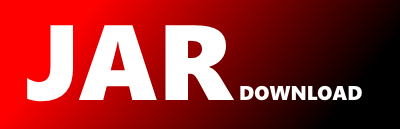
org.etlunit.feature.informatica.util.PmRepProcess Maven / Gradle / Ivy
package org.etlunit.feature.informatica.util;
import expectj.*;
import org.apache.commons.io.FileUtils;
import org.etlunit.ProcessDescription;
import org.etlunit.ProcessFacade;
import org.etlunit.RuntimeSupport;
import org.etlunit.io.Expectorator;
import org.etlunit.io.FileBuilder;
import java.io.*;
import java.util.Map;
public class PmRepProcess {
public static final String PMREP = "pmrep>";
public static final String NEWLINE = "\r\n";
private Expectorator expectorator;
private final ProcessFacade processFacade;
public static final long DEFAULT_TIMEOUT = 120000L;
public PmRepProcess(
File pmRep,
RuntimeSupport runtimeSupport,
Map environment,
String identifier,
File workingDir
) throws Exception {
ProcessDescription pd = new ProcessDescription(pmRep.getAbsolutePath());
pd.getEnvironment().putAll(environment);
File output = new FileBuilder(runtimeSupport.getReportDirectory("log"))
.subdir("process_log").subdir("informatica").mkdirs().name("pmrep-" + identifier + ".out").file();
pd.output(output).workingDirectory(workingDir);
// make sure the file is available
if (output.exists())
{
FileUtils.forceDelete(output);
}
try {
processFacade = runtimeSupport.execute(pd);
processFacade.waitForStreams();
expectorator = new Expectorator(new BufferedReader(processFacade.getReader()), processFacade.getWriter());
// wait for the first pmrep prompt
expectorator.expect(PMREP, DEFAULT_TIMEOUT);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public String send(String line) throws IOException {
String resp = send(line, DEFAULT_TIMEOUT);
return resp;
}
public String send(String line, long timeout) throws IOException {
if (expectorator == null)
{
throw new IllegalStateException("Pmrep client disposed");
}
try
{
String response = expectorator.sayAndExpect(line + NEWLINE, PMREP, timeout);
return response;
}
catch (InterruptedException e)
{
throw new IllegalStateException("Process timed out:" + line, e);
}
}
public void dispose() throws IOException
{
if (expectorator == null)
{
throw new IllegalStateException("Pmrep client disposed");
}
expectorator.say("exit");
processFacade.waitForCompletion();
expectorator = null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy