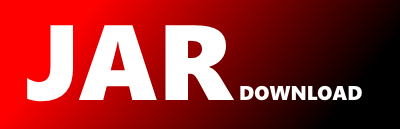
jasima.core.util.ExcelSaver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jasima-main Show documentation
Show all versions of jasima-main Show documentation
JAva SImulatior for MAnufacturing and logistics - A framework for discrete event simulation and computer experiments with a main focus on modelling and analyzing logistic/manufacturing systems.
/*******************************************************************************
* Copyright (c) 2010-2013 Torsten Hildebrandt and jasima contributors
*
* This file is part of jasima, v1.0.
*
* jasima is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* jasima is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with jasima. If not, see .
*
* $Id: ExcelSaver.java 74 2013-01-08 17:31:49Z [email protected] $
*******************************************************************************/
package jasima.core.util;
import jasima.core.experiment.Experiment;
import jasima.core.statistics.SummaryStat;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.EOFException;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import jxl.Workbook;
import jxl.WorkbookSettings;
import jxl.write.Label;
import jxl.write.NumberFormats;
import jxl.write.WritableCell;
import jxl.write.WritableCellFormat;
import jxl.write.WritableFont;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
/**
* Saves results of an experiment in a handy excel file. The data is stored in a
* file named like "runResults_2009-08-27_164340.xls". The timestamp in this
* name is the time the method saveAsExcel() was first called.
*
* This class stores final results of an experiment as well as results of any
* sub-experiments.
*
* This class supports more than 256 columns per sheet (Excel-Limit) by
* splitting data on multiple sheets.
*
* Data can be transposed when stored, i.e., rows and columns swapped.
*
* @author Torsten Hildebrandt, 2009-08-27
* @version
* "$Id: ExcelSaver.java 74 2013-01-08 17:31:49Z [email protected] $"
*/
public class ExcelSaver extends ResultSaver {
public static final String XLS_EXTENSION = ".xls";
private static final String SHEET_NAME_MAIN = "main experiment";
private static final String SHEET_NAME_OVERVIEW = "sub-exp. overview";
private static final String SHEET_NAME_MEAN = "sub-exp. value|mean";
private static final String SHEET_NAME_MIN = "sub-exp. min";
private static final String SHEET_NAME_MAX = "sub-exp. max";
private static final String SHEET_NAME_SD = "sub-exp. stdDev";
private static final String SHEET_NAME_COUNT = "sub-exp. count";
private static final String SHEET_NAME_SUM = "sub-exp. sum";
private static final String[] SUB_RES_SHEETS = { SHEET_NAME_MEAN,
SHEET_NAME_MIN, SHEET_NAME_MAX, SHEET_NAME_SD, SHEET_NAME_COUNT,
SHEET_NAME_SUM };
private static final long serialVersionUID = 342144249972918192L;
public static void main(String[] args) {
if (args.length == 0) {
System.err.println("usage: " + ExcelSaver.class.getName()
+ " [ ...]");
return;
}
for (String a : args) {
File in = new File(a);
File out = new File(a + XLS_EXTENSION);
System.out.println("reading '" + in.toString() + "', writing to '"
+ out.toString() + "'...");
if (out.exists()) {
System.out.println(" skipping '" + out
+ "', file already exists.");
} else {
try {
ExcelSaver es = new ExcelSaver();
es.convertFile(in, out);
System.out.println(" done.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public void convertFile(File in, File out) throws IOException {
ObjectInputStream is = new ObjectInputStream(new BufferedInputStream(
new FileInputStream(in)));
OutputStream os = null;
try {
// recover column names by reading file once
readColumns(is);
is.close();
is = null;
// now read a second time and produce Excel file
is = new ObjectInputStream(new BufferedInputStream(
new FileInputStream(in)));
os = new BufferedOutputStream(new FileOutputStream(out));
convertToExcelFile(is, os);
} finally {
try {
if (is != null)
is.close();
} catch (IOException ignore) {
}
try {
if (os != null)
os.close();
} catch (IOException ignore) {
}
}
}
public static final int MAX_ROWS = 65536;
public static final int MAX_COLUMNS = 256;
private boolean keepDataFile = false;
private boolean transpose = false;
private int maxParamValues = 20;
private int maxStringLength = 500;
private WritableWorkbook workbook;
private Map> paramValues;
private ArrayList columns;
private ArrayList mainExpColumns;
private final WritableCellFormat headerCellFormat;
private final WritableCellFormat defFormat;
private final WritableCellFormat intFormat;
private final WritableCellFormat floatFormat;
public ExcelSaver() {
super();
WritableFont arial10ptBold = new WritableFont(WritableFont.ARIAL, 10,
WritableFont.BOLD);
headerCellFormat = new WritableCellFormat(arial10ptBold);
defFormat = new WritableCellFormat(NumberFormats.DEFAULT);
intFormat = new WritableCellFormat(NumberFormats.INTEGER);
floatFormat = new WritableCellFormat(NumberFormats.FLOAT);
}
@Override
protected void finished(Experiment e, Map results) {
super.finished(e, results);
// convert data to Excel file
File tmp = new File(getActualResultBaseName() + SER_EXTENSION);
File out = new File(getActualResultBaseName() + XLS_EXTENSION);
try {
convertFile(tmp, out);
} catch (IOException ex) {
throw new RuntimeException(ex);
}
if (!isKeepDataFile())
tmp.delete();
}
@Override
public boolean checkBaseName(String base) {
if (!super.checkBaseName(base))
return false;
if (new File(base + XLS_EXTENSION).exists())
return false;
return true;
}
protected void convertToExcelFile(ObjectInputStream is, OutputStream os) {
// create workbook
try {
WorkbookSettings ws = new WorkbookSettings();
// ws.setLocale(new Locale("de", "DE"));
workbook = Workbook.createWorkbook(os, ws);
} catch (Exception e) {
throw new RuntimeException(e);
}
// read data a second time and add to workbook
try {
writeMainExpHeader();
if (columns.size() > 0)
writeSubExpColumnHeaders();
boolean isSubExp = true;
try {
int row = 2;
while (true) {
CellData cd = (CellData) is.readObject();
if (cd.colIdx == -3) {
isSubExp = false;
} else {
if (isSubExp) {
if (cd.colIdx == -1) {
row++;
} else {
handleSubExpData(row, cd);
}
} else
handleMainExpData(cd);
}
}
} catch (EOFException ignore) {
// System.out.println("Finished reading objects");
}
} catch (Exception e) {
throw new RuntimeException(e);
}
// All sheets and cells added. Now write out the workbook
try {
workbook.write();
workbook.close();
workbook = null;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
private void writeMainExpHeader() throws Exception {
ArrayList sortedColumns = new ArrayList(
mainExpColumns);
Collections.sort(sortedColumns, new Comparator() {
@Override
public int compare(ColumnData cd1, ColumnData cd2) {
if (cd1.isParamColumn && !cd2.isParamColumn)
return -1;
else if (!cd1.isParamColumn && cd2.isParamColumn)
return +1;
else {
return cd1.name.compareToIgnoreCase(cd2.name);
}
}
});
addHeaderCell(SHEET_NAME_MAIN, 0, 0, "parameters:");
addHeaderCell(SHEET_NAME_MAIN, 0, 1, "name");
addHeaderCell(SHEET_NAME_MAIN, 0, 2, "value");
boolean params = true;
int n = 1;
for (int i = 0; i < sortedColumns.size(); i++) {
ColumnData cd = sortedColumns.get(i);
// first result column?
if (!cd.isParamColumn && params) {
n++;
addHeaderCell(SHEET_NAME_MAIN, n, 0, "results:");
addHeaderCell(SHEET_NAME_MAIN, n, 1, "name");
addHeaderCell(SHEET_NAME_MAIN, n, 2, "value/mean");
addHeaderCell(SHEET_NAME_MAIN, n, 3, "min");
addHeaderCell(SHEET_NAME_MAIN, n, 4, "max");
addHeaderCell(SHEET_NAME_MAIN, n, 5, "stdDev");
addHeaderCell(SHEET_NAME_MAIN, n, 6, "count");
addHeaderCell(SHEET_NAME_MAIN, n, 7, "sum");
n++;
params = false;
}
cd.sortedIndex = n++;
// write parameter/result name
addCell(SHEET_NAME_MAIN, cd.sortedIndex, 1, cd.name);
}
}
private void handleMainExpData(CellData cd) throws Exception {
if (cd.colIdx >= 0) {
// parameter/result values
ColumnData col = mainExpColumns.get(cd.colIdx);
if (col.sortedIndex >= 0) {
if (cd.value instanceof SummaryStat) {
SummaryStat s = (SummaryStat) cd.value;
addCell(SHEET_NAME_MAIN, col.sortedIndex, 2, s.mean());
addCell(SHEET_NAME_MAIN, col.sortedIndex, 3, s.min());
addCell(SHEET_NAME_MAIN, col.sortedIndex, 4, s.max());
addCell(SHEET_NAME_MAIN, col.sortedIndex, 5, s.stdDev());
addCell(SHEET_NAME_MAIN, col.sortedIndex, 6, s.numObs());
addCell(SHEET_NAME_MAIN, col.sortedIndex, 7, s.sum());
} else {
addCell(SHEET_NAME_MAIN, col.sortedIndex, 2, cd.value);
}
}
} else if (cd.colIdx == -2) {
// column header, ignore
} else {
// end of row marker
assert cd.colIdx == -1;
}
}
/**
* Reads column names and values of parameter columns.
*
* @param is
* The input file which is read till the end.
*/
protected void readColumns(ObjectInputStream is) {
paramValues = new HashMap>();
columns = new ArrayList();
mainExpColumns = new ArrayList();
boolean isSubExp = true;
try {
try {
while (true) {
CellData cd = (CellData) is.readObject();
if (cd.colIdx == -1) {// ignore end of record marker
} else if (cd.colIdx == -3) { // ignore marker for begin of
// main results
isSubExp = false;
} else if (cd.colIdx == -2) { // new column
// column header
ColumnData col = (ColumnData) cd.value;
if (isSubExp)
columns.add(col);
else
mainExpColumns.add(col);
} else {
if (isSubExp) {
ColumnData col = columns.get(cd.colIdx);
if (col.isParamColumn) {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy