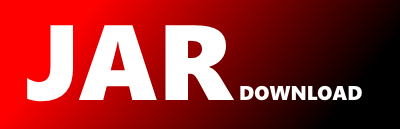
javadoc.com.google.common.primitives.UnsignedLong.html Maven / Gradle / Ivy
The newest version!
UnsignedLong (Guava: Google Core Libraries for Java 11.0.1 API)
Overview
Package
Class
Use
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
com.google.common.primitives
Class UnsignedLong
java.lang.Object
java.lang.Number
com.google.common.primitives.UnsignedLong
- All Implemented Interfaces:
- Serializable, Comparable<UnsignedLong>
@Beta
@GwtCompatible(serializable=true)
public class UnsignedLong
- extends Number
- implements Comparable<UnsignedLong>, Serializable
A wrapper class for unsigned long
values, supporting arithmetic operations.
In some cases, when speed is more important than code readability, it may be faster simply to
treat primitive long
values as unsigned, using the methods from UnsignedLongs
.
Please do not extend this class; it will be made final in the near future.
- Since:
- 11.0
- Author:
- Louis Wasserman, Colin Evans
- See Also:
- Serialized Form
Field Summary | |
---|---|
static UnsignedLong |
MAX_VALUE
|
static UnsignedLong |
ONE
|
static UnsignedLong |
ZERO
|
Constructor Summary | |
---|---|
protected |
UnsignedLong(long value)
|
Method Summary | |
---|---|
UnsignedLong |
add(UnsignedLong val)
Returns the result of adding this and val . |
static UnsignedLong |
asUnsigned(long value)
Returns an UnsignedLong that, when treated as signed, is equal to value . |
BigInteger |
bigIntegerValue()
Returns the value of this UnsignedLong as a BigInteger . |
int |
compareTo(UnsignedLong o)
|
UnsignedLong |
divide(UnsignedLong val)
Returns the result of dividing this by val . |
double |
doubleValue()
Returns the value of this UnsignedLong as a double , analogous to a widening
primitive conversion from long to double , and correctly rounded. |
boolean |
equals(Object obj)
|
float |
floatValue()
Returns the value of this UnsignedLong as a float , analogous to a widening
primitive conversion from long to float , and correctly rounded. |
int |
hashCode()
|
int |
intValue()
Returns the value of this UnsignedLong as an int . |
long |
longValue()
Returns the value of this UnsignedLong as a long . |
UnsignedLong |
multiply(UnsignedLong val)
Returns the result of multiplying this and val . |
UnsignedLong |
remainder(UnsignedLong val)
Returns the remainder of dividing this by val . |
UnsignedLong |
subtract(UnsignedLong val)
Returns the result of subtracting this and val . |
String |
toString()
Returns a string representation of the UnsignedLong value, in base 10. |
String |
toString(int radix)
Returns a string representation of the UnsignedLong value, in base radix . |
static UnsignedLong |
valueOf(BigInteger value)
Returns a UnsignedLong representing the same value as the specified BigInteger
. |
static UnsignedLong |
valueOf(String string)
Returns an UnsignedLong holding the value of the specified String , parsed as
an unsigned long value. |
static UnsignedLong |
valueOf(String string,
int radix)
Returns an UnsignedLong holding the value of the specified String , parsed as
an unsigned long value in the specified radix. |
Methods inherited from class java.lang.Number |
---|
byteValue, shortValue |
Methods inherited from class java.lang.Object |
---|
clone, finalize, getClass, notify, notifyAll, wait, wait, wait |
Field Detail |
---|
ZERO
public static final UnsignedLong ZERO
ONE
public static final UnsignedLong ONE
MAX_VALUE
public static final UnsignedLong MAX_VALUE
Constructor Detail |
---|
UnsignedLong
protected UnsignedLong(long value)
Method Detail |
---|
asUnsigned
public static UnsignedLong asUnsigned(long value)
- Returns an
UnsignedLong
that, when treated as signed, is equal tovalue
. The inverse operation islongValue()
.Put another way, if
value
is negative, the returned result will be equal to2^64 + value
; otherwise, the returned result will be equal tovalue
.
valueOf
public static UnsignedLong valueOf(BigInteger value)
- Returns a
UnsignedLong
representing the same value as the specifiedBigInteger
. This is the inverse operation ofbigIntegerValue()
.- Throws:
IllegalArgumentException
- ifvalue
is negative orvalue >= 2^64
valueOf
public static UnsignedLong valueOf(String string)
- Returns an
UnsignedLong
holding the value of the specifiedString
, parsed as an unsignedlong
value.- Throws:
NumberFormatException
- if the string does not contain a parsable unsignedlong
value
valueOf
public static UnsignedLong valueOf(String string, int radix)
- Returns an
UnsignedLong
holding the value of the specifiedString
, parsed as an unsignedlong
value in the specified radix.- Throws:
NumberFormatException
- if the string does not contain a parsable unsignedlong
value, orradix
is not betweenCharacter.MIN_RADIX
andCharacter.MAX_RADIX
add
public UnsignedLong add(UnsignedLong val)
- Returns the result of adding this and
val
. If the result would have more than 64 bits, returns the low 64 bits of the result.
subtract
public UnsignedLong subtract(UnsignedLong val)
- Returns the result of subtracting this and
val
. If the result would be negative, returns the low 64 bits of the result.
multiply
public UnsignedLong multiply(UnsignedLong val)
- Returns the result of multiplying this and
val
. If the result would have more than 64 bits, returns the low 64 bits of the result.
divide
public UnsignedLong divide(UnsignedLong val)
- Returns the result of dividing this by
val
.
remainder
public UnsignedLong remainder(UnsignedLong val)
- Returns the remainder of dividing this by
val
.
intValue
public int intValue()
longValue
public long longValue()
- Returns the value of this
UnsignedLong
as along
. This is an inverse operation toasUnsigned(long)
.Note that if this
UnsignedLong
holds a value>= 2^63
, the returned value will be equal tothis - 2^64
.
floatValue
public float floatValue()
- Returns the value of this
UnsignedLong
as afloat
, analogous to a widening primitive conversion fromlong
tofloat
, and correctly rounded.- Specified by:
floatValue
in classNumber
doubleValue
public double doubleValue()
- Returns the value of this
UnsignedLong
as adouble
, analogous to a widening primitive conversion fromlong
todouble
, and correctly rounded.- Specified by:
doubleValue
in classNumber
bigIntegerValue
public BigInteger bigIntegerValue()
- Returns the value of this
UnsignedLong
as aBigInteger
.
compareTo
public int compareTo(UnsignedLong o)
- Specified by:
compareTo
in interfaceComparable<UnsignedLong>
hashCode
public int hashCode()
equals
public boolean equals(@Nullable Object obj)
toString
public String toString()
- Returns a string representation of the
UnsignedLong
value, in base 10.
toString
public String toString(int radix)
- Returns a string representation of the
UnsignedLong
value, in baseradix
. Ifradix < Character.MIN_RADIX
orradix > Character.MAX_RADIX
, the radix10
is used.
|
||||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |
Copyright © 2010-2012. All Rights Reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy