeatureMetaInfo fmi = feature.getMetaInfo();
if (fmi != null)
{
Map exports = fmi.getExportedAnnotations();
if (exports != null)
{
FeatureAnnotation fmiannot = exports.get(annotation.getName());
if (fmiannot != null)
{
if (annotation.hasValue())
{
if (fmiannot.getPropertyType() == FeatureAnnotation.propertyType.none)
{
throw new IllegalArgumentException(TestConstants.ERR_INVALID_ANNOTATION_PROPERTIES
+ " not allowed: "
+ annotation.toString());
}
List validator = fmiannot.getValidator();
if (validator != null)
{
for (JsonSchema jschema : validator)
{
JsonValidator jval = new JsonValidator(jschema);
try
{
jval.validate(annotation.getValue().getJsonNode());
// the one and only good case
return;
}
catch (JsonSchemaValidationException e)
{
}
}
}
}
else
{
if (fmiannot.getPropertyType() == FeatureAnnotation.propertyType.required)
{
throw new IllegalArgumentException(TestConstants.ERR_INVALID_ANNOTATION_PROPERTIES
+ ": "
+ annotation.toString());
}
else
{
return;
}
}
}
}
}
}
userLog.severe("Annotation " + annotation.getName() + " is invalid on object " + qualifiedName);
applicationLog.severe("Annotation " + annotation.getName() + " is invalid on object " + qualifiedName);
throw new IllegalArgumentException(TestConstants.ERR_INVALID_ANNOTATION + ": " + annotation.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy