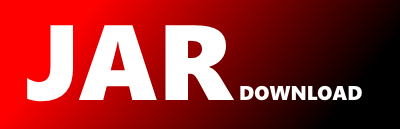
jasima.shopSim.core.PR Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2010-2015 Torsten Hildebrandt and jasima contributors
*
* This file is part of jasima, v1.2.
*
* jasima is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* jasima is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with jasima. If not, see .
*******************************************************************************/
package jasima.shopSim.core;
import jasima.shopSim.prioRules.meta.LookaheadThreshold;
import java.io.Serializable;
/**
* Abstract base class for a priority rule to be used to sequence items in a
* {@code PriorityQueue}.
*
* @author Torsten Hildebrandt
* @version "$Id: PR.java 550 2015-01-23 15:07:23Z [email protected] $"
* @see PriorityQueue
*/
public abstract class PR implements Cloneable, Serializable {
private static final long serialVersionUID = -880043612686550471L;
public static final double MIN_PRIO = PriorityQueue.MIN_PRIO;
public static final double MAX_PRIO = PriorityQueue.MAX_PRIO;
private WorkStation owner;
private PR tieBreaker;
private PR primaryRule;
private LookaheadThreshold firstLookaheadRule = null;
private boolean lookaheadRuleValid = false;
/**
* This method is called upon start of a simulation to perform any
* initializations required.
*/
public void init() {
}
/**
* This method is called by a queue before evaluating it's elements. Use it
* to do some initialization prior to calcPrio().
*
* @param q
* The current queue.
*/
public void beforeCalc(PriorityQueue extends PrioRuleTarget> q) {
}
/**
* Returns the priority value of entry
. This method has to be
* overwritten by a priority rule.
*/
public abstract double calcPrio(PrioRuleTarget entry);
/**
* If this method returns true, the machine is kept idle. This method is
* called after beforeCalc(PriorityQueue) but before
* calcPrio(PrioRuleTarget).
*/
public boolean keepIdle() {
return false;
}
@Override
public final String toString() {
String res = getName();
if (getTieBreaker() != null)
return res + "[" + getTieBreaker().toString() + "]";
else
return res;
}
public String getName() {
return getClass().getSimpleName();
}
@Override
public PR clone() throws CloneNotSupportedException {
PR clone = (PR) super.clone();
clone.firstLookaheadRule = null;
clone.lookaheadRuleValid = false;
if (getTieBreaker() != null) {
clone.tieBreaker = null;
clone.setTieBreaker(getTieBreaker().clone());
}
return clone;
}
/**
* This method simply calls {@link #clone()}, but hides the checked
* exception {@link CloneNotSupportedException}.
*/
public PR silentClone() {
try {
return clone();
} catch (CloneNotSupportedException e) {
throw new RuntimeException(e);
}
}
public WorkStation getOwner() {
return owner;
}
public void setOwner(WorkStation o) {
owner = o;
if (getTieBreaker() != null)
getTieBreaker().setOwner(o);
}
/**
* Sets the tie breaker rule to use.
*
* @param tieBreaker
* The tie-breaker to use.
*/
public void setTieBreaker(PR tieBreaker) {
if (this.tieBreaker != null) {
this.tieBreaker.primaryRule = null;
}
this.tieBreaker = tieBreaker;
if (tieBreaker != null) {
tieBreaker.primaryRule = this;
tieBreaker.setOwner(getOwner());
}
}
/**
* Convenience method to set the last tie breaker rule in a chain. The chain
* of rules is traversed until a rule without a tie breaker is found. The
* tie breaker of this rule is set to tieBreaker
.
*
* @param tieBreaker
* The tie-breaker to use.
* @return The main rule, i.e., this
.
* @see #setTieBreaker(PR)
*/
public PR setFinalTieBreaker(PR tieBreaker) {
PR pr = this;
while (pr.getTieBreaker() != null)
pr = pr.getTieBreaker();
pr.setTieBreaker(tieBreaker);
return this;
}
public PR getTieBreaker() {
return tieBreaker;
}
/**
* If this rule is used as a tie-breaker for another rule, PrimaryRule
* points to the rule this rule is the tieBreaker for, i.e.
* this.primaryRule().getTieBreaker()==this
.
*/
public PR primaryRule() {
return primaryRule;
}
public boolean arrivesTooLate(PrioRuleTarget j) {
if (!lookaheadRuleValid) {
// find highest LookaheadThreshold
firstLookaheadRule = null;
PR own = primaryRule();
while (own != null) {
if (own instanceof LookaheadThreshold)
firstLookaheadRule = (LookaheadThreshold) own;
own = own.primaryRule();
}
lookaheadRuleValid = true;
}
if (firstLookaheadRule != null)
return firstLookaheadRule.arrivesTooLate(j);
else
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy