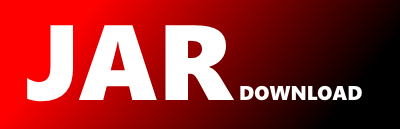
org.etlunit.feature.informatica.InformaticaFeatureModule Maven / Gradle / Ivy
package org.etlunit.feature.informatica;
import com.google.inject.Binder;
import com.google.inject.Injector;
import com.google.inject.Module;
import org.etlunit.*;
import org.etlunit.feature.AbstractFeature;
import org.etlunit.feature.FeatureModule;
import org.etlunit.feature.RuntimeOption;
import org.etlunit.feature.execute.ExecuteFeatureModule;
import org.etlunit.feature.file.FileRuntimeSupport;
import org.etlunit.feature.informatica.util.*;
import org.etlunit.io.FileBuilder;
import org.etlunit.parser.ETLTestValueObject;
import org.etlunit.util.IOUtils;
import org.etlunit.util.StringUtils;
import org.etlunit.util.VelocityUtil;
import javax.inject.Inject;
import javax.inject.Named;
import java.io.File;
import java.io.IOException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@FeatureModule
public class InformaticaFeatureModule extends AbstractFeature {
private static final List prerequisites = Arrays.asList("logging", "execute", "file");
private ExecuteFeatureModule executeFeatureModule;
private File etlBinDir;
private InformaticaClassListener informaticaClassListener;
private InformaticaConfiguration informaticaConfiguration;
private InformaticaDirector informaticaDirector;
private RuntimeSupport runtimeSupport;
private FileRuntimeSupport fileRuntimeSupport;
private File etlDomainFile;
private boolean clientOutOfProcess = false;
@Override
public void dispose()
{
for (Map.Entry domain : informaticaConfiguration.getDomains().entrySet())
{
for (InformaticaRepository repo : domain.getValue().getRepositories())
{
try
{
repo.dispose();
}
catch(Exception exc)
{
System.err.println(exc);
}
}
for (InformaticaIntegrationService is: domain.getValue().getIntegrationServices())
{
try
{
is.dispose();
}
catch(Exception exc)
{
System.err.println(exc);
}
}
}
}
@Inject
public void receiveFileRuntimeSupport(FileRuntimeSupport support)
{
fileRuntimeSupport = support;
}
@Inject
public void setClientOutOfProcess(@Named("informatica.clientOutOfProcess") RuntimeOption option)
{
clientOutOfProcess = option.isEnabled();
applicationLog.info(clientOutOfProcess ? "Using out of process client" : "Using process-per-request client.");
}
@Override
protected Injector preCreateSub(Injector inj1)
{
etlDomainFile = new File(runtimeSupport.getFeatureConfigurationDirectory("informatica"), "domains.infa");
if (!etlDomainFile.exists()) {
try {
IOUtils.writeBufferToFile(etlDomainFile, new StringBuffer(""));
} catch (IOException e) {
applicationLog.severe("", e);
throw new IllegalArgumentException("Could not create infa domain file path = '" + etlDomainFile.getAbsolutePath() + "', message = '" + e.toString() + "'");
}
}
informaticaConfiguration = postCreate(new InformaticaConfiguration(this, featureConfiguration, runtimeSupport, etlDomainFile));
final InformaticaRuntimeSupport irs = postCreate(new InformaticaRuntimeSupportImpl());
return inj1.createChildInjector(new Module()
{
public void configure(Binder binder)
{
binder.bind(InformaticaRuntimeSupport.class).toInstance(irs);
binder.bind(InformaticaConfiguration.class).toInstance(informaticaConfiguration);
}
});
}
@Override
public void initialize(Injector inj1) {
informaticaDirector = postCreate(new InformaticaDirector());
fileRuntimeSupport.registerFileProducer(postCreate(new InformaticaFileProducer()));
if (featureConfiguration == null) {
throw new IllegalArgumentException("Missing informatica configuration");
}
ETLTestValueObject value = configuration.query("vendor-binary-directory");
if (value == null) {
throw new IllegalArgumentException("Configuration property 'vendor-binary-directory' not present");
}
etlBinDir = new File(value.getValueAsString());
File resourceDirectory = runtimeSupport.getFeatureSourceDirectory("informatica");
applicationLog.info("Using resource directory: " + resourceDirectory.getAbsolutePath());
InformaticaExecutor exe = new InformaticaExecutor(this, resourceDirectory, StringUtils.sanitize(runtimeSupport.getProjectUID(), '_'));
executeFeatureModule.extend(postCreate(exe));
informaticaClassListener = postCreate(new InformaticaClassListener(this));
}
@Override
public ClassListener getListener() {
return informaticaClassListener;
}
@Inject
public void installExecuteFeature(ExecuteFeatureModule f) {
// ugly cast
executeFeatureModule = f;
}
@Override
public List getPrerequisites() {
return prerequisites;
}
public String getFeatureName() {
return "informatica";
}
@Override
public ClassDirector getDirector()
{
return informaticaDirector;
}
@Inject
public void setRuntimeSupport(RuntimeSupport runtimeSupport) {
this.runtimeSupport = runtimeSupport;
}
/*
String integrationService(InformaticaRepository repository, ETLTestValueObject etlTestValueObject) throws TestExecutionError {
ETLTestValueObject is = etlTestValueObject.query("informatica-integration-service");
String effectiveIs = effectiveRepository.getDefaultIntegrationService();
if (is != null) {
String isValueAsString = is.getValueAsString();
if (!effectiveRepository.getIntegrationServices().contains(isValueAsString)) {
throw new IllegalArgumentException("Integration service " + isValueAsString + " not found in repository " + effectiveRepository.getRepositoryName() + " in domain " + effectiveDomain.getDomainName());
}
effectiveIs = isValueAsString;
}
}
*/
InformaticaIntegrationService integrationServiceForRequest(ETLTestValueObject etlTestValueObject) throws TestExecutionError
{
ETLTestValueObject domain = etlTestValueObject.query("informatica-domain");
ETLTestValueObject is = etlTestValueObject.query("informatica-integration-service");
InformaticaDomain effectiveDomain = informaticaConfiguration.getDefaultDomain();
if (domain != null) {
String domainValueAsString = domain.getValueAsString();
effectiveDomain = informaticaConfiguration.getDomains().get(domainValueAsString);
if (effectiveDomain == null) {
throw new IllegalArgumentException("Domain named " + domainValueAsString + " not found in Informatica configuration");
}
}
InformaticaIntegrationService effectiveIs = effectiveDomain.getDefaultIntegrationService();
if (is != null) {
String isValueAsString = is.getValueAsString();
effectiveIs = effectiveDomain.getIntegrationService(isValueAsString);
if (effectiveIs == null) {
throw new IllegalArgumentException("Integration service " + isValueAsString + " not found in domain " + effectiveDomain.getDomainName());
}
}
return effectiveIs;
}
InformaticaRepository repositoryForRequest(ETLTestValueObject etlTestValueObject) throws TestExecutionError
{
ETLTestValueObject domain = etlTestValueObject.query("informatica-domain");
ETLTestValueObject repository = etlTestValueObject.query("informatica-repository");
InformaticaDomain effectiveDomain = informaticaConfiguration.getDefaultDomain();
if (domain != null) {
String domainValueAsString = domain.getValueAsString();
effectiveDomain = informaticaConfiguration.getDomains().get(domainValueAsString);
if (effectiveDomain == null) {
throw new IllegalArgumentException("Domain named " + domainValueAsString + " not found in Informatica configuration");
}
}
InformaticaRepository effectiveRepository = effectiveDomain.getDefaultRepository();
if (repository != null) {
String repositoryValueAsString = repository.getValueAsString();
effectiveRepository = effectiveDomain.getRepository(repositoryValueAsString);
if (effectiveRepository == null) {
throw new IllegalArgumentException("Repository named " + repositoryValueAsString + " not found in Informatica configuration for domain " + effectiveDomain.getDomainName());
}
}
return effectiveRepository;
}
File getBinDir(InformaticaDomain domain)
{
// get the client version for the binary
String ver = domain.getClientVersion();
// construct a path to the power center binaries using a well-known path combined with the version number
return new FileBuilder(etlBinDir).subdir("PowerCenter").subdir(ver).subdir("server").subdir("bin").file();
}
InformaticaRepositoryClient getRepositoryClientImpl(InformaticaRepository repository) throws TestExecutionError {
File binDir = getBinDir(repository.getInformaticaDomain());
try {
if (clientOutOfProcess) {
applicationLog.info("Creating out of process client");
return new InformaticaOutOfProcessRepositoryClient(
repository,
binDir,
runtimeSupport,
applicationLog
);
}
applicationLog.info("Creating in process client");
return new InformaticaRepositoryClientImpl(
repository,
null,
binDir,
runtimeSupport,
applicationLog
);
} catch (Exception exc) {
throw new TestExecutionError("", InformaticaConstants.ERR_CREATE_CLIENT, exc);
}
}
public InformaticaIntegrationServiceClient getIntegrationServiceClient(InformaticaIntegrationService informaticaIntegrationService) throws Exception
{
File binDir = getBinDir(informaticaIntegrationService.getInformaticaDomain());
if (false) {
applicationLog.info("Creating out of process is client");
return new InformaticaOutOfProcessIntegrationServiceClient(
informaticaIntegrationService,
binDir,
runtimeSupport,
applicationLog
);
}
applicationLog.info("Creating in process is client");
return new InformaticaRepositoryClientImpl(
informaticaIntegrationService.getInformaticaDomain().getDefaultRepository(),
informaticaIntegrationService,
binDir,
runtimeSupport,
applicationLog
);
}
public InformaticaConfiguration getInformaticaConfiguration() {
return informaticaConfiguration;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy