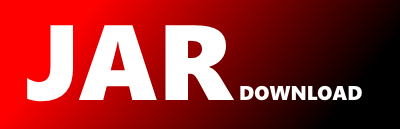
org.jace.util.DelimitedCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jace-core-java Show documentation
Show all versions of jace-core-java Show documentation
Jace core module, Java source-code
package org.jace.util;
import java.util.Collection;
import java.util.Iterator;
/**
* A utility class that returns the String representation of a collection.
*
* @param the list type
* @author Toby Reyelts
*/
public class DelimitedCollection
{
private final Collection collection;
/**
* Returns the String representation of an Object.
*
* @param the object type
* @author Toby Reyelts
*/
@SuppressWarnings("PublicInnerClass")
public interface Stringifier
{
/**
* Returns the String representation of the object.
*
* @param t the object
* @return the String representation
*/
public String toString(T t);
}
/**
* Returns Object.toString().
*
* @author Toby Reyelts
*/
private static Stringifier
© 2015 - 2024 Weber Informatics LLC | Privacy Policy