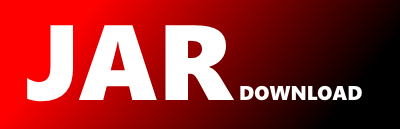
javadoc.com.google.common.collect.ImmutableList.html Maven / Gradle / Ivy
ImmutableList (Guava: Google Core Libraries for Java 11.0.1 API)
Overview
Package
Class
Use
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
com.google.common.collect
Class ImmutableList<E>
java.lang.Object
com.google.common.collect.ImmutableCollection<E>
com.google.common.collect.ImmutableList<E>
- All Implemented Interfaces:
- Serializable, Iterable<E>, Collection<E>, List<E>, RandomAccess
@GwtCompatible(serializable=true,
emulated=true)
public abstract class ImmutableList<E>
- extends ImmutableCollection<E>
- implements List<E>, RandomAccess
A high-performance, immutable, random-access List
implementation.
Does not permit null elements.
Unlike Collections.unmodifiableList(java.util.List extends T>)
, which is a view of a
separate collection that can still change, an instance of ImmutableList
contains its own private data and will never change.
ImmutableList
is convenient for public static final
lists
("constant lists") and also lets you easily make a "defensive copy" of a list
provided to your class by a caller.
Note: Although this class is not final, it cannot be subclassed as it has no public or protected constructors. Thus, instances of this type are guaranteed to be immutable.
- Since:
- 2.0 (imported from Google Collections Library)
- Author:
- Kevin Bourrillion
- See Also:
ImmutableMap
,ImmutableSet
, Serialized Form
Nested Class Summary | |
---|---|
static class |
ImmutableList.Builder<E>
A builder for creating immutable list instances, especially public
static final lists ("constant lists"). |
Method Summary | ||
---|---|---|
void |
add(int index,
E element)
Guaranteed to throw an exception and leave the list unmodified. |
|
boolean |
addAll(int index,
Collection<? extends E> newElements)
Guaranteed to throw an exception and leave the list unmodified. |
|
ImmutableList<E> |
asList()
Returns this list instance. |
|
static
|
builder()
Returns a new builder. |
|
static
|
copyOf(Collection<? extends E> elements)
Returns an immutable list containing the given elements, in order. |
|
static
|
copyOf(E[] elements)
Returns an immutable list containing the given elements, in order. |
|
static
|
copyOf(Iterable<? extends E> elements)
Returns an immutable list containing the given elements, in order. |
|
static
|
copyOf(Iterator<? extends E> elements)
Returns an immutable list containing the given elements, in order. |
|
boolean |
equals(Object obj)
|
|
int |
hashCode()
|
|
abstract int |
indexOf(Object object)
|
|
UnmodifiableIterator<E> |
iterator()
Returns an unmodifiable iterator across the elements in this collection. |
|
abstract int |
lastIndexOf(Object object)
|
|
UnmodifiableListIterator<E> |
listIterator()
|
|
abstract UnmodifiableListIterator<E> |
listIterator(int index)
|
|
static
|
of()
Returns the empty immutable list. |
|
static
|
of(E element)
Returns an immutable list containing a single element. |
|
static
|
of(E e1,
E e2)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5,
E e6)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5,
E e6,
E e7)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5,
E e6,
E e7,
E e8)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5,
E e6,
E e7,
E e8,
E e9)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5,
E e6,
E e7,
E e8,
E e9,
E e10)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5,
E e6,
E e7,
E e8,
E e9,
E e10,
E e11)
Returns an immutable list containing the given elements, in order. |
|
static
|
of(E e1,
E e2,
E e3,
E e4,
E e5,
E e6,
E e7,
E e8,
E e9,
E e10,
E e11,
E e12,
E... others)
Returns an immutable list containing the given elements, in order. |
|
E |
remove(int index)
Guaranteed to throw an exception and leave the list unmodified. |
|
ImmutableList<E> |
reverse()
Returns a view of this immutable list in reverse order. |
|
E |
set(int index,
E element)
Guaranteed to throw an exception and leave the list unmodified. |
|
abstract ImmutableList<E> |
subList(int fromIndex,
int toIndex)
Returns an immutable list of the elements between the specified fromIndex , inclusive, and toIndex , exclusive. |
Methods inherited from class com.google.common.collect.ImmutableCollection |
---|
add, addAll, clear, contains, containsAll, isEmpty, remove, removeAll, retainAll, toArray, toArray, toString |
Methods inherited from class java.lang.Object |
---|
clone, finalize, getClass, notify, notifyAll, wait, wait, wait |
Methods inherited from interface java.util.List |
---|
add, addAll, clear, contains, containsAll, get, isEmpty, remove, removeAll, retainAll, size, toArray, toArray |
Method Detail |
---|
of
public static <E> ImmutableList<E> of()
- Returns the empty immutable list. This set behaves and performs comparably
to
Collections.emptyList()
, and is preferable mainly for consistency and maintainability of your code.
of
public static <E> ImmutableList<E> of(E element)
- Returns an immutable list containing a single element. This list behaves
and performs comparably to
Collections.singleton(T)
, but will not accept a null element. It is preferable mainly for consistency and maintainability of your code.- Throws:
NullPointerException
- ifelement
is null
of
public static <E> ImmutableList<E> of(E e1, E e2)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4, E e5)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4, E e5, E e6)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7, E e8)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7, E e8, E e9)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7, E e8, E e9, E e10)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7, E e8, E e9, E e10, E e11)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null
of
public static <E> ImmutableList<E> of(E e1, E e2, E e3, E e4, E e5, E e6, E e7, E e8, E e9, E e10, E e11, E e12, E... others)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any element is null- Since:
- 3.0 (source-compatible since 2.0)
copyOf
public static <E> ImmutableList<E> copyOf(Iterable<? extends E> elements)
- Returns an immutable list containing the given elements, in order. If
elements
is aCollection
, this method behaves exactly ascopyOf(Collection)
; otherwise, it behaves exactly ascopyOf(elements.iterator()
.- Throws:
NullPointerException
- if any ofelements
is null
copyOf
public static <E> ImmutableList<E> copyOf(Collection<? extends E> elements)
- Returns an immutable list containing the given elements, in order.
Despite the method name, this method attempts to avoid actually copying the data when it is safe to do so. The exact circumstances under which a copy will or will not be performed are undocumented and subject to change.
Note that if
list
is aList<String>
, thenImmutableList.copyOf(list)
returns anImmutableList<String>
containing each of the strings inlist
, while ImmutableList.of(list)} returns anImmutableList<List<String>>
containing one element (the given list itself).This method is safe to use even when
elements
is a synchronized or concurrent collection that is currently being modified by another thread.- Throws:
NullPointerException
- if any ofelements
is null
copyOf
public static <E> ImmutableList<E> copyOf(Iterator<? extends E> elements)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any ofelements
is null
copyOf
public static <E> ImmutableList<E> copyOf(E[] elements)
- Returns an immutable list containing the given elements, in order.
- Throws:
NullPointerException
- if any ofelements
is null- Since:
- 3.0
iterator
public UnmodifiableIterator<E> iterator()
- Description copied from class:
ImmutableCollection
- Returns an unmodifiable iterator across the elements in this collection.
listIterator
public UnmodifiableListIterator<E> listIterator()
- Specified by:
listIterator
in interfaceList<E>
listIterator
public abstract UnmodifiableListIterator<E> listIterator(int index)
- Specified by:
listIterator
in interfaceList<E>
indexOf
public abstract int indexOf(@Nullable Object object)
lastIndexOf
public abstract int lastIndexOf(@Nullable Object object)
- Specified by:
lastIndexOf
in interfaceList<E>
subList
public abstract ImmutableList<E> subList(int fromIndex, int toIndex)
- Returns an immutable list of the elements between the specified
fromIndex
, inclusive, andtoIndex
, exclusive. (IffromIndex
andtoIndex
are equal, the empty immutable list is returned.)
addAll
public final boolean addAll(int index, Collection<? extends E> newElements)
- Guaranteed to throw an exception and leave the list unmodified.
- Throws:
UnsupportedOperationException
- always
set
public final E set(int index, E element)
- Guaranteed to throw an exception and leave the list unmodified.
- Throws:
UnsupportedOperationException
- always
add
public final void add(int index, E element)
- Guaranteed to throw an exception and leave the list unmodified.
- Throws:
UnsupportedOperationException
- always
remove
public final E remove(int index)
- Guaranteed to throw an exception and leave the list unmodified.
- Throws:
UnsupportedOperationException
- always
asList
public ImmutableList<E> asList()
- Returns this list instance.
- Overrides:
asList
in classImmutableCollection<E>
- Since:
- 2.0
reverse
public ImmutableList<E> reverse()
- Returns a view of this immutable list in reverse order. For example,
ImmutableList.of(1, 2, 3).reverse()
is equivalent toImmutableList.of(3, 2, 1)
.- Returns:
- a view of this immutable list in reverse order
- Since:
- 7.0
equals
public boolean equals(Object obj)
hashCode
public int hashCode()
builder
public static <E> ImmutableList.Builder<E> builder()
- Returns a new builder. The generated builder is equivalent to the builder
created by the
ImmutableList.Builder
constructor.
|
||||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |
Copyright © 2010-2012. All Rights Reserved.