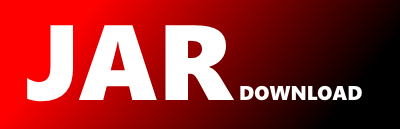
org.etlunit.maven.util.JavaProperty Maven / Gradle / Ivy
package org.etlunit.maven.util;
import org.etlunit.json.validator.JsonSchemaObjectNode;
import java.util.List;
public class JavaProperty
{
private final String name;
private final JsonSchemaObjectNode.valid_type dataType;
private final List enumValues;
private final String dataTypeName;
private final boolean required;
public JavaProperty(String name, JsonSchemaObjectNode.valid_type type, List enumValues, boolean required)
{
this.name = name;
this.required = required;
dataType = type;
this.enumValues = enumValues;
if (enumValues == null)
{
switch (type)
{
case t_string:
dataTypeName = "String";
break;
case t_number:
dataTypeName = "String";
break;
case t_integer:
dataTypeName = "int";
break;
case t_boolean:
dataTypeName = "boolean";
break;
case t_object:
dataTypeName = "Map";
break;
case t_array:
dataTypeName = "List";
break;
case t_any:
dataTypeName = "Object";
break;
case t_null:
throw new IllegalArgumentException("Data type null not supported");
default:
throw new IllegalArgumentException("Data type not supported");
}
}
else
{
this.dataTypeName = null;
}
}
public void append(StringBuffer buffer)
{
String typeName = dataTypeName;
if (typeName == null)
{
// this is an enum
typeName = getPropertyName() + "_enum";
buffer.append("\tpublic enum ").append(typeName).append("\n\t{\n");
for (int i = 0; i < enumValues.size(); i++)
{
String e = enumValues.get(i);
buffer.append("\t\t").append(makePropertyName(e));
if (i != (enumValues.size() - 1))
{
buffer.append(",");
}
buffer.append("\n");
}
buffer.append("\t}\n");
}
buffer.append("\tpublic static final String ").append(getProperPropertyName().toUpperCase()).append("_JSON_NAME = \"").append(name).append("\";\n");
buffer.append("\tprivate ").append(typeName).append(" ").append(getPropertyName()).append(";\n");
if (!required)
{
/*
buffer.append("\tpublic boolean has" + getProperPropertyName()).append("()\n");
buffer.append("\t{\n");
buffer.append("\t\treturn ").append(getPropertyName()).append(" != null;\n");
buffer.append("\t}\n");
*/
}
buffer.append("\tpublic " + typeName + " get" + getProperPropertyName()).append("()\n");
buffer.append("\t{\n");
/*
if (required)
{
buffer.append("\t\tif (!has").append(getProperPropertyName()).append(") {throw new IllegalStateException(\"Property '" + getPropertyName() + "' never assigned\");}\n\n");
}
*/
buffer.append("\t\treturn " + getPropertyName() + ";\n");
buffer.append("\t}\n");
}
public String getProperPropertyName()
{
return makeProperPropertyName(name);
}
public static String makeProperPropertyName(String property)
{
String base = makePropertyName(property);
base = Character.toUpperCase(base.charAt(0)) + base.substring(1);
return base;
}
public String getPropertyName()
{
return makePropertyName(name);
}
public static String makePropertyName(String property)
{
StringBuffer buffer = new StringBuffer();
char[] array = property.toCharArray();
boolean capNext = false;
for (char ch : array)
{
if (ch == '-' || ch == '_' || ch == '.')
{
capNext = true;
}
else
{
if (capNext)
{
buffer.append(Character.toUpperCase(ch));
capNext = false;
}
else
{
buffer.append(ch);
}
}
}
return buffer.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy