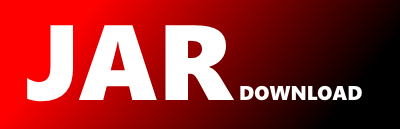
org.etlunit.feature.execute.RuntimeExecutor Maven / Gradle / Ivy
package org.etlunit.feature.execute;
import javax.inject.Inject;
import javax.inject.Named;
import org.etlunit.*;
import org.etlunit.context.VariableContext;
import org.etlunit.feature.Feature;
import org.etlunit.feature.extend.Extender;
import org.etlunit.parser.ETLTestOperation;
import org.etlunit.parser.ETLTestValueObject;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class RuntimeExecutor implements Extender {
private RuntimeSupport runtimeSupport;
private Log applicationLog;
private final Feature executeFeature;
public RuntimeExecutor(Feature executeFeature) {
this.executeFeature = executeFeature;
}
@Inject
public void setLogger(@Named("applicationLog") Log log)
{
applicationLog = log;
}
@Inject
public void addRuntimeSupport(RuntimeSupport support)
{
runtimeSupport = support;
}
public boolean canHandleRequest(ETLTestOperation etlTestOperation, ETLTestValueObject etlTestValueObject, VariableContext context, ExecutionContext executionContext) {
return
etlTestValueObject != null
&&
etlTestValueObject.query("executable") != null;
}
public ClassResponder.action_code process(ETLTestOperation etlTestOperation, ETLTestValueObject etlTestValueObject, VariableContext context, ExecutionContext executionContext) {
// executable is required,
// arguments, redirect stderr and working directory are optional
String exe = etlTestValueObject.query("executable").getValueAsString();
List args = new ArrayList();
ETLTestValueObject argvalue = etlTestValueObject.query("argument-list");
if (argvalue != null)
{
args.addAll(argvalue.getValueAsListOfStrings());
}
File working = null;
argvalue = etlTestValueObject.query("working-directory");
if (argvalue != null)
{
working = new File(etlTestValueObject.getValueAsString());
}
Boolean redirect = null;
argvalue = etlTestValueObject.query("redirect-error-stream");
if (argvalue != null)
{
redirect = etlTestValueObject.getValueAsBoolean() ? Boolean.TRUE : Boolean.FALSE;
}
ProcessDescription pd = new ProcessDescription(exe).arguments(args);
if (working != null)
{
pd.workingDirectory(working);
}
ProcessFacade facade = null;
try {
facade = runtimeSupport.execute(pd);
facade.waitForCompletion();
applicationLog.info("Process completed with code [" + facade.getCompletionCode() + "]");
applicationLog.info("Process output [" + facade.getInputBuffered() + "]");
} catch (IOException e) {
applicationLog.severe(e.toString(), e);
}
return ClassResponder.action_code.handled;
}
public Feature getFeature() {
return executeFeature;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy