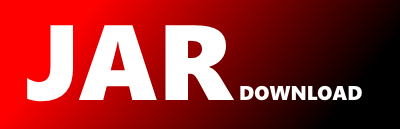
org.etlunit.io.JavaForker Maven / Gradle / Ivy
package org.etlunit.io;
import org.etlunit.ProcessDescription;
import org.etlunit.ProcessFacade;
import org.etlunit.RuntimeSupport;
import java.io.File;
import java.io.IOException;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.List;
public class JavaForker
{
private Class mainClass;
private int startingHeapSizeInMegabytes = -1;
private int maximumHeapSizeInMegabytes = -1;
private File workingDirectory = new File(".");
private List classpathEntries = new ArrayList();
private List mainClassArguments = new ArrayList();
private String javaRuntime = "java";
private File output;
private final RuntimeSupport runtimeSupport;
public JavaForker(RuntimeSupport runtimeSupport)
{
this.runtimeSupport = runtimeSupport;
}
public void setOutput(File output)
{
this.output = output;
}
public Class getMainClass()
{
return mainClass;
}
public void setMainClass(Class mainClass)
{
this.mainClass = mainClass;
}
public int getStartingHeapSizeInMegabytes()
{
return startingHeapSizeInMegabytes;
}
public void setStartingHeapSizeInMegabytes(int startingHeapSizeInMegabytes)
{
this.startingHeapSizeInMegabytes = startingHeapSizeInMegabytes;
}
public int getMaximumHeapSizeInMegabytes()
{
return maximumHeapSizeInMegabytes;
}
public void setMaximumHeapSizeInMegabytes(int maximumHeapSizeInMegabytes)
{
this.maximumHeapSizeInMegabytes = maximumHeapSizeInMegabytes;
}
private String getClasspath() throws IOException
{
List cpEntries = new ArrayList(classpathEntries);
if (cpEntries.size() == 0)
{
// use the system class path
String systemCp = System.getProperty("java.class.path");
String[] pathArray = systemCp.split(File.pathSeparator);
for (String pathEntry : pathArray)
{
cpEntries.add(new File(pathEntry));
}
}
StringBuilder builder = new StringBuilder();
int count = 0;
final int totalSize = cpEntries.size();
for (File classpathEntry : cpEntries)
{
builder.append(classpathEntry.getCanonicalPath());
count++;
if (count < totalSize)
{
builder.append(File.pathSeparator);
}
}
return builder.toString();
}
public void setWorkingDirectory(File workingDirectory)
{
this.workingDirectory = workingDirectory;
}
public void addClasspathEntry(File classpathEntry)
{
this.classpathEntries.add(classpathEntry);
}
public void addArgument(String argument)
{
this.mainClassArguments.add(argument);
}
public void setJavaRuntime(String javaRuntime)
{
this.javaRuntime = javaRuntime;
}
public ProcessFacade startProcess() throws IOException
{
ProcessDescription pd = new ProcessDescription(this.javaRuntime);
if (startingHeapSizeInMegabytes != -1)
{
pd.argument(MessageFormat.format("-Xms{0}M", String.valueOf(startingHeapSizeInMegabytes)));
}
if (maximumHeapSizeInMegabytes != -1)
{
pd.argument(MessageFormat.format("-Xmx{0}M", String.valueOf(maximumHeapSizeInMegabytes)));
}
pd
.output(output)
.argument("-classpath")
.argument(getClasspath())
.argument(this.mainClass.getName())
.workingDirectory(this.workingDirectory.getCanonicalFile())
.output(output);
for (String arg : mainClassArguments)
{
pd.argument(arg);
}
return runtimeSupport.execute(pd);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy