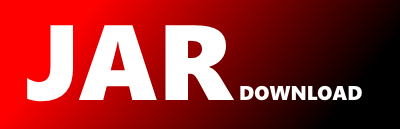
jasima.core.experiment.AbstractMultiExperiment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jasima-main Show documentation
Show all versions of jasima-main Show documentation
JAva SImulatior for MAnufacturing and logistics - A framework for discrete event simulation and computer experiments with a main focus on modelling and analyzing logistic/manufacturing systems.
/*******************************************************************************
* Copyright (c) 2010-2013 Torsten Hildebrandt and jasima contributors
*
* This file is part of jasima, v1.0.
*
* jasima is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* jasima is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with jasima. If not, see .
*
* $Id: AbstractMultiExperiment.java 74 2013-01-08 17:31:49Z [email protected] $
*******************************************************************************/
package jasima.core.experiment;
import jasima.core.expExecution.ExperimentExecutor;
import jasima.core.expExecution.ExperimentFuture;
import jasima.core.statistics.SummaryStat;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Random;
/**
* Parent class of an experiment which runs a number of child experiments.
*
* @author Torsten Hildebrandt
* @version
* "$Id: AbstractMultiExperiment.java 74 2013-01-08 17:31:49Z [email protected] $"
*/
public abstract class AbstractMultiExperiment extends Experiment {
private static final long serialVersionUID = 4018379771127550074L;
public static final String NUM_TASKS_EXECUTED = "numTasks";
/**
* Complex event object triggered upon sub-experiment completion.
*/
public static class BaseExperimentCompleted extends ExperimentEvent {
public BaseExperimentCompleted(Experiment experimentRun,
Map results) {
super();
this.experimentRun = experimentRun;
this.results = results;
}
public final Experiment experimentRun;
public final Map results;
}
// parameters
private boolean allowParallelExecution = true;
private boolean commonRandomNumbers = true;
private int skipSeedCount = 0;
private boolean abortUponBaseExperimentAbort = false;
protected HashSet keepResults = new HashSet();
private boolean produceAveragedResults = true;
// fields used during run
protected Map detailedResults;
private Random seedStream;
protected List experiments;
private int numTasksExecuted;
@Override
public void init() {
super.init();
experiments = new ArrayList();
seedStream = null;
detailedResults = new UniqueNamesCheckingHashMap();
numTasksExecuted = 0;
for (int i = 0; i < getSkipSeedCount(); i++) {
// throw away seed
getExperimentSeed();
}
}
@Override
protected void performRun() {
do {
createExperiments();
executeExperiments();
} while (hasMoreTasks());
experiments.clear();
}
protected boolean hasMoreTasks() {
return false;
}
protected abstract void createExperiments();
protected void executeExperiments() {
try {
if (isAllowParallelExecution()) {
// start execution and store process results in the same order
// as they are stored in tasks
int n = 0;
Collection allFutures = ExperimentExecutor
.getExecutor().runAllExperiments(experiments);
Iterator it = allFutures.iterator();
while (it.hasNext()) {
ExperimentFuture f = it.next();
it.remove();
getAndStoreResults(experiments.get(n), f);
experiments.set(n, null);
n++;
// check if to abort this experiment, if so cancel all
// future tasks
if (aborted != 0) {
for (ExperimentFuture f2 : allFutures) {
f2.cancel(true);
}
break; // while
}
}
} else {
// sequential execution
ExperimentExecutor ex = ExperimentExecutor.getExecutor();
for (int i = 0; i < experiments.size(); i++) {
Experiment e = experiments.get(i);
experiments.set(i, null);
if (aborted == 0) {
ExperimentFuture future = ex.runExperiment(e);
getAndStoreResults(e, future);
} else {
break; // for i
}
}
}
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
private void getAndStoreResults(Experiment e, ExperimentFuture f)
throws InterruptedException {
Map res = f.get();
numTasksExecuted++;
storeRunResults(e, res);
if (numListener() > 0) {
fire(new BaseExperimentCompleted(e, res));
}
}
protected void configureRunExperiment(Experiment e) {
long s = getExperimentSeed();
e.setInitialSeed(s);
e.nestingLevel(nestingLevel() + 1);
String name = prefix() + padNumTasks(experiments.size() + 1);
if (e.getName() != null)
name = name + "." + e.getName();
e.setName(name);
}
protected long getExperimentSeed() {
if (isCommonRandomNumbers())
return getInitialSeed();
else {
if (seedStream == null)
seedStream = new Random(getInitialSeed());
return seedStream.nextLong();
}
}
protected void storeRunResults(Experiment e, Map r) {
Integer aborted = (Integer) r.get(Experiment.EXP_ABORTED);
if (aborted != null) {
if (aborted.intValue() > 0 && isAbortUponBaseExperimentAbort()) {
abort();
}
}
for (String key : r.keySet()) {
Object val = r.get(key);
if (shouldKeepDetails(key))
detailedResults.put(key + "." + prefix()
+ padNumTasks(getNumTasksExecuted()), r.get(key));
if (isProduceAveragedResults()) {
if ((val != null)
&& ((val instanceof SummaryStat) || ((val instanceof Number))))
handleNumericValue(key, val);
else
handleOtherValue(key, val);
}
}
}
private boolean shouldKeepDetails(String key) {
for (String s : keepResults) {
if (s.equals(key) || key.startsWith(s + '.'))
return true;
}
return false;
}
private String padNumTasks(int v) {
int l = String.valueOf(getNumExperiments()).length();
StringBuilder sb = new StringBuilder(l);
sb.append(v);
while (sb.length() < l)
sb.insert(0, '0');
return sb.toString();
}
public abstract int getNumExperiments();
public int getNumTasks() {
return experiments.size();
}
public int getNumTasksExecuted() {
return numTasksExecuted;
}
protected abstract String prefix();
public void abort() {
this.aborted++;
}
/**
* Handles arbitrary values "val" by storing them in an object array.
*/
protected void handleOtherValue(String key, Object val) {
@SuppressWarnings("unchecked")
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy