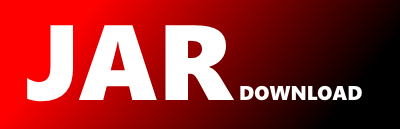
javadoc.com.google.common.util.concurrent.AtomicDouble.html Maven / Gradle / Ivy
AtomicDouble (Guava: Google Core Libraries for Java 11.0.1 API)
Overview
Package
Class
Use
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
com.google.common.util.concurrent
Class AtomicDouble
java.lang.Object
java.lang.Number
com.google.common.util.concurrent.AtomicDouble
- All Implemented Interfaces:
- Serializable
@Beta
public class AtomicDouble
- extends Number
- implements Serializable
A double
value that may be updated atomically. See the
java.util.concurrent.atomic
package specification for
description of the properties of atomic variables. An AtomicDouble
is used in applications such as atomic accumulation,
and cannot be used as a replacement for a Double
. However,
this class does extend Number
to allow uniform access by
tools and utilities that deal with numerically-based classes.
This class compares primitive double
values in methods such as compareAndSet(double, double)
by comparing their
bitwise representation using Double.doubleToRawLongBits(double)
,
which differs from both the primitive double ==
operator
and from Double.equals(java.lang.Object)
, as if implemented by:
static boolean bitEquals(double x, double y) {
long xBits = Double.doubleToRawLongBits(x);
long yBits = Double.doubleToRawLongBits(y);
return xBits == yBits;
}
It is possible to write a more scalable updater, at the cost of
giving up strict atomicity. See for example
and
.
Constructor Summary | |
---|---|
AtomicDouble()
Creates a new AtomicDouble with initial value 0.0 . |
|
AtomicDouble(double initialValue)
Creates a new AtomicDouble with the given initial value. |
Method Summary | |
---|---|
double |
addAndGet(double delta)
Atomically adds the given value to the current value. |
boolean |
compareAndSet(double expect,
double update)
Atomically sets the value to the given updated value if the current value is bitwise equal to the expected value. |
double |
doubleValue()
Returns the value of this AtomicDouble as a double . |
float |
floatValue()
Returns the value of this AtomicDouble as a float
after a narrowing primitive conversion. |
double |
get()
Gets the current value. |
double |
getAndAdd(double delta)
Atomically adds the given value to the current value. |
double |
getAndSet(double newValue)
Atomically sets to the given value and returns the old value. |
int |
intValue()
Returns the value of this AtomicDouble as an int
after a narrowing primitive conversion. |
void |
lazySet(double newValue)
Eventually sets to the given value. |
long |
longValue()
Returns the value of this AtomicDouble as a long
after a narrowing primitive conversion. |
void |
set(double newValue)
Sets to the given value. |
String |
toString()
Returns the String representation of the current value. |
boolean |
weakCompareAndSet(double expect,
double update)
Atomically sets the value to the given updated value if the current value is bitwise equal to the expected value. |
Methods inherited from class java.lang.Number |
---|
byteValue, shortValue |
Methods inherited from class java.lang.Object |
---|
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait |
Constructor Detail |
---|
AtomicDouble
public AtomicDouble(double initialValue)
- Creates a new
AtomicDouble
with the given initial value.- Parameters:
initialValue
- the initial value
AtomicDouble
public AtomicDouble()
- Creates a new
AtomicDouble
with initial value0.0
.
Method Detail |
---|
get
public final double get()
- Gets the current value.
- Returns:
- the current value
set
public final void set(double newValue)
- Sets to the given value.
- Parameters:
newValue
- the new value
lazySet
public final void lazySet(double newValue)
- Eventually sets to the given value.
- Parameters:
newValue
- the new value
getAndSet
public final double getAndSet(double newValue)
- Atomically sets to the given value and returns the old value.
- Parameters:
newValue
- the new value- Returns:
- the previous value
compareAndSet
public final boolean compareAndSet(double expect, double update)
- Atomically sets the value to the given updated value
if the current value is bitwise equal
to the expected value.
- Parameters:
expect
- the expected valueupdate
- the new value- Returns:
true
if successful. False return indicates that the actual value was not bitwise equal to the expected value.
weakCompareAndSet
public final boolean weakCompareAndSet(double expect, double update)
- Atomically sets the value to the given updated value
if the current value is bitwise equal
to the expected value.
May fail spuriously and does not provide ordering guarantees, so is only rarely an appropriate alternative to
compareAndSet
.- Parameters:
expect
- the expected valueupdate
- the new value- Returns:
true
if successful
getAndAdd
public final double getAndAdd(double delta)
- Atomically adds the given value to the current value.
- Parameters:
delta
- the value to add- Returns:
- the previous value
addAndGet
public final double addAndGet(double delta)
- Atomically adds the given value to the current value.
- Parameters:
delta
- the value to add- Returns:
- the updated value
toString
public String toString()
- Returns the String representation of the current value.
- Returns:
- the String representation of the current value
intValue
public int intValue()
- Returns the value of this
AtomicDouble
as anint
after a narrowing primitive conversion.
longValue
public long longValue()
- Returns the value of this
AtomicDouble
as along
after a narrowing primitive conversion.
floatValue
public float floatValue()
- Returns the value of this
AtomicDouble
as afloat
after a narrowing primitive conversion.- Specified by:
floatValue
in classNumber
doubleValue
public double doubleValue()
- Returns the value of this
AtomicDouble
as adouble
.- Specified by:
doubleValue
in classNumber
|
||||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | |||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |
Copyright © 2010-2012. All Rights Reserved.