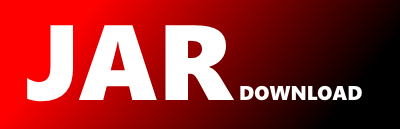
javadoc.src-html.com.google.common.primitives.Chars.html Maven / Gradle / Ivy
The newest version!
001 /*
002 * Copyright (C) 2008 The Guava Authors
003 *
004 * Licensed under the Apache License, Version 2.0 (the "License");
005 * you may not use this file except in compliance with the License.
006 * You may obtain a copy of the License at
007 *
008 * http://www.apache.org/licenses/LICENSE-2.0
009 *
010 * Unless required by applicable law or agreed to in writing, software
011 * distributed under the License is distributed on an "AS IS" BASIS,
012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
013 * See the License for the specific language governing permissions and
014 * limitations under the License.
015 */
016
017 package com.google.common.primitives;
018
019 import static com.google.common.base.Preconditions.checkArgument;
020 import static com.google.common.base.Preconditions.checkElementIndex;
021 import static com.google.common.base.Preconditions.checkNotNull;
022 import static com.google.common.base.Preconditions.checkPositionIndexes;
023
024 import com.google.common.annotations.GwtCompatible;
025 import com.google.common.annotations.GwtIncompatible;
026
027 import java.io.Serializable;
028 import java.util.AbstractList;
029 import java.util.Arrays;
030 import java.util.Collection;
031 import java.util.Collections;
032 import java.util.Comparator;
033 import java.util.List;
034 import java.util.RandomAccess;
035
036 /**
037 * Static utility methods pertaining to {@code char} primitives, that are not
038 * already found in either {@link Character} or {@link Arrays}.
039 *
040 * <p>All the operations in this class treat {@code char} values strictly
041 * numerically; they are neither Unicode-aware nor locale-dependent.
042 *
043 * @author Kevin Bourrillion
044 * @since 1.0
045 */
046 @GwtCompatible(emulated = true)
047 public final class Chars {
048 private Chars() {}
049
050 /**
051 * The number of bytes required to represent a primitive {@code char}
052 * value.
053 */
054 public static final int BYTES = Character.SIZE / Byte.SIZE;
055
056 /**
057 * Returns a hash code for {@code value}; equal to the result of invoking
058 * {@code ((Character) value).hashCode()}.
059 *
060 * @param value a primitive {@code char} value
061 * @return a hash code for the value
062 */
063 public static int hashCode(char value) {
064 return value;
065 }
066
067 /**
068 * Returns the {@code char} value that is equal to {@code value}, if possible.
069 *
070 * @param value any value in the range of the {@code char} type
071 * @return the {@code char} value that equals {@code value}
072 * @throws IllegalArgumentException if {@code value} is greater than {@link
073 * Character#MAX_VALUE} or less than {@link Character#MIN_VALUE}
074 */
075 public static char checkedCast(long value) {
076 char result = (char) value;
077 checkArgument(result == value, "Out of range: %s", value);
078 return result;
079 }
080
081 /**
082 * Returns the {@code char} nearest in value to {@code value}.
083 *
084 * @param value any {@code long} value
085 * @return the same value cast to {@code char} if it is in the range of the
086 * {@code char} type, {@link Character#MAX_VALUE} if it is too large,
087 * or {@link Character#MIN_VALUE} if it is too small
088 */
089 public static char saturatedCast(long value) {
090 if (value > Character.MAX_VALUE) {
091 return Character.MAX_VALUE;
092 }
093 if (value < Character.MIN_VALUE) {
094 return Character.MIN_VALUE;
095 }
096 return (char) value;
097 }
098
099 /**
100 * Compares the two specified {@code char} values. The sign of the value
101 * returned is the same as that of {@code ((Character) a).compareTo(b)}.
102 *
103 * @param a the first {@code char} to compare
104 * @param b the second {@code char} to compare
105 * @return a negative value if {@code a} is less than {@code b}; a positive
106 * value if {@code a} is greater than {@code b}; or zero if they are equal
107 */
108 public static int compare(char a, char b) {
109 return a - b; // safe due to restricted range
110 }
111
112 /**
113 * Returns {@code true} if {@code target} is present as an element anywhere in
114 * {@code array}.
115 *
116 * @param array an array of {@code char} values, possibly empty
117 * @param target a primitive {@code char} value
118 * @return {@code true} if {@code array[i] == target} for some value of {@code
119 * i}
120 */
121 public static boolean contains(char[] array, char target) {
122 for (char value : array) {
123 if (value == target) {
124 return true;
125 }
126 }
127 return false;
128 }
129
130 /**
131 * Returns the index of the first appearance of the value {@code target} in
132 * {@code array}.
133 *
134 * @param array an array of {@code char} values, possibly empty
135 * @param target a primitive {@code char} value
136 * @return the least index {@code i} for which {@code array[i] == target}, or
137 * {@code -1} if no such index exists.
138 */
139 public static int indexOf(char[] array, char target) {
140 return indexOf(array, target, 0, array.length);
141 }
142
143 // TODO(kevinb): consider making this public
144 private static int indexOf(
145 char[] array, char target, int start, int end) {
146 for (int i = start; i < end; i++) {
147 if (array[i] == target) {
148 return i;
149 }
150 }
151 return -1;
152 }
153
154 /**
155 * Returns the start position of the first occurrence of the specified {@code
156 * target} within {@code array}, or {@code -1} if there is no such occurrence.
157 *
158 * <p>More formally, returns the lowest index {@code i} such that {@code
159 * java.util.Arrays.copyOfRange(array, i, i + target.length)} contains exactly
160 * the same elements as {@code target}.
161 *
162 * @param array the array to search for the sequence {@code target}
163 * @param target the array to search for as a sub-sequence of {@code array}
164 */
165 public static int indexOf(char[] array, char[] target) {
166 checkNotNull(array, "array");
167 checkNotNull(target, "target");
168 if (target.length == 0) {
169 return 0;
170 }
171
172 outer:
173 for (int i = 0; i < array.length - target.length + 1; i++) {
174 for (int j = 0; j < target.length; j++) {
175 if (array[i + j] != target[j]) {
176 continue outer;
177 }
178 }
179 return i;
180 }
181 return -1;
182 }
183
184 /**
185 * Returns the index of the last appearance of the value {@code target} in
186 * {@code array}.
187 *
188 * @param array an array of {@code char} values, possibly empty
189 * @param target a primitive {@code char} value
190 * @return the greatest index {@code i} for which {@code array[i] == target},
191 * or {@code -1} if no such index exists.
192 */
193 public static int lastIndexOf(char[] array, char target) {
194 return lastIndexOf(array, target, 0, array.length);
195 }
196
197 // TODO(kevinb): consider making this public
198 private static int lastIndexOf(
199 char[] array, char target, int start, int end) {
200 for (int i = end - 1; i >= start; i--) {
201 if (array[i] == target) {
202 return i;
203 }
204 }
205 return -1;
206 }
207
208 /**
209 * Returns the least value present in {@code array}.
210 *
211 * @param array a <i>nonempty</i> array of {@code char} values
212 * @return the value present in {@code array} that is less than or equal to
213 * every other value in the array
214 * @throws IllegalArgumentException if {@code array} is empty
215 */
216 public static char min(char... array) {
217 checkArgument(array.length > 0);
218 char min = array[0];
219 for (int i = 1; i < array.length; i++) {
220 if (array[i] < min) {
221 min = array[i];
222 }
223 }
224 return min;
225 }
226
227 /**
228 * Returns the greatest value present in {@code array}.
229 *
230 * @param array a <i>nonempty</i> array of {@code char} values
231 * @return the value present in {@code array} that is greater than or equal to
232 * every other value in the array
233 * @throws IllegalArgumentException if {@code array} is empty
234 */
235 public static char max(char... array) {
236 checkArgument(array.length > 0);
237 char max = array[0];
238 for (int i = 1; i < array.length; i++) {
239 if (array[i] > max) {
240 max = array[i];
241 }
242 }
243 return max;
244 }
245
246 /**
247 * Returns the values from each provided array combined into a single array.
248 * For example, {@code concat(new char[] {a, b}, new char[] {}, new
249 * char[] {c}} returns the array {@code {a, b, c}}.
250 *
251 * @param arrays zero or more {@code char} arrays
252 * @return a single array containing all the values from the source arrays, in
253 * order
254 */
255 public static char[] concat(char[]... arrays) {
256 int length = 0;
257 for (char[] array : arrays) {
258 length += array.length;
259 }
260 char[] result = new char[length];
261 int pos = 0;
262 for (char[] array : arrays) {
263 System.arraycopy(array, 0, result, pos, array.length);
264 pos += array.length;
265 }
266 return result;
267 }
268
269 /**
270 * Returns a big-endian representation of {@code value} in a 2-element byte
271 * array; equivalent to {@code
272 * ByteBuffer.allocate(2).putChar(value).array()}. For example, the input
273 * value {@code '\\u5432'} would yield the byte array {@code {0x54, 0x32}}.
274 *
275 * <p>If you need to convert and concatenate several values (possibly even of
276 * different types), use a shared {@link java.nio.ByteBuffer} instance, or use
277 * {@link com.google.common.io.ByteStreams#newDataOutput()} to get a growable
278 * buffer.
279 */
280 @GwtIncompatible("doesn't work")
281 public static byte[] toByteArray(char value) {
282 return new byte[] {
283 (byte) (value >> 8),
284 (byte) value};
285 }
286
287 /**
288 * Returns the {@code char} value whose big-endian representation is
289 * stored in the first 2 bytes of {@code bytes}; equivalent to {@code
290 * ByteBuffer.wrap(bytes).getChar()}. For example, the input byte array
291 * {@code {0x54, 0x32}} would yield the {@code char} value {@code '\\u5432'}.
292 *
293 * <p>Arguably, it's preferable to use {@link java.nio.ByteBuffer}; that
294 * library exposes much more flexibility at little cost in readability.
295 *
296 * @throws IllegalArgumentException if {@code bytes} has fewer than 2
297 * elements
298 */
299 @GwtIncompatible("doesn't work")
300 public static char fromByteArray(byte[] bytes) {
301 checkArgument(bytes.length >= BYTES,
302 "array too small: %s < %s", bytes.length, BYTES);
303 return fromBytes(bytes[0], bytes[1]);
304 }
305
306 /**
307 * Returns the {@code char} value whose byte representation is the given 2
308 * bytes, in big-endian order; equivalent to {@code Chars.fromByteArray(new
309 * byte[] {b1, b2})}.
310 *
311 * @since 7.0
312 */
313 @GwtIncompatible("doesn't work")
314 public static char fromBytes(byte b1, byte b2) {
315 return (char) ((b1 << 8) | (b2 & 0xFF));
316 }
317
318 /**
319 * Returns an array containing the same values as {@code array}, but
320 * guaranteed to be of a specified minimum length. If {@code array} already
321 * has a length of at least {@code minLength}, it is returned directly.
322 * Otherwise, a new array of size {@code minLength + padding} is returned,
323 * containing the values of {@code array}, and zeroes in the remaining places.
324 *
325 * @param array the source array
326 * @param minLength the minimum length the returned array must guarantee
327 * @param padding an extra amount to "grow" the array by if growth is
328 * necessary
329 * @throws IllegalArgumentException if {@code minLength} or {@code padding} is
330 * negative
331 * @return an array containing the values of {@code array}, with guaranteed
332 * minimum length {@code minLength}
333 */
334 public static char[] ensureCapacity(
335 char[] array, int minLength, int padding) {
336 checkArgument(minLength >= 0, "Invalid minLength: %s", minLength);
337 checkArgument(padding >= 0, "Invalid padding: %s", padding);
338 return (array.length < minLength)
339 ? copyOf(array, minLength + padding)
340 : array;
341 }
342
343 // Arrays.copyOf() requires Java 6
344 private static char[] copyOf(char[] original, int length) {
345 char[] copy = new char[length];
346 System.arraycopy(original, 0, copy, 0, Math.min(original.length, length));
347 return copy;
348 }
349
350 /**
351 * Returns a string containing the supplied {@code char} values separated
352 * by {@code separator}. For example, {@code join("-", '1', '2', '3')} returns
353 * the string {@code "1-2-3"}.
354 *
355 * @param separator the text that should appear between consecutive values in
356 * the resulting string (but not at the start or end)
357 * @param array an array of {@code char} values, possibly empty
358 */
359 public static String join(String separator, char... array) {
360 checkNotNull(separator);
361 int len = array.length;
362 if (len == 0) {
363 return "";
364 }
365
366 StringBuilder builder
367 = new StringBuilder(len + separator.length() * (len - 1));
368 builder.append(array[0]);
369 for (int i = 1; i < len; i++) {
370 builder.append(separator).append(array[i]);
371 }
372 return builder.toString();
373 }
374
375 /**
376 * Returns a comparator that compares two {@code char} arrays
377 * lexicographically. That is, it compares, using {@link
378 * #compare(char, char)}), the first pair of values that follow any
379 * common prefix, or when one array is a prefix of the other, treats the
380 * shorter array as the lesser. For example,
381 * {@code [] < ['a'] < ['a', 'b'] < ['b']}.
382 *
383 * <p>The returned comparator is inconsistent with {@link
384 * Object#equals(Object)} (since arrays support only identity equality), but
385 * it is consistent with {@link Arrays#equals(char[], char[])}.
386 *
387 * @see <a href="http://en.wikipedia.org/wiki/Lexicographical_order">
388 * Lexicographical order article at Wikipedia</a>
389 * @since 2.0
390 */
391 public static Comparator<char[]> lexicographicalComparator() {
392 return LexicographicalComparator.INSTANCE;
393 }
394
395 private enum LexicographicalComparator implements Comparator<char[]> {
396 INSTANCE;
397
398 @Override
399 public int compare(char[] left, char[] right) {
400 int minLength = Math.min(left.length, right.length);
401 for (int i = 0; i < minLength; i++) {
402 int result = Chars.compare(left[i], right[i]);
403 if (result != 0) {
404 return result;
405 }
406 }
407 return left.length - right.length;
408 }
409 }
410
411 /**
412 * Copies a collection of {@code Character} instances into a new array of
413 * primitive {@code char} values.
414 *
415 * <p>Elements are copied from the argument collection as if by {@code
416 * collection.toArray()}. Calling this method is as thread-safe as calling
417 * that method.
418 *
419 * @param collection a collection of {@code Character} objects
420 * @return an array containing the same values as {@code collection}, in the
421 * same order, converted to primitives
422 * @throws NullPointerException if {@code collection} or any of its elements
423 * is null
424 */
425 public static char[] toArray(Collection<Character> collection) {
426 if (collection instanceof CharArrayAsList) {
427 return ((CharArrayAsList) collection).toCharArray();
428 }
429
430 Object[] boxedArray = collection.toArray();
431 int len = boxedArray.length;
432 char[] array = new char[len];
433 for (int i = 0; i < len; i++) {
434 // checkNotNull for GWT (do not optimize)
435 array[i] = (Character) checkNotNull(boxedArray[i]);
436 }
437 return array;
438 }
439
440 /**
441 * Returns a fixed-size list backed by the specified array, similar to {@link
442 * Arrays#asList(Object[])}. The list supports {@link List#set(int, Object)},
443 * but any attempt to set a value to {@code null} will result in a {@link
444 * NullPointerException}.
445 *
446 * <p>The returned list maintains the values, but not the identities, of
447 * {@code Character} objects written to or read from it. For example, whether
448 * {@code list.get(0) == list.get(0)} is true for the returned list is
449 * unspecified.
450 *
451 * @param backingArray the array to back the list
452 * @return a list view of the array
453 */
454 public static List<Character> asList(char... backingArray) {
455 if (backingArray.length == 0) {
456 return Collections.emptyList();
457 }
458 return new CharArrayAsList(backingArray);
459 }
460
461 @GwtCompatible
462 private static class CharArrayAsList extends AbstractList<Character>
463 implements RandomAccess, Serializable {
464 final char[] array;
465 final int start;
466 final int end;
467
468 CharArrayAsList(char[] array) {
469 this(array, 0, array.length);
470 }
471
472 CharArrayAsList(char[] array, int start, int end) {
473 this.array = array;
474 this.start = start;
475 this.end = end;
476 }
477
478 @Override public int size() {
479 return end - start;
480 }
481
482 @Override public boolean isEmpty() {
483 return false;
484 }
485
486 @Override public Character get(int index) {
487 checkElementIndex(index, size());
488 return array[start + index];
489 }
490
491 @Override public boolean contains(Object target) {
492 // Overridden to prevent a ton of boxing
493 return (target instanceof Character)
494 && Chars.indexOf(array, (Character) target, start, end) != -1;
495 }
496
497 @Override public int indexOf(Object target) {
498 // Overridden to prevent a ton of boxing
499 if (target instanceof Character) {
500 int i = Chars.indexOf(array, (Character) target, start, end);
501 if (i >= 0) {
502 return i - start;
503 }
504 }
505 return -1;
506 }
507
508 @Override public int lastIndexOf(Object target) {
509 // Overridden to prevent a ton of boxing
510 if (target instanceof Character) {
511 int i = Chars.lastIndexOf(array, (Character) target, start, end);
512 if (i >= 0) {
513 return i - start;
514 }
515 }
516 return -1;
517 }
518
519 @Override public Character set(int index, Character element) {
520 checkElementIndex(index, size());
521 char oldValue = array[start + index];
522 array[start + index] = checkNotNull(element); // checkNotNull for GWT (do not optimize)
523 return oldValue;
524 }
525
526 @Override public List<Character> subList(int fromIndex, int toIndex) {
527 int size = size();
528 checkPositionIndexes(fromIndex, toIndex, size);
529 if (fromIndex == toIndex) {
530 return Collections.emptyList();
531 }
532 return new CharArrayAsList(array, start + fromIndex, start + toIndex);
533 }
534
535 @Override public boolean equals(Object object) {
536 if (object == this) {
537 return true;
538 }
539 if (object instanceof CharArrayAsList) {
540 CharArrayAsList that = (CharArrayAsList) object;
541 int size = size();
542 if (that.size() != size) {
543 return false;
544 }
545 for (int i = 0; i < size; i++) {
546 if (array[start + i] != that.array[that.start + i]) {
547 return false;
548 }
549 }
550 return true;
551 }
552 return super.equals(object);
553 }
554
555 @Override public int hashCode() {
556 int result = 1;
557 for (int i = start; i < end; i++) {
558 result = 31 * result + Chars.hashCode(array[i]);
559 }
560 return result;
561 }
562
563 @Override public String toString() {
564 StringBuilder builder = new StringBuilder(size() * 3);
565 builder.append('[').append(array[start]);
566 for (int i = start + 1; i < end; i++) {
567 builder.append(", ").append(array[i]);
568 }
569 return builder.append(']').toString();
570 }
571
572 char[] toCharArray() {
573 // Arrays.copyOfRange() requires Java 6
574 int size = size();
575 char[] result = new char[size];
576 System.arraycopy(array, start, result, 0, size);
577 return result;
578 }
579
580 private static final long serialVersionUID = 0;
581 }
582 }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy