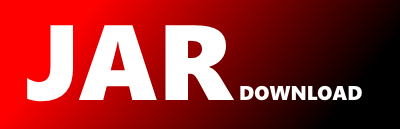
org.etlunit.util.CommandLine Maven / Gradle / Ivy
package org.etlunit.util;
import java.util.*;
public class CommandLine
{
private enum switch_type
{
full,
terse
}
public static final class Switch
{
private final String name;
private final String shortName;
private final String description;
public Switch(String n, String d)
{
name = n;
description = d;
int offset = 0;
StringBuffer buffer = new StringBuffer();
buffer.append(Character.toLowerCase(name.charAt(0)));
while ((offset = name.indexOf('-', offset)) != -1)
{
offset++;
if (offset == name.length())
{
break;
}
char next = name.charAt(offset);
if (next == '-')
{
continue;
}
buffer.append(Character.toLowerCase(next));
}
shortName = buffer.toString();
}
public String getName()
{
return name;
}
public String getCommandLineForm()
{
return "--" + getName();
}
public String getAbbreviatedCommandLineForm()
{
return "-" + getShortName();
}
public String getShortName()
{
return shortName;
}
public String getDescription()
{
return description;
}
}
private final CommandLine deferred;
private final List args = new ArrayList();
private final Map switches = new HashMap();
private final List plainSwitches = new ArrayList();
public CommandLine()
{
this((CommandLine) null);
}
public CommandLine(String[] args)
{
this(args, null);
}
public CommandLine(String[] args, CommandLine def)
{
this(def);
setArguments(args);
}
public CommandLine(String args)
{
this(args, null);
}
public CommandLine(String args, CommandLine def)
{
this(def);
setArguments(args);
}
public CommandLine(CommandLine df)
{
deferred = df;
}
public void setArguments(String pArgs)
{
setArguments(listArgs(pArgs));
}
public void setArguments(String[] pArgs)
{
for (int i = 0; i < pArgs.length; i++)
{
if (pArgs[i].startsWith("-"))
{
String text = pArgs[i];
// strip the first '-'
text = text.substring(1);
// optionally strip the second '-'
if (text.charAt(0) == '-')
{
text = text.substring(1);
}
int indexOfEq = text.indexOf("=");
if (indexOfEq == -1)
{
String sw = text.toLowerCase();
plainSwitches.add(sw);
switches.put(sw, sw);
}
else
{
String sw = text.substring(0, indexOfEq).toLowerCase();
String value = text.substring(indexOfEq + 1);
switches.put(sw, value);
}
}
else
{
args.add(pArgs[i]);
}
}
}
public int getArgumentCount()
{
return args.size();
}
public String getArgument(int offset)
{
return args.get(offset);
}
public List getArguments()
{
return Collections.unmodifiableList(args);
}
public List getSwitches()
{
return Collections.unmodifiableList(new ArrayList(switches.values()));
}
private boolean hasSwitch0(String name)
{
return
switches.containsKey(name);
}
private boolean hasSwitch0(Switch name)
{
return
hasSwitch0(name.getName())
|| hasSwitch0(name.getShortName());
}
public boolean hasSwitch(Switch name)
{
return
hasSwitch0(name) || (deferred != null && deferred.hasSwitch(name));
}
public switch_type getSwitchType(Switch name)
{
if (switches.containsKey(name.getName()))
{
return switch_type.full;
}
else if (switches.containsKey(name.getShortName()))
{
return switch_type.terse;
}
if (deferred != null)
{
return deferred.getSwitchType(name);
}
throw new IllegalArgumentException("Switch not present: " + name);
}
public boolean hasSwitch(String name)
{
return switches.containsKey(name) || (deferred != null && deferred.hasSwitch(name));
}
private boolean hasSwitchOption0(String name)
{
boolean ihs = hasSwitch0(name);
boolean ihp = plainSwitches.contains(name);
if (ihs && !ihp)
{
return true;
}
return false;
}
public boolean hasSwitchOption(String name)
{
return hasSwitchOption0(name) || (deferred != null && deferred.hasSwitchOption0(name));
}
public boolean hasSwitchOption(Switch name)
{
return hasSwitchOption(name.getShortName()) || hasSwitchOption(name.getName());
}
private String getSwitch0(String name)
{
String n = switches.get(name);
return n;
}
public String getSwitch(String name)
{
String s = getSwitch0(name);
if (s != null)
{
return s;
}
if (deferred != null)
{
return deferred.getSwitch0(name);
}
return null;
}
public String getSwitch(Switch name)
{
String s = getSwitch(name.getShortName());
if (s != null)
{
return s;
}
return getSwitch(name.getName());
}
public boolean getBooleanSwitch(String name)
{
return getBooleanSwitch(name, false, true);
}
public boolean getBooleanSwitch(Switch name)
{
return getBooleanSwitch(name, false, true);
}
public boolean getBooleanSwitch(String name, boolean optionDefault, boolean flagDefault)
{
String s = getSwitch(name);
return s == null ? optionDefault : (s.equals(name)) ? flagDefault : (s.equalsIgnoreCase("true"));
}
public boolean getBooleanSwitch(Switch name, boolean optionDefault, boolean flagDefault)
{
if (hasSwitchOption0(name.getName()))
{
return getSwitch0(name.getName()).equalsIgnoreCase("true");
}
else if (hasSwitchOption0(name.getShortName()))
{
return getSwitch0(name.getShortName()).equalsIgnoreCase("true");
}
else if (deferred != null && deferred.hasSwitchOption0(name.getName()))
{
return deferred.getSwitch0(name.getName()).equalsIgnoreCase("true");
}
else if (deferred != null && deferred.hasSwitchOption0(name.getShortName()))
{
return deferred.getSwitch0(name.getShortName()).equalsIgnoreCase("true");
}
return hasSwitch(name) ? flagDefault : optionDefault;
}
public String toString()
{
return "Switches: " + switches + ", args: " + args;
}
private static String[] listArgs(String pArgs)
{
List list = new ArrayList();
StringTokenizer st = new StringTokenizer(pArgs);
while (st.hasMoreTokens())
{
list.add(st.nextToken());
}
return list.toArray(new String[list.size()]);
}
public String verifyAllSwitches(Switch[] swa)
{
Iterator it = switches.keySet().iterator();
while (it.hasNext())
{
String switchName = it.next();
Switch sw = null;
// look up in the list of allowed
for (int i = 0; i < swa.length; i++)
{
if (switchName.equals(swa[i].getName()) || switchName.equals(swa[i].getShortName()))
{
sw = swa[i];
break;
}
}
if (sw == null)
{
return switchName;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy