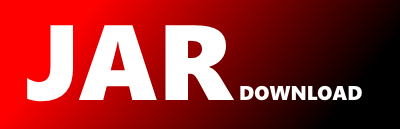
org.etlunit.feature.informatica.InformaticaDomain Maven / Gradle / Ivy
package org.etlunit.feature.informatica;
import org.etlunit.RuntimeSupport;
import org.etlunit.io.FileBuilder;
import org.etlunit.parser.ETLTestValueObject;
import org.etlunit.util.IOUtils;
import org.etlunit.util.Incomplete;
import org.etlunit.util.VelocityUtil;
import java.io.File;
import java.util.*;
/*
working-root: 'path',
client-version: '9.1.0hf3',
connectivity-host: 'etldev01',
connectivity-port: 6005,
username: 'bsmith',
password-encrypted: '',
repositories: {
dev_pc_repo: {},
}
integration-services: [
'dev_ci_is',
'dev_is',
'dev_java_is'
]
*/
public class InformaticaDomain {
private final String domainName;
private final String clientVersion;
private final String connectivityHost;
private final int connectivityPort;
private final String userName;
private final String passwordEncrypted;
private final File domainFile;
private final File workingRoot;
private final File localizedWorkingRoot;
private final InformaticaRepository defaultRepository;
private final List integrationServices = new ArrayList();
private final InformaticaIntegrationService defaultIntegrationService;
private final List repositories = new ArrayList();
@Incomplete(explanation = "Implement domains.infa as a user-supplied file")
public InformaticaDomain(InformaticaFeatureModule module, String name, ETLTestValueObject value, RuntimeSupport runtimeSupport, File domainsFile)
{
domainName = name;
clientVersion = getRequiredAttribute("client-version", value);
connectivityHost = getOptionalAttribute("connectivity-host", value);
String root = getRequiredAttribute("working-root", value);
workingRoot = new File(root);
String projectName = runtimeSupport.getProjectName();
String projectVersion = runtimeSupport.getProjectVersion();
String projectUser = runtimeSupport.getProjectUser();
String projectUid = runtimeSupport.getProjectUID();
localizedWorkingRoot = new FileBuilder(workingRoot).subdir(projectUser).subdir(projectName).subdir(projectVersion).subdir(projectUid).file();
String port = getOptionalAttribute("connectivity-port", value);
if (port != null)
{
connectivityPort = Integer.parseInt(port);
}
else
{
connectivityPort = -1;
}
userName = getRequiredAttribute("username", value);
passwordEncrypted = getRequiredAttribute("password-encrypted", value);
ETLTestValueObject erepositories = value.query("repositories");
if (erepositories == null)
{
throw new IllegalArgumentException("Informatica domain missing repositories");
}
for (String repo : erepositories.getValueAsListOfStrings())
{
InformaticaRepository repository = new InformaticaRepository(this, repo, module);
if (getRepository(repo) != null)
{
throw new IllegalArgumentException("Duplicate repository declaration: " + repo);
}
repositories.add(repository);
}
if (repositories.size() == 0)
{
throw new IllegalArgumentException("Informatica domain repositories element is empty");
}
String defRepo = getOptionalAttribute("default-repository", value);
InformaticaRepository repo = repositories.get(0);
if (defRepo != null)
{
repo = getRepository(defRepo);
if (repo == null)
{
throw new IllegalArgumentException("Default repository does not exist in domain: " + defRepo);
}
}
defaultRepository = repo;
ETLTestValueObject list = value.query("integration-services");
if (list == null)
{
throw new IllegalArgumentException("Missing integration-services element in repository configuration " + name);
}
List list1 = list.getValueAsListOfStrings();
Iterator isit = list1.iterator();
while (isit.hasNext())
{
String next = isit.next();
if (getIntegrationService(next) != null)
{
throw new IllegalArgumentException("Integration service " + next + " specified more than once");
}
integrationServices.add(new InformaticaIntegrationService(this, next, module));
}
if (integrationServices.size() == 0)
{
throw new IllegalArgumentException("Missing integration-services element in repository configuration " + name + " is empty");
}
InformaticaIntegrationService primalIs = integrationServices.get(0);
String defIs = getOptionalAttribute("default-integration-service", value);
InformaticaIntegrationService dis = primalIs;
if (defIs != null)
{
dis = getIntegrationService(defIs);
if (dis == null)
{
throw new IllegalArgumentException("Default integration service not found in domain: " + defIs);
}
}
defaultIntegrationService = dis == null ? primalIs : dis;
if (getConnectivityHost() != null) {
String template = null;
try
{
template = IOUtils.readURLToString(getClass().getResource("/domains.infa.vm"));
Map bean = new HashMap();
bean.put("domainName", getDomainName());
bean.put("connectivityHost", getConnectivityHost());
bean.put("connectivityPort", String.valueOf(getConnectivityPort()));
String vel = VelocityUtil.writeTemplate(template, bean);
domainFile = runtimeSupport.createTempFile("domains.infa." + getDomainName());
IOUtils.writeBufferToFile(domainFile, new StringBuffer(vel));
}
catch (Exception e)
{
throw new IllegalStateException(e);
}
}
else
{
domainFile = domainsFile;
}
}
private String getOptionalAttribute(String s, ETLTestValueObject value) {
return getAttribute(s, value, false);
}
private String getRequiredAttribute(String s, ETLTestValueObject value) {
return getAttribute(s, value, true);
}
private String getAttribute(String s, ETLTestValueObject value, boolean required) {
ETLTestValueObject res = value.query(s);
if (required && res == null)
{
throw new IllegalArgumentException("Informatica domain missing attribute: " + s);
}
return res == null ? null : res.getValueAsString();
}
public String getDomainName() {
return domainName;
}
public String getClientVersion() {
return clientVersion;
}
public String getConnectivityHost() {
return connectivityHost;
}
public int getConnectivityPort() {
return connectivityPort;
}
public String getUserName() {
return userName;
}
public String getPasswordEncrypted() {
return passwordEncrypted;
}
public InformaticaRepository getRepository(String name)
{
for (InformaticaRepository repo : repositories)
{
if (repo.getRepositoryName().equals(name))
{
return repo;
}
}
return null;
}
public List getRepositories() {
return repositories;
}
public InformaticaRepository getDefaultRepository() {
return defaultRepository;
}
public File getWorkingRoot() {
return workingRoot;
}
public File getLocalizedWorkingRoot()
{
return localizedWorkingRoot;
}
public List getIntegrationServices()
{
return integrationServices;
}
public InformaticaIntegrationService getDefaultIntegrationService()
{
return defaultIntegrationService;
}
public InformaticaIntegrationService getIntegrationService(String isName)
{
for (InformaticaIntegrationService is : integrationServices)
{
if (is.getIntegrationServiceName().equals(isName))
{
return is;
}
}
return null;
}
public boolean containsIntegrationService(String isName)
{
return getIntegrationService(isName) != null;
}
public File getDomainFile()
{
if (domainFile == null)
{
throw new UnsupportedOperationException("Domain file not supported yet");
}
return domainFile;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy