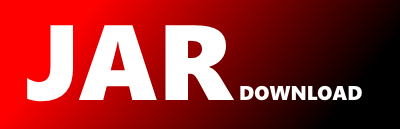
com.googlecode.fightinglayoutbugs.DetectInvalidImageUrls Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fighting-layout-bugs Show documentation
Show all versions of fighting-layout-bugs Show documentation
A library for automatic detection of layout bugs in web pages
The newest version!
/*
* Copyright 2009-2012 Michael Tamm
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.fightinglayoutbugs;
import org.apache.commons.httpclient.*;
import org.apache.commons.httpclient.methods.GetMethod;
import org.apache.commons.io.IOUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.net.MalformedURLException;
import java.net.URISyntaxException;
import java.net.URL;
import java.nio.charset.Charset;
import java.util.*;
import java.util.concurrent.*;
import java.util.concurrent.atomic.AtomicInteger;
import static java.lang.Character.isWhitespace;
/**
* Detects invalid image URLs in the HTML source of the analyzed web page as well
* as all directly or indirectly referenced CSS resources.
*/
public class DetectInvalidImageUrls extends AbstractLayoutBugDetector {
private static final Log LOG = LogFactory.getLog(DetectInvalidImageUrls.class);
static String stripCommentsFrom(String css) {
final int n = css.length();
int j = css.indexOf("/*");
final String result;
if (j == -1) {
result = css;
} else {
final StringBuilder sb = new StringBuilder(n);
int i = 0;
do {
sb.append(css, i, j);
i = css.indexOf("*/", i) + 2;
if (i == 1) {
i = n;
}
j = css.indexOf("/*", i);
} while (j != -1);
sb.append(css, i, n);
result = sb.toString();
}
return result;
}
private static boolean isValidCharset(String charset) {
boolean result = false;
if (charset != null && charset.length() > 0) {
try {
result = (Charset.forName(charset) != null);
} catch (Exception ignored) {}
}
return result;
}
private static boolean hasProtocol(String url) {
boolean result = false;
if (url != null) {
final int i = url.indexOf(':');
final int j = url.indexOf('?');
result = (i > 0 && (j == -1 || i < j));
}
return result;
}
/**
* ""
as value means the image URL is either currently being checked or valid,
* all other values are the error message for the image URL.
*/
private final ConcurrentMap _checkedImageUrls = new ConcurrentHashMap();
private WebPage _webPage;
private URL _baseUrl;
private String _documentCharset;
private boolean _screenshotTaken;
private Set _checkedCssUrls;
private String _faviconUrl;
private List _layoutBugs;
private HttpClient _httpClient;
private MockBrowser _mockBrowser;
public Collection findLayoutBugsIn(@Nonnull WebPage webPage) {
try {
_webPage = webPage;
_baseUrl = _webPage.getUrl();
_documentCharset = (String) _webPage.executeJavaScript("return document.characterSet");
_screenshotTaken = false;
_checkedCssUrls = new ConcurrentSkipListSet();
_faviconUrl = "/favicon.ico";
_layoutBugs = new ArrayList();
_mockBrowser = new MockBrowser(_httpClient == null ? new HttpClient(new MultiThreadedHttpConnectionManager()) : _httpClient);
try {
// 1. Check the src attribute of all visible
elements ...
checkVisibleImgElements();
// 2. Check the style attribute of all elements ...
checkStyleAttributes();
// 3. Check all