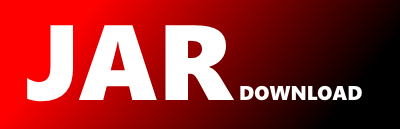
org.etlunit.util.VelocityUtil Maven / Gradle / Ivy
package org.etlunit.util;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.app.VelocityEngine;
import org.etlunit.context.VariableContext;
import java.io.File;
import java.io.StringWriter;
import java.util.Map;
public class VelocityUtil
{
static VelocityEngine getVelocityEngine()
{
return getVelocityEngine(new File("."));
}
static VelocityEngine getVelocityEngine(File templateRoot)
{
VelocityEngine velocityEngine = new VelocityEngine();
velocityEngine.addProperty("file.resource.loader.path", templateRoot.getAbsolutePath());
velocityEngine.init();
return velocityEngine;
}
public static void writeTestReport(File testReport, TestSuite suite) throws Exception
{
VelocityContext vcontext = new VelocityContext();
vcontext.put("testSuite", suite);
writeTemplate(new File("..\\conf\\TEST-report.vm"), testReport, suite);
}
public static void writeTemplate(File template, File output, Object bean) throws Exception
{
VelocityContext vcontext = new VelocityContext();
vcontext.put("bean", bean);
IOUtils.writeBufferToFile(output, new StringBuffer(writeTemplate(template, bean)));
}
public static String writeTemplate(File template, Object bean) throws Exception
{
VelocityContext vcontext = new VelocityContext();
vcontext.put("bean", bean);
/* lets render a template */
String st = IOUtils.readFileToString(template);
return writeTemplate(st, bean);
}
public static String writeTemplate(String template, Object bean) throws Exception
{
VelocityContext vcontext = new VelocityContext();
vcontext.put("bean", bean);
StringWriter w = new StringWriter();
getVelocityEngine().evaluate(vcontext, w, "log", template);
return w.toString();
}
public static String writeTemplate(String template, Map bean) throws Exception
{
return writeTemplate(template, bean, new File("."));
}
public static String writeTemplate(String template, Map bean, File templateRoot) throws Exception
{
VelocityContext context = new VelocityContext(bean);
StringWriter w = new StringWriter();
getVelocityEngine(templateRoot).evaluate(context, w, "log", template);
return w.toString();
}
public static String writeTemplate(String template, VariableContext bean) throws Exception
{
return writeTemplate(template, bean, new File("."));
}
public static String writeTemplate(String template, VariableContext bean, File templateRoot) throws Exception
{
if (!bean.hasVariableBeenDeclared("D"))
{
bean.declareVariable("D");
bean.setStringValue("D", "$");
}
VelocityContext context = new VelocityContext(bean.getVelocityWrapper());
StringWriter w = new StringWriter();
getVelocityEngine(templateRoot).evaluate(context, w, "log", template);
return w.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy