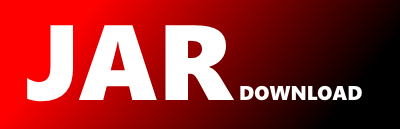
com.googlecode.javacpp.DoublePointer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javacpp Show documentation
Show all versions of javacpp Show documentation
The missing bridge between Java and native C++
/*
* Copyright (C) 2011,2012,2013 Samuel Audet
*
* This file is part of JavaCPP.
*
* JavaCPP is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 2 of the License, or
* (at your option) any later version (subject to the "Classpath" exception
* as provided in the LICENSE.txt file that accompanied this code).
*
* JavaCPP is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with JavaCPP. If not, see .
*/
package com.googlecode.javacpp;
import java.nio.DoubleBuffer;
/**
* The peer class to native pointers and arrays of double.
* All operations take into account the position and limit, when appropriate.
*
* @author Samuel Audet
*/
public class DoublePointer extends Pointer {
/**
* Allocates enough memory for the array and copies it.
*
* @param array the array to copy
* @see #put(double[])
*/
public DoublePointer(double ... array) {
this(array.length);
put(array);
}
/**
* For direct buffers, calls {@link Pointer#Pointer(Buffer)}, while for buffers
* backed with an array, allocates enough memory for the array and copies it.
*
* @param buffer the Buffer to reference or copy
* @see #put(double[])
*/
public DoublePointer(DoubleBuffer buffer) {
super(buffer);
if (buffer != null && buffer.hasArray()) {
double[] array = buffer.array();
allocateArray(array.length);
put(array);
position(buffer.position());
limit(buffer.limit());
}
}
/**
* Allocates a native double array of the given size.
*
* @param size the number of double elements to allocate
*/
public DoublePointer(int size) {
try {
allocateArray(size);
} catch (UnsatisfiedLinkError e) {
throw new RuntimeException("No native JavaCPP library in memory. (Has Loader.load() been called?)", e);
}
}
/** @see Pointer#Pointer(Pointer) */
public DoublePointer(Pointer p) { super(p); }
private native void allocateArray(int size);
/** @see Pointer#position(int) */
@Override public DoublePointer position(int position) {
return super.position(position);
}
/** @see Pointer#limit(int) */
@Override public DoublePointer limit(int limit) {
return super.limit(limit);
}
/** @see Pointer#capacity(int) */
@Override public DoublePointer capacity(int capacity) {
return super.capacity(capacity);
}
/** @return get(0) */
public double get() { return get(0); }
/** @return the i-th double value of a native array */
public native double get(int i);
/** @return put(0, d) */
public DoublePointer put(double d) { return put(0, d); }
/**
* Copies the double value to the i-th element of a native array.
*
* @param i the index into the array
* @param d the double value to copy
* @return this
*/
public native DoublePointer put(int i, double d);
/** @return get(array, 0, array.length) */
public DoublePointer get(double[] array) { return get(array, 0, array.length); }
/** @return put(array, 0, array.length) */
public DoublePointer put(double[] array) { return put(array, 0, array.length); }
/**
* Reads a portion of the native array into a Java array.
*
* @param array the array to write to
* @param offset the offset into the array where to start writing
* @param length the length of data to read and write
* @return this
*/
public native DoublePointer get(double[] array, int offset, int length);
/**
* Writes a portion of a Java array into the native array.
*
* @param array the array to read from
* @param offset the offset into the array where to start reading
* @param length the length of data to read and write
* @return this
*/
public native DoublePointer put(double[] array, int offset, int length);
/** @return asByteBuffer().asDoubleBuffer() */
@Override public final DoubleBuffer asBuffer() {
return asByteBuffer().asDoubleBuffer();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy