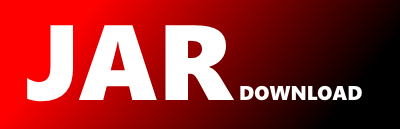
utsupport.test_report Maven / Gradle / Ivy
package utsupport;
import org.apache.velocity.VelocityContext;
import org.apache.velocity.app.Velocity;
import java.io.File;
import java.io.FileFilter;
import java.io.StringWriter;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class test_report
{
public static void main(String[] argv) throws Exception
{
Velocity.init();
/* lets make a Context and put data into it */
VelocityContext context = new VelocityContext();
TestSuite ts = new TestSuite(argv[1]);
context.put("testSuite", ts);
/* lets render a template */
StringWriter w = new StringWriter();
String st = create_test_cases.readFile(new File("..\\conf\\TEST-report.vm")).toString();
File out = new File(argv[2]);
if (argv[0].equals("init"))
{
StringBuffer sb = new StringBuffer();
// write the start time
sb.append(System.currentTimeMillis());
create_test_cases.writeFile(new File(out.getParentFile(), argv[1] + ".time"), sb);
ts.addTestCase(argv[1], argv[1], 0L);
}
else if (argv[0].equals("complete"))
{
File log = new File("\\\\etldev01\\F$\\unit_test\\log");
System.out.println("Log files path: " + log.getAbsolutePath());
File[] logs = log
.listFiles(new FileFilterImpl());
String sessionLog = "No Session Log: " + logs;
if (logs != null && logs.length > 0)
{
sessionLog = create_test_cases.readFile(logs[0]).toString();
}
ts.getStdout().append(sessionLog);
StringBuffer sb = create_test_cases.readFile(new File(out.getParentFile(), argv[1] + ".time"));
System.out.println(sb);
long start = Long.parseLong(sb.toString().trim());
long end = System.currentTimeMillis();
String s = create_test_cases.readFile(new File(argv[3])).toString();
// check for success messages
Pattern p = Pattern.compile("INFO: Workflow \\[[^\\]]+\\]: Execution succeeded.");
Matcher m = p.matcher(s);
if (!m.find())
{
ts.addTestCase(argv[1], argv[1], end - start, "Informatica error", s);
}
else
{
p = Pattern.compile("TST Status: Passed");
m = p.matcher(s);
if (m.find())
{
ts.addTestCase(argv[1], argv[1], end - start);
}
else
{
p = Pattern.compile("-----------\\r\\n 0\\r\\n\\r\\n\\(1 rows affected\\)");
m = p.matcher(s);
if (m.find())
{
ts.addTestCase(argv[1], argv[1], end - start);
}
else
{
p = Pattern.compile("Files \\[[^ ]+\\] and \\[[^ ]]+\\] are identical");
m = p.matcher(s);
if (!m.find())
{
ts.addTestCase(argv[1], argv[1], end - start, "File Assertion error", s);
}
else
{
ts.addTestCase(argv[1], argv[1], end - start);
}
}
}
}
ts.getTestCases().get(0).getStdout().append(sessionLog);
w = new StringWriter();
Velocity.evaluate(context, w, "mystring", st);
create_test_cases.writeFile(out, new StringBuffer(w.toString()));
}
}
public static class FileFilterImpl implements FileFilter
{
public static final Pattern p = Pattern.compile("session\\.log(\\.201\\d{11})?");
public boolean accept(File pathname)
{
boolean b = p.matcher(pathname.getName()).matches();
System.out.println("Log file: " + pathname.getAbsolutePath() + " matches[" + b + "]");
return b;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy