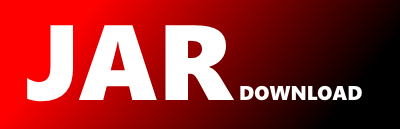
jaitools.tilecache.BasicCacheVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jt-all Show documentation
Show all versions of jt-all Show documentation
Provides a single jar containing all JAI-tools modules which you can
use instead of including individual modules in your project. Note:
It does not include the Jiffle scripting language or Jiffle image
operator.
The newest version!
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package jaitools.tilecache;
import java.awt.image.RenderedImage;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
/**
* A basic visitor class for DiskMemTileCache
that can be used
* to examine cache contents.
*
* Example of use:
*
* // Query the cache for tiles that belong to a given image
* // and that are currently resident in memory
* DiskMemTileCache cache = ...
* RenderedImage owner = ...
*
* Map filters = CollectionFactory.newMap();
* filters.put(BasicCacheVisitor.Key.OWNER, owner);
* filters.put(BasicCachevisitor.Key.RESIDENT, Boolean.TRUE);
*
* BasicCacheVisitor visitor = new BasicCacheVisitor();
* visitor.setFilters(filters);
* cache.accept(visitor);
*
* for (DiskCachedTile tile : visitor.getTiles()) {
* System.out.println(String.format("tile %d,%d", tile.getTileX(), tile.getTileY()));
* }
*
*
* @author Michael Bedward
* @since 1.0
* @version $Id: BasicCacheVisitor.java 1383 2011-02-10 11:22:29Z michael.bedward $
*/
public class BasicCacheVisitor implements DiskMemTileCacheVisitor {
/**
* Defines keys to filter the tles visited
*/
public enum Key {
/**
* Filter on the image that owns the tile. The value
* provided for this key must be a RenderedImage.
*/
OWNER(RenderedImage.class),
/**
* Filter on whether the tile is currently resident
* in memory. The value provided for this key must
* be of type Boolean.
*/
RESIDENT(Boolean.class);
private Class> clazz;
private Key(Class> clazz) {
this.clazz = clazz;
}
public Class> getValueClass() {
return clazz;
}
}
private List tiles = new ArrayList();
private Map filters = new HashMap();
/**
* Set filter conditions. Any existing conditions are cleared.
*
* @param params a Map
where each key is one of the constants
* defined by BasicCacheVisitor.Key
and each value is the
* corresponding value to match tiles against
*/
public void setFilters(Map params) {
filters.clear();
for (Entry e : params.entrySet()) {
addFilter(e.getKey(), e.getValue());
}
}
/**
* Set a single filter condition. Any existing conditions
* are cleared
*
* @param key one of the constants defined by BasicCacheVisitor.Key
* @param value corresponding value to match tiles against
*/
public void setFilter(Key key, Object value) {
filters.clear();
addFilter(key, value);
}
/**
* Clear all existing filter conditions
*/
public void clearFilters() {
filters.clear();
}
/**
* Returns an unmodifiable collection of tiles retrieved from
* the cache.
*
* @return tiles retrieved from the cache
*/
public Collection getTiles() {
return Collections.unmodifiableCollection(tiles);
}
/**
* This method is called by the cache for each tile in turn. Tiles that
* pass this visitor's filter conditions, if any, will be added to the
* visitor's tile collection.
*
* @param tile the cached tile being visited
* @param isResident set by the cache to indicate whether the tile is
* currently resident in memory
*/
public void visit(DiskCachedTile tile, boolean isResident) {
if (filters.isEmpty()) {
tiles.add(tile);
} else {
boolean pass = true;
if (filters.containsKey(Key.OWNER)) {
if ( !(tile.getOwner().equals(filters.get(Key.OWNER))) ) {
pass = false;
}
}
if (pass && filters.containsKey(Key.RESIDENT)) {
if ( ((Boolean)filters.get(Key.RESIDENT)).booleanValue() != isResident) {
pass = false;
}
}
if (pass) {
tiles.add(tile);
}
}
}
/**
* Clear collected tile data. Useful if an instance of this
* class makes multiple visits to a cache.
*/
public void clear() {
tiles.clear();
}
/**
* Helper method for setFilters and setFilter
* @param key
* @param value
*/
private void addFilter(Key key, Object value) {
if (key.getValueClass().isAssignableFrom(value.getClass())) {
filters.put(key, value);
} else {
throw new IllegalArgumentException(
String.format("Object of type %s cannot be used as value for %s",
value.getClass().getName(), key.toString()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy