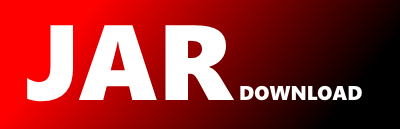
org.etlunit.regular_expression_compiler.RegularExpressionProxyCompiler Maven / Gradle / Ivy
package org.etlunit.regular_expression_compiler;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class RegularExpressionProxyCompiler {
public static class SubGroup
{
private static int REPEATING_GROUP = 0;
private static int SINGLE_GROUP = 1;
private final String groupName;
private final int type;
private final boolean optional;
private final String classPackage;
private final String className;
private SubGroup(String pGroupName, int pType, boolean pOptional, String pClassPackage, String pClassName)
{
groupName = pGroupName;
type = pType;
optional = pOptional;
classPackage = pClassPackage;
className = pClassName;
}
public String getFullClassName() {
return classPackage + "." + className;
}
public String getClassName() {
return className;
}
public String getClassPackage() {
return classPackage;
}
public String getGroupName() {
return groupName;
}
public boolean isOptional() {
return optional;
}
public int getType() {
return type;
}
public static SubGroup nextSubGroup(Properties props, String name)
{
String stype = props.getProperty("subGroup." + name + ".type");
int type = SINGLE_GROUP;
if (stype != null && stype.equalsIgnoreCase("repeating"))
{
type = REPEATING_GROUP;
}
boolean optional = false;
String soptional = props.getProperty("subGroup." + name + ".optional");
if (soptional != null)
{
optional = Boolean.valueOf(soptional);
}
String classReference = getProperty(props, "subGroup." + name + ".groupReference");
PackageExpression pe = PackageExpression.match(classReference);
if (!pe.hasNext())
{
throw new IllegalArgumentException("Bad class reference: " + classReference);
}
String pack = getClassPackageFromSpec(props);
if (pe.hasPackageName())
{
pack = pe.getPackageName();
}
return new SubGroup(
name,
type,
optional,
pack,
pe.getSimpleClassName()
);
}
}
public static List getSubGroupsFromSpec(Properties props)
{
List groups = new ArrayList();
SubGroupExpression sge = SubGroupExpression.match(getPatternFromSpec(props));
while (sge.hasNext())
{
String name = sge.getSubGroupName();
groups.add(SubGroup.nextSubGroup(props, name));
}
return groups;
}
public static String getClassNameFromSpec(Properties props)
{
return getProperty(props, "className");
}
public static String getPatternFromSpec(Properties props)
{
return getProperty(props, "pattern");
}
public static String getClassPackageFromSpec(Properties props)
{
return getProperty(props, "package");
}
public static StringBuffer compile(Properties properties) {
// get the class name and package
String className = getClassNameFromSpec(properties);
String classPackage = getClassPackageFromSpec(properties);
String superClassName = properties.getProperty("superClassName");
String interfaceName = properties.getProperty("interfaceName");
String pattern = getPatternFromSpec(properties);
// grab any sub groups
List sgroups = getSubGroupsFromSpec(properties);
// 'fix' the pattern
pattern = pattern.replace("\r\n", "[\\s]*").replace("\n", "[\\s]*").replace("\r", "[\\s]*").replace("\t", "[\\s]*");
pattern = escapeText(pattern);
pattern = expandPattern(pattern, sgroups);
// expand the pattern to include any sub groups
System.out.println("Verifying pattern: " + pattern);
// precompile
//Pattern ppattern = Pattern.compile(pattern, Pattern.CASE_INSENSITIVE);
//int groups = Integer.parseInt(getProperty(properties, "groups"));
//if (ppattern.matcher("").groupCount() < groups) {
// throw new IllegalArgumentException("Pattern (" + pattern + ") does not have " + groups + " groups");
//}
StringBuffer importBuffer = new StringBuffer("package " + classPackage + ";\n\n");
importBuffer.append("/*\n\n");
importBuffer.append("\t\tRegular expression class compiled by the Regular Expression Compiler 1.5.0-SNAPSHOT\n\n");
importBuffer.append("*/\n\n");
importBuffer.append("import java.util.Map;\n");
importBuffer.append("import java.util.HashMap;\n");
importBuffer.append("import java.util.regex.Pattern;\n");
importBuffer.append("import java.util.regex.Matcher;\n");
if (superClassName != null)
{
String iPack = getProperty(properties, "superPackage", classPackage);
if (!iPack.equals(classPackage))
{
importBuffer.append("import " + iPack + "." + superClassName + ";\n");
}
}
if (interfaceName != null)
{
String iPack = getProperty(properties, "interfacePackage", classPackage);
if (!iPack.equals(classPackage))
{
importBuffer.append("import " + iPack + "." + interfaceName + ";\n");
}
}
StringBuffer memberBuffer = new StringBuffer("\tpublic static final String PATTERN_RAW_TEXT = \"" + pattern + "\";\n");
boolean hasParams = false;
List paramList = new ArrayList();
Map paramSubMap = new HashMap();
StringBuffer paramBuffer = new StringBuffer();
StringBuffer argBuffer = new StringBuffer();
// create substitution parameter variables
ParameterExpression ps = ParameterExpression.match(pattern);
while (ps.hasNext())
{
String pn = ps.getParameterName();
memberBuffer.append("\tprivate final String parameter_" + pn + ";\n");
if (paramList.contains(pn))
{
throw new IllegalArgumentException("Duplicate parameter declared: " + pn);
}
paramList.add(pn);
if (hasParams)
{
paramBuffer.append(", ");
argBuffer.append(", ");
}
paramBuffer.append("String ");
paramBuffer.append(pn);
argBuffer.append(pn);
paramSubMap.put(pn, ps.group(0));
hasParams = true;
}
memberBuffer.append("\tpublic " + (hasParams ? "" : "static ") + "final String " + (hasParams ? "pattern_text" : "PATTERN_TEXT") + ";\n");
memberBuffer.append("\tpublic " + (hasParams ? "" : "static ") + "final Pattern pattern;\n");
memberBuffer.append("\tprivate " + (hasParams ? "" : "static ") + "final Map groupOffsets = new HashMap();\n\n");
memberBuffer.append("\tprivate final Matcher matcher;\n");
memberBuffer.append("\tprivate final CharSequence matchText;\n\n");
memberBuffer.append("\tpublic static interface RegExpIterator\n");
memberBuffer.append("\t{\n");
memberBuffer.append("\t\tString replaceMatch(" + className + " e);\n");
memberBuffer.append("\t}\n\n");
//System.out.println("RAW:" + PATTERN_RAW_TEXT);
//System.out.println("PARSED:" + PATTERN_TEXT);
//System.out.println("OFFSETS:" + groupOffsets);
StringBuffer toStringBuffer = new StringBuffer();
toStringBuffer.append("\tpublic String toString()\n");
toStringBuffer.append("\t{\n");
toStringBuffer.append("\t\treturn \"{" + className + ", matchText='\" + matchText + \"'}[\"");
StringBuffer methodBuffer = null;
if (hasParams)
{
methodBuffer = new StringBuffer("\tpublic " + className + "(CharSequence ch, " + paramBuffer.toString() + ")\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\tString s_pattern_text = PATTERN_RAW_TEXT.replaceAll(\"\\\\(\\\\?'[^']+'\", \"(\");\n\n");
Set> set = paramSubMap.entrySet();
Iterator> mapit = set.iterator();
while (mapit.hasNext())
{
Map.Entry entry = mapit.next();
methodBuffer.append("\t\t//" + entry.getKey() + " >> " + entry.getValue() + ";\n");
methodBuffer.append("\t\tparameter_" + entry.getKey() + " = " + entry.getKey() + ";\n\n");
methodBuffer.append("\t\ts_pattern_text = s_pattern_text.replace(\"" + entry.getValue() + "\", parameter_" + entry.getKey() + ");\n\n");
}
methodBuffer.append("\t\tpattern_text = s_pattern_text;\n\n");
methodBuffer.append("\t\tPattern cpattern = Pattern.compile(\"(\\\\\\\\)?\\\\((\\\\?'([^']+)')?\");\n\n");
methodBuffer.append("\t\tMatcher m = cpattern.matcher(PATTERN_RAW_TEXT);\n\n");
methodBuffer.append("\t\tint groupCount = 1;\n\n");
methodBuffer.append("\t\twhile (m.find())\n");
methodBuffer.append("\t\t{\n");
methodBuffer.append("\t\t\tif (m.group(1) != null)\n");
methodBuffer.append("\t\t\t{\n");
methodBuffer.append("\t\t\t\tcontinue;\n");
methodBuffer.append("\t\t\t}\n\n");
methodBuffer.append("\t\t\tString groupName = m.group(3);\n\n");
methodBuffer.append("\t\t\tif (groupName != null)\n");
methodBuffer.append("\t\t\t{\n");
methodBuffer.append("\t\t\t\tgroupOffsets.put(groupName, new Integer(groupCount));\n");
methodBuffer.append("\t\t\t}\n\n");
methodBuffer.append("\t\t\tgroupCount++;\n");
methodBuffer.append("\t\t}\n\n");
methodBuffer.append("\t\tpattern = Pattern.compile(pattern_text, Pattern.CASE_INSENSITIVE | Pattern.MULTILINE);\n");
methodBuffer.append("\n");
methodBuffer.append("\t\tmatchText = ch;\n");
methodBuffer.append("\t\tmatcher = pattern.matcher(matchText);\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic static " + className + " match(CharSequence pText, " + paramBuffer.toString() + ")\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn new " + className + "(pText, " + argBuffer.toString() + ");\n");
methodBuffer.append("\t}\n");
}
else
{
memberBuffer.append("\tstatic\n");
memberBuffer.append("\t{\n");
memberBuffer.append("\t\tPATTERN_TEXT = PATTERN_RAW_TEXT.replaceAll(\"\\\\(\\\\?'[^']+'\", \"(\");\n\n");
memberBuffer.append("\t\tPattern cpattern = Pattern.compile(\"(\\\\\\\\)?\\\\((\\\\?'([^']+)')?\");\n\n");
memberBuffer.append("\t\tMatcher matcher = cpattern.matcher(PATTERN_RAW_TEXT);\n\n");
memberBuffer.append("\t\tint groupCount = 1;\n\n");
memberBuffer.append("\t\twhile (matcher.find())\n");
memberBuffer.append("\t\t{\n");
memberBuffer.append("\t\t\tif (matcher.group(1) != null)\n");
memberBuffer.append("\t\t\t{\n");
memberBuffer.append("\t\t\t\tcontinue;\n");
memberBuffer.append("\t\t\t}\n\n");
memberBuffer.append("\t\t\tString groupName = matcher.group(3);\n\n");
memberBuffer.append("\t\t\tif (groupName != null)\n");
memberBuffer.append("\t\t\t{\n");
memberBuffer.append("\t\t\t\tgroupOffsets.put(groupName, new Integer(groupCount));\n");
memberBuffer.append("\t\t\t}\n\n");
memberBuffer.append("\t\t\tgroupCount++;\n");
memberBuffer.append("\t\t}\n\n");
memberBuffer.append("\t\tpattern = Pattern.compile(PATTERN_TEXT, Pattern.CASE_INSENSITIVE | Pattern.MULTILINE);\n");
memberBuffer.append("\t}\n\n");
methodBuffer = new StringBuffer("\tprivate " + className + "(Matcher pMatcher, CharSequence ch)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\tmatcher = pMatcher;\n");
methodBuffer.append("\t\tmatchText = ch;\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic " + className + "(CharSequence ch)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\tthis(pattern.matcher(ch), ch);\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic static " + className + " match(CharSequence pText)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn new " + className + "(pText);\n");
methodBuffer.append("\t}\n");
}
methodBuffer.append("\n");
methodBuffer.append("\tpublic int end()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.end();\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic int end(int group)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.end(group);\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic int start()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.start();\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic int start(int group)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.start(group);\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic String replaceAll(String replacement)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.replaceAll(replacement);\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic String replaceAll(RegExpIterator it)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\tString match = matchText.toString();\n");
methodBuffer.append("\t\tString str = \"\";\n");
methodBuffer.append("\t\tint end = -1;\n");
methodBuffer.append("\n");
methodBuffer.append("\t\twhile (hasNext())\n");
methodBuffer.append("\t\t{\n");
methodBuffer.append("\t\t\tstr += match.substring(end == -1 ? 0 : end, start());\n");
methodBuffer.append("\t\t\tstr += it.replaceMatch(this);\n");
methodBuffer.append("\t\t\tend = end();\n");
methodBuffer.append("\t\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\t\tstr += match.substring(end == -1 ? 0 : end, match.length());\n");
methodBuffer.append("\t\treturn str;\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic boolean matches()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.matches();\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic boolean hasNext()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.find();\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic int groupCount()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.groupCount();\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic String group()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.group();\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tpublic String group(int i)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.group(i);\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
if (hasParams)
{
methodBuffer.append("\tpublic " + className + " resetMatch(CharSequence seq, " + paramBuffer.toString() + ")\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn match(seq, " + argBuffer.toString() + ");\n");
methodBuffer.append("\t}\n");
}
else
{
methodBuffer.append("\tpublic " + className + " resetMatch(CharSequence seq)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn match(seq);\n");
methodBuffer.append("\t}\n");
}
methodBuffer.append("\n");
methodBuffer.append("\tpublic String group(String name)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn matcher.group(groupOffsets.get(name).intValue());\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
methodBuffer.append("\tprivate static String scope(String text, String name)\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn text.replaceAll(\"(\\\\(\\\\?')(\\\\w+')\", \"$1\" + name + \".$2\");\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
// create accessor methods for parameters
Iterator pit = paramList.iterator();
while (pit.hasNext())
{
String pn = pit.next();
methodBuffer.append("\tpublic final String get" + pn + "Parameter()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn parameter_" + pn + ";\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
}
Iterator sgit = sgroups.iterator();
while (sgit.hasNext())
{
SubGroup sg = sgit.next();
String groupPropertyName = getPropertyName(sg.getGroupName());
// add an import if needed
importBuffer.append("import " + sg.getFullClassName() + ";\n");
// get the raw sub group text
methodBuffer.append("\tpublic String get" + groupPropertyName + "Text()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn group(\"" + sg.getGroupName() + "\");\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
if (sg.isOptional())
{
// test the sub group
methodBuffer.append("\tpublic boolean has" + groupPropertyName + "()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn get" + groupPropertyName + "Text() != null;\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
}
// get the sub group
methodBuffer.append("\tpublic " + sg.getClassName() + " get" + groupPropertyName + "()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn " + sg.getClassName() + ".match(get" + groupPropertyName + "Text());\n");
methodBuffer.append("\t}\n");
methodBuffer.append("\n");
}
appendProperties(properties, importBuffer, memberBuffer, methodBuffer, toStringBuffer);
StringBuffer classBuffer = new StringBuffer();
classBuffer.append(importBuffer);
classBuffer.append("\n");
// declare the class with the correct interface and/or super class
classBuffer.append("public final class " + className + "");
if (superClassName != null)
{
classBuffer.append(" extends " + superClassName);
}
if (interfaceName != null)
{
classBuffer.append(" implements " + interfaceName);
}
classBuffer.append("\n");
classBuffer.append("{\n");
classBuffer.append(memberBuffer);
classBuffer.append("\n");
classBuffer.append(methodBuffer);
classBuffer.append("\n");
toStringBuffer.append(" + \"]\";\n");
toStringBuffer.append("\t}\n");
classBuffer.append(toStringBuffer);
classBuffer.append("\n");
classBuffer.append("\tpublic static void main(String [] argv)\n");
classBuffer.append("\t{\n");
classBuffer.append("\t\tSystem.out.println(" + (hasParams ? "PATTERN_RAW_TEXT" : "PATTERN_TEXT") + ");\n");
classBuffer.append("\t}\n");
classBuffer.append("}");
return classBuffer;
}
private static String expandPattern(String pattern, List groups) {
Iterator it = groups.iterator();
while (it.hasNext())
{
SubGroup sgroup = it.next();
Pattern regexp = Pattern.compile("\\(\\?\\\\\"" + sgroup.getGroupName() + "\\\\\"\\)");
Matcher matcher = regexp.matcher(pattern);
String replacement = "\" + scope(" + sgroup.getClassName() + ".PATTERN_TEXT, \"" + sgroup.getGroupName() + "\") + \"";
boolean repeating = sgroup.getType() == SubGroup.REPEATING_GROUP;
/*
{
if (sgroup.isOptional())
{
replacement = replacement + "*";
}
else
{
replacement = replacement + "+";
}
}
else
{
if (sgroup.isOptional())
{
replacement = replacement + "?";
}
}
*/
replacement = "(?'" + sgroup.getGroupName() + "'(" + replacement + ")" + (repeating ? (sgroup.isOptional() ? "*" : "+") : (sgroup.isOptional() ? "?" : "")) + ")";
System.out.println("Expanding \"" + pattern + "\" with subgroup " + sgroup.getGroupName());
pattern = matcher.replaceAll(replacement);
System.out.println("Expanded to \"" + pattern + "\"");
}
return pattern;
}
private static void appendProperties(Properties properties, StringBuffer importBuffer, StringBuffer memberBuffer, StringBuffer methodBuffer, StringBuffer toStringBuffer) {
methodBuffer.append("\n");
boolean dateImported = false;
boolean bdImported = false;
boolean urlImported = false;
GroupNameExpression gne = GroupNameExpression.match(getPatternFromSpec(properties));
boolean first = true;
while (gne.hasNext())
{
if (gne.hasEscape())
{
continue;
}
String propName = gne.getGroupName();
boolean needsTryCatch = false;
String exceptionType = null;
if (!first) {
methodBuffer.append("\n");
}
String type = properties.getProperty("group." + propName + ".type");
String propetyName = getPropertyName(propName);
toStringBuffer.append(" + \"" + (!first ? ", " : "") + "(" + propName + ")=\" + (has" + propetyName + "() ? get" + propetyName + "() : \"null\")");
if (type == null) {
type = "String";
}
String typeName = "String";
String typeAccessor = "group(\"" + propName + "\")";
if (type.equals("int")) {
typeName = "int";
typeAccessor = "Integer.parseInt(" + typeAccessor + ")";
} else if (type.equals("char")) {
typeName = "char";
typeAccessor = typeAccessor + ".charAt(0)";
} else if (type.equals("BigDecimal")) {
typeName = "BigDecimal";
typeAccessor = "new BigDecimal(" + typeAccessor + ")";
if (!bdImported)
{
importBuffer.append("import java.math.BigDecimal;\n");
bdImported = true;
}
} else if (type.equals("URL")) {
typeName = "URL";
typeAccessor = "new URL(" + typeAccessor + ")";
if (!urlImported)
{
importBuffer.append("import java.net.URL;\n");
importBuffer.append("import java.net.MalformedURLException;\n");
urlImported = true;
}
needsTryCatch = true;
exceptionType = "MalformedURLException";
} else if (type.equals("Integer")) {
typeName = "Integer";
typeAccessor = "new Integer(" + typeAccessor + ")";
} else if (type.equals("long")) {
typeName = "long";
typeAccessor = "Long.parseLong(" + typeAccessor + ")";
} else if (type.equals("Long")) {
typeName = "Long";
typeAccessor = "new Long(" + typeAccessor + ")";
} else if (type.equals("group")) {
typeName = getProperty(properties, "group." + propName + ".groupClass");
typeAccessor = typeName + ".match(" + typeAccessor + ")";
} else if (type.equals("Date")) {
if (!dateImported)
{
importBuffer.append("import java.text.ParseException;\n");
importBuffer.append("import java.text.DateFormat;\n");
importBuffer.append("import java.text.SimpleDateFormat;\n");
importBuffer.append("import java.util.Date;\n");
dateImported = true;
}
String format = getProperty(properties, "group." + propName + ".format");
// precompile date format
DateFormat df = new SimpleDateFormat(format);
memberBuffer.append("\tprivate final DateFormat group_" + propName + "_format = new SimpleDateFormat(\"" + escapeText(format) + "\");\n");
typeName = "Date";
typeAccessor = "group_" + propName + "_format.parse(" + typeAccessor + ")";
needsTryCatch = true;
exceptionType = "ParseException";
} else if (type.equals("String")) {
} else {
throw new IllegalArgumentException("Bad property type: " + propName + ", " + type);
}
// create a boolean accessor first
methodBuffer.append("\tpublic boolean has" + propetyName + "()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\treturn group(\"" + propName + "\") != null;\n");
methodBuffer.append("\t}\n\n");
methodBuffer.append("\tpublic " + typeName + " get" + propetyName + "()\n");
methodBuffer.append("\t{\n");
methodBuffer.append("\t\tif (!has" + propetyName + "())\n");
methodBuffer.append("\t\t{\n");
methodBuffer.append("\t\t\tthrow new IllegalArgumentException(\"Property not defined: " + propetyName + "\");\n");
methodBuffer.append("\t\t}\n");
if (needsTryCatch)
{
methodBuffer.append("\t\ttry\n");
methodBuffer.append("\t\t{\n");
methodBuffer.append("\t\t\treturn " + typeAccessor + ";\n");
methodBuffer.append("\t\t}\n");
methodBuffer.append("\t\tcatch(" + exceptionType + " exc)\n");
methodBuffer.append("\t\t{\n");
methodBuffer.append("\t\t\tthrow new IllegalArgumentException(group(\"" + propName + "\"));\n");
methodBuffer.append("\t\t}\n");
}
else
{
methodBuffer.append("\t\treturn " + typeAccessor + ";\n");
}
methodBuffer.append("\t}\n");
first = false;
}
}
private static String escapeText(String format) {
return format.replace("\\", "\\\\").replace("\"", "\\\"");
}
private static String getProperty(Properties properties, String string) {
return getProperty(properties, string, null);
}
private static String getProperty(Properties properties, String string, String def) {
String val = properties.getProperty(string);
if (val == null || val.trim().equals("")) {
if (def != null)
{
return def;
}
throw new IllegalArgumentException("Missing property: " + string + " props=" + properties);
}
return val.trim();
}
private static String getPropertyName(String name) {
return Character.toUpperCase(name.charAt(0)) + "" + (name.length() > 1 ? name.substring(1) : "");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy