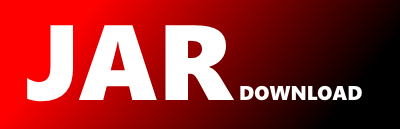
org.etlunit.regular_expression_compiler.RegularExpressionProxyClassGenerator Maven / Gradle / Ivy
package org.etlunit.regular_expression_compiler;
import java.io.File;
import java.io.FileFilter;
import java.io.FileInputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Properties;
public class RegularExpressionProxyClassGenerator {
private static final Map map = new HashMap();
private static final List toDoList = new ArrayList();
private static final List doingList = new ArrayList();
private static final List doneList = new ArrayList();
public static void generateProxyFromPropertiesDirectory(File propertiesDir, File outputDirectory) throws Exception
{
propertiesDir.listFiles(new FileFilter(){
public boolean accept(File pathname) {
try {
accept0(pathname);
} catch (Exception ex) {
throw new RuntimeException(pathname.toString(), ex);
}
return false;
}
private void accept0(File pathname) throws Exception {
if (pathname.isFile() && (pathname.getName().endsWith(".properties") || pathname.getName().endsWith(".xml")))
{
Properties properties = getProperties(pathname);
// get the classname
String fullName = RegularExpressionProxyCompiler.getClassPackageFromSpec(properties) + "." + RegularExpressionProxyCompiler.getClassNameFromSpec(properties);
RegExpSpec spec = new RegExpSpec(pathname, fullName, properties);
List groups = RegularExpressionProxyCompiler.getSubGroupsFromSpec(properties);
spec.addSubGroups(groups);
map.put(fullName, spec);
toDoList.add(spec);
}
}
});
while (toDoList.size() != 0)
{
RegExpSpec spec = toDoList.remove(0);
if (!doneList.contains(spec))
{
// and process
processSpec(spec, outputDirectory);
}
}
map.clear();
toDoList.clear();
doingList.clear();
doneList.clear();
}
private static void processSpec(RegExpSpec spec, File outputDirectory) throws Exception {
// add to the in progress list
doingList.add(spec);
if (spec.hasSubGroups())
{
// grab each sub group and if it hasn't been processed yet
// process it
List groups = spec.getSubGroups();
Iterator it = groups.iterator();
while (it.hasNext())
{
RegularExpressionProxyCompiler.SubGroup group = it.next();
// get the spec
RegExpSpec sg_spec = map.get(group.getFullClassName());
if (sg_spec == null)
{
throw new IllegalArgumentException("SubGroup reference not found: " + spec.getFile() + ", subgroup " + group.getGroupName() + ", " + group.getFullClassName());
}
// if this one is processed - skip
if (!doneList.contains(sg_spec))
{
// if this one is in process we have a circular dependency
if (doingList.contains(sg_spec))
{
throw new IllegalArgumentException("SubGroup reference causes circular dependency: " + spec.getFile() + ", subgroup " + group.getGroupName() + ", " + group.getFullClassName());
}
// process the sub group
processSpec(sg_spec, outputDirectory);
}
}
}
// do this one
generateProxyFromProperties(spec, outputDirectory);
doneList.add(spec);
}
public static Properties getProperties(File properties) throws IOException
{
System.out.println("Processing properties file: " + properties);
Properties props = new Properties();
FileInputStream fin = new FileInputStream(properties);
try
{
if (properties.getName().endsWith(".xml"))
{
props.loadFromXML(fin);
}
else
{
props.load(fin);
}
}
finally
{
fin.close();
}
return props;
}
private static void generateProxyFromProperties(RegExpSpec spec, File outputDirectory) throws IOException
{
System.out.println("Processing spec: " + spec.getFile());
StringBuffer classDefinition = RegularExpressionProxyCompiler.compile(spec.getProperties());
File file = new File(outputDirectory + "/" + spec.getProperties().getProperty("package").replace(".", "/"), spec.getProperties().getProperty("className") + ".java");
file.getParentFile().mkdirs();
FileWriter writer = new FileWriter(file);
try
{
writer.write(classDefinition.toString());
}
finally
{
writer.close();
}
}
}
class RegExpSpec
{
private final List subGroups = new ArrayList();
private final String className;
private final File file;
private final Properties properties;
public RegExpSpec(File pFile, String pClassName, Properties pProperties)
{
file = pFile;
className = pClassName;
properties = pProperties;
}
public String getClassName() {
return className;
}
public File getFile() {
return file;
}
public List getSubGroups()
{
return subGroups;
}
public void addSubGroups(List groups)
{
subGroups.addAll(groups);
}
public Properties getProperties()
{
return properties;
}
public boolean hasSubGroups()
{
return subGroups.size() != 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy