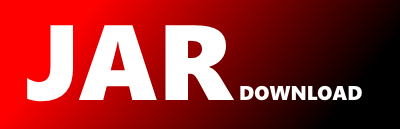
jaitools.CollectionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt-all Show documentation
Show all versions of jt-all Show documentation
Provides a single jar containing all JAI-tools modules which you can
use instead of including individual modules in your project. Note:
It does not include the Jiffle scripting language or Jiffle image
operator.
The newest version!
/*
* Copyright 2009-2011 Michael Bedward
*
* This file is part of jai-tools.
*
* jai-tools is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* jai-tools is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with jai-tools. If not, see .
*
*/
package jaitools;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.Stack;
import java.util.TreeMap;
import java.util.TreeSet;
/**
* Convenience methods to create generic collections
* following the DRY (Don't Repeat Yourself) principle.
* Examples of use:
*
* // simple
* List<Integer> list1 = CollectionFactory.list();
*
* // nested
* List<List<String>> list2 = CollectionFactory.list();
*
* // a multi-map
* Map<String, List<Integer>> multiMap = CollectionFactory.map();
*
*
* @author Michael Bedward
* @since 1.0
* @version $Id: CollectionFactory.java 1504 2011-03-05 10:56:10Z michael.bedward $
*/
public class CollectionFactory {
/**
* Returns a new {@code Map} object. The returned
* object is a {@link HashMap}.
*
* Example of use:
*
* Map<Foo, Bar> myMap = CollectionFactory.map();
*
*
* @param key type
* @param value type
* @return a new Map<K,V> instance
*/
public static Map map() {
return new HashMap();
}
/**
* Returns a new {@code Map} object that maintains insertion
* order. The returned object is a {@link LinkedHashMap}.
*
* Example of use:
*
* Map<Foo, Bar> myMap = CollectionFactory.orderedMap();
*
*
* @param key type
* @param value type
* @return a new Map<K,V> instance
*/
public static Map orderedMap() {
return new LinkedHashMap();
}
/**
* Returns a new {@code SortedMap} object. Key type {@code K} must
* implement {@linkplain Comparable}. The returned object is a
* {@link TreeMap}.
*
* Example of use:
*
* Map<Foo, Bar> myMap = CollectionFactory.sortedMap();
*
*
* @param key type
* @param value type
* @return a new SortedMap<K,V> instance
*/
public static SortedMap sortedMap() {
return new TreeMap();
}
/**
* Returns a new {@code List} object. The returned object is
* an {@link ArrayList}.
*
* Example of use:
*
* List<Foo> myList = CollectionFactory.list();
*
*
* @param element type
* @return a new List<T> instance
*/
public static List list() {
return new ArrayList();
}
/**
* Returns a new {@code Stack} object.
*
* Example of use:
*
* Stack<Foo> myStack = CollectionFactory.stack();
*
*
* @param element type
* @return a new Stack<T> instance
*/
public static Stack stack() {
return new Stack();
}
/**
* Returns a new {@code Set} instance. The returned object is
* a {@link HashSet}.
*
* Example of use:
*
* Set<Foo> mySet = CollectionFactory.set();
*
*
* @param element type
* @return a new HashSet<T> instance
*/
public static Set set() {
return new HashSet();
}
/**
* Returns a new {@code Set} instance that maintains
* the insertion order of its elements.
*
* Example of use:
*
* Set<Foo> mySet = CollectionFactory.set();
*
*
* @param element type
* @return a new LinkedHashSet<T> instance
*/
public static Set orderedSet() {
return new LinkedHashSet();
}
/**
* Returns a new {@code SortedSet} instance. The type {@code T}
* must implement {@linkplain Comparable}. The returned object
* is a {@link TreeSet}.
*
* Example of use:
*
* Set<MyObject> foo = CollectionFactory.sortedSet();
*
*
* @param element type
* @return a new TreeSet<T> instance
*/
public static SortedSet sortedSet() {
return new TreeSet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy