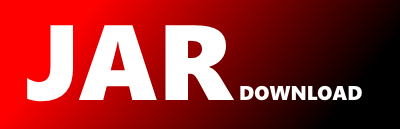
javadoc.src-html.com.google.common.base.Enums.html Maven / Gradle / Ivy
The newest version!
001 /*
002 * Copyright (C) 2011 The Guava Authors
003 *
004 * Licensed under the Apache License, Version 2.0 (the "License");
005 * you may not use this file except in compliance with the License.
006 * You may obtain a copy of the License at
007 *
008 * http://www.apache.org/licenses/LICENSE-2.0
009 *
010 * Unless required by applicable law or agreed to in writing, software
011 * distributed under the License is distributed on an "AS IS" BASIS,
012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
013 * See the License for the specific language governing permissions and
014 * limitations under the License.
015 */
016
017 package com.google.common.base;
018
019 import static com.google.common.base.Preconditions.checkNotNull;
020
021 import com.google.common.annotations.Beta;
022 import com.google.common.annotations.GwtCompatible;
023
024 import java.io.Serializable;
025
026 import javax.annotation.Nullable;
027
028 /**
029 * Utility methods for working with {@link Enum} instances.
030 *
031 * @author Steve McKay
032 *
033 * @since 9.0
034 */
035 @GwtCompatible
036 @Beta
037 public final class Enums {
038
039 private Enums() {}
040
041 /**
042 * Returns a {@link Function} that maps an {@link Enum} name to the associated
043 * {@code Enum} constant. The {@code Function} will return {@code null} if the
044 * {@code Enum} constant does not exist.
045 *
046 * @param enumClass the {@link Class} of the {@code Enum} declaring the
047 * constant values.
048 */
049 public static <T extends Enum<T>> Function<String, T> valueOfFunction(Class<T> enumClass) {
050 return new ValueOfFunction<T>(enumClass);
051 }
052
053 /**
054 * {@link Function} that maps an {@link Enum} name to the associated
055 * constant, or {@code null} if the constant does not exist.
056 */
057 private static final class ValueOfFunction<T extends Enum<T>> implements
058 Function<String, T>, Serializable {
059
060 private final Class<T> enumClass;
061
062 private ValueOfFunction(Class<T> enumClass) {
063 this.enumClass = checkNotNull(enumClass);
064 }
065
066 @Override
067 public T apply(String value) {
068 try {
069 return Enum.valueOf(enumClass, value);
070 } catch (IllegalArgumentException e) {
071 return null;
072 }
073 }
074
075 @Override public boolean equals(@Nullable Object obj) {
076 return obj instanceof ValueOfFunction &&
077 enumClass.equals(((ValueOfFunction) obj).enumClass);
078 }
079
080 @Override public int hashCode() {
081 return enumClass.hashCode();
082 }
083
084 @Override public String toString() {
085 return "Enums.valueOf(" + enumClass + ")";
086 }
087
088 private static final long serialVersionUID = 0;
089 }
090 }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy