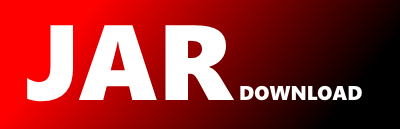
javadoc.src-html.com.google.common.io.LimitInputStream.html Maven / Gradle / Ivy
The newest version!
001 /*
002 * Copyright (C) 2007 The Guava Authors
003 *
004 * Licensed under the Apache License, Version 2.0 (the "License");
005 * you may not use this file except in compliance with the License.
006 * You may obtain a copy of the License at
007 *
008 * http://www.apache.org/licenses/LICENSE-2.0
009 *
010 * Unless required by applicable law or agreed to in writing, software
011 * distributed under the License is distributed on an "AS IS" BASIS,
012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
013 * See the License for the specific language governing permissions and
014 * limitations under the License.
015 */
016
017 package com.google.common.io;
018
019 import com.google.common.annotations.Beta;
020 import com.google.common.base.Preconditions;
021
022 import java.io.FilterInputStream;
023 import java.io.IOException;
024 import java.io.InputStream;
025
026 /**
027 * An InputStream that limits the number of bytes which can be read.
028 *
029 * @author Charles Fry
030 * @since 1.0
031 */
032 @Beta
033 public final class LimitInputStream extends FilterInputStream {
034
035 private long left;
036 private long mark = -1;
037
038 /**
039 * Wraps another input stream, limiting the number of bytes which can be read.
040 *
041 * @param in the input stream to be wrapped
042 * @param limit the maximum number of bytes to be read
043 */
044 public LimitInputStream(InputStream in, long limit) {
045 super(in);
046 Preconditions.checkNotNull(in);
047 Preconditions.checkArgument(limit >= 0, "limit must be non-negative");
048 left = limit;
049 }
050
051 @Override public int available() throws IOException {
052 return (int) Math.min(in.available(), left);
053 }
054
055 @Override public synchronized void mark(int readlimit) {
056 in.mark(readlimit);
057 mark = left;
058 // it's okay to mark even if mark isn't supported, as reset won't work
059 }
060
061 @Override public int read() throws IOException {
062 if (left == 0) {
063 return -1;
064 }
065
066 int result = in.read();
067 if (result != -1) {
068 --left;
069 }
070 return result;
071 }
072
073 @Override public int read(byte[] b, int off, int len) throws IOException {
074 if (left == 0) {
075 return -1;
076 }
077
078 len = (int) Math.min(len, left);
079 int result = in.read(b, off, len);
080 if (result != -1) {
081 left -= result;
082 }
083 return result;
084 }
085
086 @Override public synchronized void reset() throws IOException {
087 if (!in.markSupported()) {
088 throw new IOException("Mark not supported");
089 }
090 if (mark == -1) {
091 throw new IOException("Mark not set");
092 }
093
094 in.reset();
095 left = mark;
096 }
097
098 @Override public long skip(long n) throws IOException {
099 n = Math.min(n, left);
100 long skipped = in.skip(n);
101 left -= skipped;
102 return skipped;
103 }
104 }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy