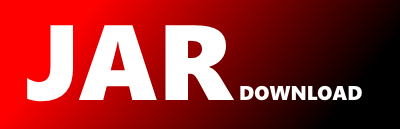
jaitools.numeric.DoubleComparison Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt-all Show documentation
Show all versions of jt-all Show documentation
Provides a single jar containing all JAI-tools modules which you can
use instead of including individual modules in your project. Note:
It does not include the Jiffle scripting language or Jiffle image
operator.
The newest version!
/*
* Copyright 2009 Michael Bedward
*
* This file is part of jai-tools.
* jai-tools is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
* jai-tools is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public
* License along with jai-tools. If not, see .
*
*/
package jaitools.numeric;
/**
* Provides static method to compare double values with either a default or
* specified tolerance.
*
* @deprecated This class will be removed in JAI-tools version 1.2. Please
* use {@linkplain CompareOp} instead.
*
* @author Michael Bedward
* @since 1.0
* @version $Id: DoubleComparison.java 1610 2011-03-31 04:44:28Z michael.bedward $
*/
public class DoubleComparison {
/** Default tolerance for comparisons: 1.0e-8 */
public static final double TOL = 1.0e-8;
/**
* Tests if the given value is 0 within the default tolerance.
* @param x the value
* @return {@code true} if zero; {@code false} otherwise
*/
public static boolean dzero(double x) {
return Math.abs(x) < TOL;
}
/**
* Tests if the given value is 0 within the user-specified tolerance.
* @param x the value
* @param tol the user-specified tolerance (assumed to be positive)
* @return {@code true} if zero; {@code false} otherwise
*/
public static boolean dzero(double x, double tol) {
if (tol < TOL) {
return dzero(x);
}
return Math.abs(x) < tol;
}
/**
* Compares two values using the default tolerance.
* @param x1 first value
* @param x2 second value
* @return a value less than 0 if x1 is less than x2; 0 if x1 is equal to x2;
* a value greater than 0 if x1 is greater than x2
*/
public static int dcomp(double x1, double x2) {
if (dzero(x1 - x2)) {
return 0;
} else {
return Double.compare(x1, x2);
}
}
/**
* Compares two values using the user-specified tolerance
* @param x1 first value
* @param x2 second value
* @param tol the tolerance
* @return a value less than 0 if x1 is less than x2; 0 if x1 is equal to x2;
* a value greater than 0 if x1 is greater than x2
*/
public static int dcomp(double x1, double x2, double tol) {
if (tol < TOL) {
return dcomp(x1, x2);
}
if (dzero(x1 - x2, tol)) {
return 0;
} else {
return Double.compare(x1, x2);
}
}
/**
* Tests if two double values are equal within the default tolerance.
* This is a short-cut for {@code dzero(x1 - x2)}.
* @param x1 first value
* @param x2 second value
* @return {@code true} if equal; {@code false} otherwise
*/
public static boolean dequal(double x1, double x2) {
return dzero(x1 - x2);
}
/**
* Tests if two double values are equal within the specified tolerance.
* This is a short-cut for {@code dzero(x1 - x2, tol)}.
* @param x1 first value
* @param x2 second value
* @param tol the tolerance
* @return {@code true} if equal; {@code false} otherwise
*/
public static boolean dequal(double x1, double x2, double tol) {
return dzero(x1 - x2, tol);
}
/**
* Converts a double value to integer taking into account the
* default tolerance when checking if it is zero, ie. values
* within TOL either side of 0 will produce a 0 return value
*
* @param x the value to convert
* @return the equivalent integer value
*/
public static int toInt(double x) {
int sign = dcomp(x, 0d);
if (sign > 0) { // +ve value
return (int)(x + 0.5);
} else if (sign < 0) { // -ve value
return (int)(x - 0.5);
} else {
return 0;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy