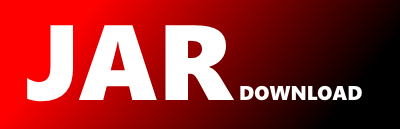
javadoc.src-html.com.google.common.hash.Hasher.html Maven / Gradle / Ivy
The newest version!
001 /*
002 * Copyright (C) 2011 The Guava Authors
003 *
004 * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
005 * in compliance with the License. You may obtain a copy of the License at
006 *
007 * http://www.apache.org/licenses/LICENSE-2.0
008 *
009 * Unless required by applicable law or agreed to in writing, software distributed under the License
010 * is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
011 * or implied. See the License for the specific language governing permissions and limitations under
012 * the License.
013 */
014
015 package com.google.common.hash;
016
017 import com.google.common.annotations.Beta;
018
019 import java.nio.charset.Charset;
020
021 /**
022 * A {@link Sink} that can compute a hash code after reading the input. Each hasher should
023 * translate all multibyte values ({@link #putInt(int)}, {@link #putLong(long)}, etc) to bytes
024 * in little-endian order.
025 *
026 * @author Kevin Bourrillion
027 * @since 11.0
028 */
029 @Beta
030 public interface Hasher extends Sink {
031 @Override Hasher putByte(byte b);
032 @Override Hasher putBytes(byte[] bytes);
033 @Override Hasher putBytes(byte[] bytes, int off, int len);
034 @Override Hasher putShort(short s);
035 @Override Hasher putInt(int i);
036 @Override Hasher putLong(long l);
037 /**
038 * Equivalent to {@code putInt(Float.floatToRawIntBits(f))}.
039 */
040 @Override Hasher putFloat(float f);
041 /**
042 * Equivalent to {@code putLong(Double.doubleToRawLongBits(d))}.
043 */
044 @Override Hasher putDouble(double d);
045 /**
046 * Equivalent to {@code putByte(b ? (byte) 1 : (byte) 0)}.
047 */
048 @Override Hasher putBoolean(boolean b);
049 @Override Hasher putChar(char c);
050 /**
051 * Equivalent to {@code putBytes(charSequence.toString().getBytes(Charsets.UTF_16LE)}.
052 */
053 @Override Hasher putString(CharSequence charSequence);
054 /**
055 * Equivalent to {@code putBytes(charSequence.toString().getBytes(charset)}.
056 */
057 @Override Hasher putString(CharSequence charSequence, Charset charset);
058
059 /**
060 * A simple convenience for {@code funnel.funnel(object, this)}.
061 */
062 <T> Hasher putObject(T instance, Funnel<? super T> funnel);
063
064 /**
065 * Computes a hash code based on the data that have been provided to this hasher. The result is
066 * unspecified if this method is called more than once on the same instance.
067 */
068 HashCode hash();
069 }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy