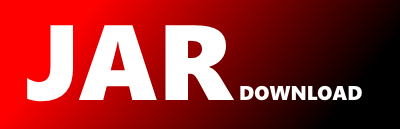
com.googlecode.fightinglayoutbugs.FightingLayoutBugs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fighting-layout-bugs Show documentation
Show all versions of fighting-layout-bugs Show documentation
A library for automatic detection of layout bugs in web pages
/*
* Copyright 2009-2011 Michael Tamm
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.fightinglayoutbugs;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import java.util.*;
/**
* Finds layout bugs in a web page by executing a certain
* set of {@link LayoutBugDetector}s. By default the following
* detectors are enabled:
*
* - {@link DetectNeedsHorizontalScrolling}
* - {@link DetectInvalidImageUrls}
* - {@link DetectTextNearOrOverlappingHorizontalEdge}
* - {@link DetectTextNearOrOverlappingVerticalEdge}
* - {@link DetectTextWithTooLowContrast}
*
*
* @author Michael Tamm
*/
public class FightingLayoutBugs extends AbstractLayoutBugDetector {
private static final Log LOG = LogFactory.getLog(FightingLayoutBugs.class);
private TextDetector _textDetector;
private EdgeDetector _edgeDetector;
private final List _detectors = new ArrayList();
/**
* Registers the following detectors:
* - {@link DetectNeedsHorizontalScrolling}
* - {@link DetectInvalidImageUrls}
* - {@link DetectTextNearOrOverlappingHorizontalEdge}
* - {@link DetectTextNearOrOverlappingVerticalEdge}
* - {@link DetectTextWithTooLowContrast}
*
*/
public FightingLayoutBugs() {
// The first detector should always be DetectNeedsHorizontalScrolling, because it resizes the browser window ...
this(
new DetectNeedsHorizontalScrolling(),
new DetectInvalidImageUrls(),
new DetectTextNearOrOverlappingHorizontalEdge(),
new DetectTextNearOrOverlappingVerticalEdge(),
new DetectTextWithTooLowContrast()
);
}
public FightingLayoutBugs(LayoutBugDetector... detectors) {
// The first detector should always be DetectNeedsHorizontalScrolling, because it resizes the browser window ...
for (LayoutBugDetector d : detectors) {
enable(d);
}
}
/**
* Sets the {@link TextDetector} to use.
*/
public void setTextDetector(TextDetector textDetector) {
_textDetector = textDetector;
}
/**
* Sets the {@link EdgeDetector} to use.
*/
public void setEdgeDetector(EdgeDetector edgeDetector) {
_edgeDetector = edgeDetector;
}
/**
* Adds the given detector to the set of detectors, which will be executed,
* when {@link #findLayoutBugsIn(WebPage) findLayoutBugsIn(...)} is called.
* If there is already a detector of the same class registered, it will be
* replaced by the given detector.
*/
public void enable(LayoutBugDetector detector) {
if (detector == null) {
throw new IllegalArgumentException("Method parameter newDetector must not be null.");
}
disable(detector.getClass());
_detectors.add(detector);
}
/**
* Removes all detectors of the given class from the set of detectors, which will be executed,
* when {@link #findLayoutBugsIn(WebPage) findLayoutBugsIn(...)} is called.
*/
public void disable(Class extends LayoutBugDetector> detectorClass) {
if (detectorClass == null) {
throw new IllegalArgumentException("Method parameter detectorClass must not be null.");
}
Iterator i = _detectors.iterator();
while (i.hasNext()) {
LayoutBugDetector detector = i.next();
if (detector.getClass().isAssignableFrom(detectorClass) || detectorClass.isAssignableFrom(detector.getClass())) {
i.remove();
}
}
}
/**
* Call this method to gain access to one of the {@link LayoutBugDetector}s
* for calling setter methods on it.
*/
public D configure(Class detectorClass) {
if (detectorClass == null) {
throw new IllegalArgumentException("Method parameter detectorClass must not be null.");
}
for (LayoutBugDetector detector : _detectors) {
if (detectorClass.isAssignableFrom(detector.getClass())) {
// noinspection unchecked
return (D) detector;
}
}
throw new IllegalArgumentException("There is no detector of class " + detectorClass.getName());
}
/**
* Runs all registered {@link LayoutBugDetector}s. Before you call this method, you might:
* - register new detectors via {@link #enable},
* - remove unwanted detectors via {@link #disable},
* - configure a registered detector via {@link #configure},
* - configure the {@link TextDetector} to be used via {@link #setTextDetector},
* - configure the {@link EdgeDetector} to be used via {@link #setEdgeDetector}.
*
*/
public Collection findLayoutBugsIn(WebPage webPage) {
webPage.setTextDetector(_textDetector == null ? new AnimationAwareTextDetector() : _textDetector);
webPage.setEdgeDetector(_edgeDetector == null ? new SimpleEdgeDetector() : _edgeDetector);
final Collection result = new ArrayList();
for (LayoutBugDetector detector : _detectors) {
detector.setScreenshotDir(screenshotDir);
LOG.debug("Running " + detector.getClass().getSimpleName() + " ...");
result.addAll(detector.findLayoutBugsIn(webPage));
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy