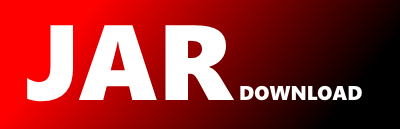
javadoc.src-html.com.google.common.cache.Cache.html Maven / Gradle / Ivy
The newest version!
001 /*
002 * Copyright (C) 2011 The Guava Authors
003 *
004 * Licensed under the Apache License, Version 2.0 (the "License");
005 * you may not use this file except in compliance with the License.
006 * You may obtain a copy of the License at
007 *
008 * http://www.apache.org/licenses/LICENSE-2.0
009 *
010 * Unless required by applicable law or agreed to in writing, software
011 * distributed under the License is distributed on an "AS IS" BASIS,
012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
013 * See the License for the specific language governing permissions and
014 * limitations under the License.
015 */
016
017 package com.google.common.cache;
018
019 import com.google.common.annotations.Beta;
020 import com.google.common.annotations.GwtCompatible;
021 import com.google.common.base.Function;
022 import com.google.common.collect.ImmutableMap;
023 import com.google.common.util.concurrent.ExecutionError;
024 import com.google.common.util.concurrent.UncheckedExecutionException;
025
026 import java.util.concurrent.Callable;
027 import java.util.concurrent.ConcurrentMap;
028 import java.util.concurrent.ExecutionException;
029
030 import javax.annotation.Nullable;
031
032 /**
033 * A semi-persistent mapping from keys to values. Cache entries are manually added using
034 * {@link #get(K, Callable)} or {@link #put(K, V)}, and are stored in the cache until either
035 * evicted or manually invalidated.
036 *
037 * <p><b>Note:</b> in release 12.0, all methods moved from {@code Cache} to {@link LoadingCache}
038 * will be deleted from {@code Cache}. As part of this transition {@code Cache} will no longer
039 * extend {@link Function}.
040 *
041 * <p>Implementations of this interface are expected to be thread-safe, and can be safely accessed
042 * by multiple concurrent threads.
043 *
044 * <p>All methods other than {@link #getIfPresent} are optional.
045 *
046 * @author Charles Fry
047 * @since 10.0
048 */
049 @Beta
050 @GwtCompatible
051 public interface Cache<K, V> extends Function<K, V> {
052
053 /**
054 * Returns the value associated with {@code key} in this cache, or {@code null} if there is no
055 * cached value for {@code key}.
056 *
057 * @since 11.0
058 */
059 @Nullable
060 V getIfPresent(K key);
061
062 /**
063 * Returns the value associated with {@code key} in this cache, obtaining that value from
064 * {@code valueLoader} if necessary. No observable state associated with this cache is modified
065 * until loading completes. This method provides a simple substitute for the conventional
066 * "if cached, return; otherwise create, cache and return" pattern.
067 *
068 * <p><b>Warning:</b> as with {@link CacheLoader#load}, {@code valueLoader} <b>must not</b> return
069 * {@code null}; it may either return a non-null value or throw an exception.
070 *
071 * @throws ExecutionException if a checked exception was thrown while loading the value
072 * @throws UncheckedExecutionException if an unchecked exception was thrown while loading the
073 * value
074 * @throws ExecutionError if an error was thrown while loading the value
075 *
076 * @since 11.0
077 */
078 V get(K key, Callable<? extends V> valueLoader) throws ExecutionException;
079
080 /**
081 * Returns a map of the values associated with {@code keys} in this cache. The returned map will
082 * only contain entries which are already present in the cache.
083 *
084 * @since 11.0
085 */
086 ImmutableMap<K, V> getAllPresent(Iterable<? extends K> keys);
087
088 /**
089 * Associates {@code value} with {@code key} in this cache. If the cache previously contained a
090 * value associated with {@code key}, the old value is replaced by {@code value}.
091 *
092 * <p>Prefer {@link #get(Object, Callable)} when using the conventional "if cached, return;
093 * otherwise create, cache and return" pattern.
094 *
095 * @since 11.0
096 */
097 void put(K key, V value);
098
099 /**
100 * Discards any cached value for key {@code key}.
101 */
102 void invalidate(Object key);
103
104 /**
105 * Discards any cached values for keys {@code keys}.
106 *
107 * @since 11.0
108 */
109 void invalidateAll(Iterable<?> keys);
110
111 /**
112 * Discards all entries in the cache.
113 */
114 void invalidateAll();
115
116 /**
117 * Returns the approximate number of entries in this cache.
118 */
119 long size();
120
121 /**
122 * Returns a current snapshot of this cache's cumulative statistics. All stats are initialized
123 * to zero, and are monotonically increasing over the lifetime of the cache.
124 */
125 CacheStats stats();
126
127 /**
128 * Returns a view of the entries stored in this cache as a thread-safe map. Modifications made to
129 * the map directly affect the cache.
130 */
131 ConcurrentMap<K, V> asMap();
132
133 /**
134 * Performs any pending maintenance operations needed by the cache. Exactly which activities are
135 * performed -- if any -- is implementation-dependent.
136 */
137 void cleanUp();
138
139 /**
140 * Returns the value associated with {@code key} in this cache, first loading that value if
141 * necessary. No observable state associated with this cache is modified until loading completes.
142 *
143 * @throws ExecutionException if a checked exception was thrown while loading the value
144 * @throws UncheckedExecutionException if an unchecked exception was thrown while loading the
145 * value
146 * @throws ExecutionError if an error was thrown while loading the value
147 * @deprecated This method has been split out into the {@link LoadingCache} interface, and will be
148 * removed from {@code Cache} in Guava release 12.0. Note that
149 * {@link CacheBuilder#build(CacheLoader)} now returns a {@code LoadingCache}, so this deprecation
150 * (migration) can be dealt with by simply changing the type of references to the results of
151 * {@link CacheBuilder#build(CacheLoader)}.
152 */
153 @Deprecated V get(K key) throws ExecutionException;
154
155 /**
156 * Returns the value associated with {@code key} in this cache, first loading that value if
157 * necessary. No observable state associated with this cache is modified until computation
158 * completes. Unlike {@link #get}, this method does not throw a checked exception, and thus should
159 * only be used in situations where checked exceptions are not thrown by the cache loader.
160 *
161 * <p><b>Warning:</b> this method silently converts checked exceptions to unchecked exceptions,
162 * and should not be used with cache loaders which throw checked exceptions.
163 *
164 * @throws UncheckedExecutionException if an exception was thrown while loading the value,
165 * regardless of whether the exception was checked or unchecked
166 * @throws ExecutionError if an error was thrown while loading the value
167 * @deprecated This method has been split out into the {@link LoadingCache} interface, and will be
168 * removed from {@code Cache} in Guava release 12.0. Note that
169 * {@link CacheBuilder#build(CacheLoader)} now returns a {@code LoadingCache}, so this deprecation
170 * (migration) can be dealt with by simply changing the type of references to the results of
171 * {@link CacheBuilder#build(CacheLoader)}.
172 */
173 @Deprecated V getUnchecked(K key);
174
175 /**
176 * Discouraged. Provided to satisfy the {@code Function} interface; use {@link #get} or
177 * {@link #getUnchecked} instead.
178 *
179 * @throws UncheckedExecutionException if an exception was thrown while loading the value,
180 * regardless of whether the exception was checked or unchecked
181 * @throws ExecutionError if an error was thrown while loading the value
182 * @deprecated This method has been split out into the {@link LoadingCache} interface, and will be
183 * removed from {@code Cache} in Guava release 12.0. Note that
184 * {@link CacheBuilder#build(CacheLoader)} now returns a {@code LoadingCache}, so this deprecation
185 * (migration) can be dealt with by simply changing the type of references to the results of
186 * {@link CacheBuilder#build(CacheLoader)}.
187 */
188 @Deprecated V apply(K key);
189 }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy