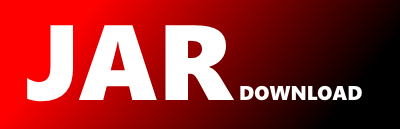
com.googlecode.genericdao.dao.hibernate.GenericDAOImpl Maven / Gradle / Ivy
The newest version!
/* Copyright 2013 David Wolverton
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.googlecode.genericdao.dao.hibernate;
import java.io.Serializable;
import java.util.List;
import com.googlecode.genericdao.dao.DAOUtil;
import com.googlecode.genericdao.search.ExampleOptions;
import com.googlecode.genericdao.search.Filter;
import com.googlecode.genericdao.search.ISearch;
import com.googlecode.genericdao.search.Search;
import com.googlecode.genericdao.search.SearchResult;
/**
* Implementation of GenericDAO
using Hibernate.
* The SessionFactory property is annotated for automatic resource injection.
*
* @author dwolverton
*
* @param
* The type of the domain object for which this instance is to be
* used.
* @param
* The type of the id of the domain object for which this instance is
* to be used.
*/
@SuppressWarnings("unchecked")
public class GenericDAOImpl extends
HibernateBaseDAO implements GenericDAO {
protected Class persistentClass = (Class) DAOUtil.getTypeArguments(GenericDAOImpl.class, this.getClass()).get(0);
public int count(ISearch search) {
if (search == null)
search = new Search();
return _count(persistentClass, search);
}
public T find(Serializable id) {
return _get(persistentClass, id);
}
public T[] find(Serializable... ids) {
return _get(persistentClass, ids);
}
public List findAll() {
return _all(persistentClass);
}
public void flush() {
_flush();
}
public T getReference(Serializable id) {
return _load(persistentClass, id);
}
public T[] getReferences(Serializable... ids) {
return _load(persistentClass, ids);
}
public boolean isAttached(T entity) {
return _sessionContains(entity);
}
public void refresh(T... entities) {
_refresh(entities);
}
public boolean remove(T entity) {
return _deleteEntity(entity);
}
public void remove(T... entities) {
_deleteEntities(entities);
}
public boolean removeById(Serializable id) {
return _deleteById(persistentClass, id);
}
public void removeByIds(Serializable... ids) {
_deleteById(persistentClass, ids);
}
public boolean save(T entity) {
return _saveOrUpdateIsNew(entity);
}
public boolean[] save(T... entities) {
return _saveOrUpdateIsNew(entities);
}
public
© 2015 - 2025 Weber Informatics LLC | Privacy Policy