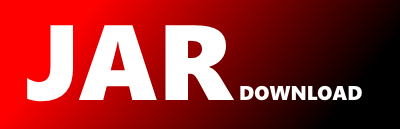
javadoc.com.google.common.primitives.UnsignedInteger.html Maven / Gradle / Ivy
The newest version!
UnsignedInteger (Guava: Google Core Libraries for Java 11.0.1 API)
Overview
Package
Class
Use
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
com.google.common.primitives
Class UnsignedInteger
java.lang.Object
java.lang.Number
com.google.common.primitives.UnsignedInteger
- All Implemented Interfaces:
- Serializable, Comparable<UnsignedInteger>
@Beta
@GwtCompatible(emulated=true)
public final class UnsignedInteger
- extends Number
- implements Comparable<UnsignedInteger>
A wrapper class for unsigned int
values, supporting arithmetic operations.
In some cases, when speed is more important than code readability, it may be faster simply to
treat primitive int
values as unsigned, using the methods from UnsignedInts
.
- Since:
- 11.0
- Author:
- Louis Wasserman
- See Also:
- Serialized Form
Field Summary | |
---|---|
static UnsignedInteger |
MAX_VALUE
|
static UnsignedInteger |
ONE
|
static UnsignedInteger |
ZERO
|
Method Summary | |
---|---|
UnsignedInteger |
add(UnsignedInteger val)
Returns the result of adding this and val . |
static UnsignedInteger |
asUnsigned(int value)
Returns an UnsignedInteger that, when treated as signed, is
equal to value . |
BigInteger |
bigIntegerValue()
Returns the value of this UnsignedInteger as a BigInteger . |
int |
compareTo(UnsignedInteger other)
Compares this unsigned integer to another unsigned integer. |
UnsignedInteger |
divide(UnsignedInteger val)
Returns the result of dividing this by val . |
double |
doubleValue()
Returns the value of this UnsignedInteger as a float , analogous to a widening
primitive conversion from int to double , and correctly rounded. |
boolean |
equals(Object obj)
|
float |
floatValue()
Returns the value of this UnsignedInteger as a float , analogous to a widening
primitive conversion from int to float , and correctly rounded. |
int |
hashCode()
|
int |
intValue()
Returns the value of this UnsignedInteger as an int . |
long |
longValue()
Returns the value of this UnsignedInteger as a long . |
UnsignedInteger |
multiply(UnsignedInteger val)
Returns the result of multiplying this and val . |
UnsignedInteger |
remainder(UnsignedInteger val)
Returns the remainder of dividing this by val . |
UnsignedInteger |
subtract(UnsignedInteger val)
Returns the result of subtracting this and val . |
String |
toString()
Returns a string representation of the UnsignedInteger value, in base 10. |
String |
toString(int radix)
Returns a string representation of the UnsignedInteger value, in base radix . |
static UnsignedInteger |
valueOf(BigInteger value)
Returns a UnsignedInteger representing the same value as the specified
BigInteger . |
static UnsignedInteger |
valueOf(long value)
Returns an UnsignedInteger that is equal to value ,
if possible. |
static UnsignedInteger |
valueOf(String string)
Returns an UnsignedInteger holding the value of the specified String , parsed
as an unsigned int value. |
static UnsignedInteger |
valueOf(String string,
int radix)
Returns an UnsignedInteger holding the value of the specified String , parsed
as an unsigned int value in the specified radix. |
Methods inherited from class java.lang.Number |
---|
byteValue, shortValue |
Methods inherited from class java.lang.Object |
---|
clone, finalize, getClass, notify, notifyAll, wait, wait, wait |
Field Detail |
---|
ZERO
public static final UnsignedInteger ZERO
ONE
public static final UnsignedInteger ONE
MAX_VALUE
public static final UnsignedInteger MAX_VALUE
Method Detail |
---|
asUnsigned
public static UnsignedInteger asUnsigned(int value)
- Returns an
UnsignedInteger
that, when treated as signed, is equal tovalue
.
valueOf
public static UnsignedInteger valueOf(long value)
- Returns an
UnsignedInteger
that is equal tovalue
, if possible. The inverse operation oflongValue()
.
valueOf
public static UnsignedInteger valueOf(BigInteger value)
- Returns a
UnsignedInteger
representing the same value as the specifiedBigInteger
. This is the inverse operation ofbigIntegerValue()
.- Throws:
IllegalArgumentException
- ifvalue
is negative orvalue >= 2^32
valueOf
public static UnsignedInteger valueOf(String string)
- Returns an
UnsignedInteger
holding the value of the specifiedString
, parsed as an unsignedint
value.- Throws:
NumberFormatException
- if the string does not contain a parsable unsignedint
value
valueOf
public static UnsignedInteger valueOf(String string, int radix)
- Returns an
UnsignedInteger
holding the value of the specifiedString
, parsed as an unsignedint
value in the specified radix.- Throws:
NumberFormatException
- if the string does not contain a parsable unsignedint
value
add
public UnsignedInteger add(UnsignedInteger val)
- Returns the result of adding this and
val
. If the result would have more than 32 bits, returns the low 32 bits of the result.
subtract
public UnsignedInteger subtract(UnsignedInteger val)
- Returns the result of subtracting this and
val
. If the result would be negative, returns the low 32 bits of the result.
multiply
@GwtIncompatible(value="Does not truncate correctly") public UnsignedInteger multiply(UnsignedInteger val)
- Returns the result of multiplying this and
val
. If the result would have more than 32 bits, returns the low 32 bits of the result.
divide
public UnsignedInteger divide(UnsignedInteger val)
- Returns the result of dividing this by
val
.
remainder
public UnsignedInteger remainder(UnsignedInteger val)
- Returns the remainder of dividing this by
val
.
intValue
public int intValue()
- Returns the value of this
UnsignedInteger
as anint
. This is an inverse operation toasUnsigned(int)
.Note that if this
UnsignedInteger
holds a value>= 2^31
, the returned value will be equal tothis - 2^32
.
longValue
public long longValue()
floatValue
public float floatValue()
- Returns the value of this
UnsignedInteger
as afloat
, analogous to a widening primitive conversion fromint
tofloat
, and correctly rounded.- Specified by:
floatValue
in classNumber
doubleValue
public double doubleValue()
- Returns the value of this
UnsignedInteger
as afloat
, analogous to a widening primitive conversion fromint
todouble
, and correctly rounded.- Specified by:
doubleValue
in classNumber
bigIntegerValue
public BigInteger bigIntegerValue()
- Returns the value of this
UnsignedInteger
as aBigInteger
.
compareTo
public int compareTo(UnsignedInteger other)
- Compares this unsigned integer to another unsigned integer.
Returns
0
if they are equal, a negative number ifthis < other
, and a positive number ifthis > other
.- Specified by:
compareTo
in interfaceComparable<UnsignedInteger>
hashCode
public int hashCode()
equals
public boolean equals(@Nullable Object obj)
toString
public String toString()
- Returns a string representation of the
UnsignedInteger
value, in base 10.
toString
public String toString(int radix)
- Returns a string representation of the
UnsignedInteger
value, in baseradix
. Ifradix < Character.MIN_RADIX
orradix > Character.MAX_RADIX
, the radix10
is used.
|
||||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |
Copyright © 2010-2012. All Rights Reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy