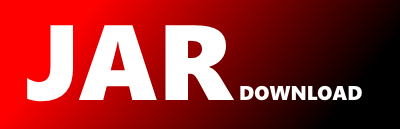
com.googlecode.javaewah.NonEmptyVirtualStorage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JavaEWAH Show documentation
Show all versions of JavaEWAH Show documentation
The bit array data structure is implemented in Java as the BitSet class. Unfortunately, this fails to scale without compression.
JavaEWAH is a word-aligned compressed variant of the Java bitset class. It uses a 64-bit run-length encoding (RLE) compression scheme.
The goal of word-aligned compression is not to achieve the best compression, but rather to improve query processing time. Hence, we try to save CPU cycles, maybe at the expense of storage. However, the EWAH scheme we implemented is always more efficient storage-wise than an uncompressed bitmap (implemented in Java as the BitSet class). Unlike some alternatives, javaewah does not rely on a patented scheme.
package com.googlecode.javaewah;
/*
* Copyright 2009-2016, Daniel Lemire, Cliff Moon, David McIntosh, Robert Becho, Google Inc., Veronika Zenz, Owen Kaser, Gregory Ssi-Yan-Kai, Rory Graves
* Licensed under the Apache License, Version 2.0.
*/
/**
* This is a BitmapStorage that can be used to determine quickly if the result
* of an operation is non-trivial... that is, whether there will be at least on
* set bit.
*
* @author Daniel Lemire and Veronika Zenz
* @since 0.4.2
*/
public class NonEmptyVirtualStorage implements BitmapStorage {
private static final NonEmptyException nonEmptyException = new NonEmptyException();
/**
* If the word to be added is non-zero, a NonEmptyException exception is
* thrown.
*
* @see com.googlecode.javaewah.BitmapStorage#addWord(long)
*/
@Override
public void addWord(long newData) {
if (newData != 0)
throw nonEmptyException;
}
/**
* If the word to be added is non-zero, a NonEmptyException exception is
* thrown.
*
* @see com.googlecode.javaewah.BitmapStorage#addWord(long)
*/
@Override
public void addLiteralWord(long newData) {
if (newData != 0)
throw nonEmptyException;
}
/**
* throws a NonEmptyException exception when number is greater than 0
*/
@Override
public void addStreamOfLiteralWords(Buffer buffer, int start, int number) {
for(int x = start; x < start + number ; ++x)
if(buffer.getWord(x)!=0) throw nonEmptyException;
}
/**
* If the boolean value is true and number is greater than 0, then it
* throws a NonEmptyException exception, otherwise, nothing happens.
*
* @see com.googlecode.javaewah.BitmapStorage#addStreamOfEmptyWords(boolean,
* long)
*/
@Override
public void addStreamOfEmptyWords(boolean v, long number) {
if (v && (number > 0))
throw nonEmptyException;
}
/**
* throws a NonEmptyException exception when number is greater than 0
*/
@Override
public void addStreamOfNegatedLiteralWords(Buffer buffer, int start,
int number) {
if (number > 0) {
throw nonEmptyException;
}
}
@Override
public void clear() {
}
/**
* Does nothing.
*
* @see com.googlecode.javaewah.BitmapStorage#setSizeInBitsWithinLastWord(int)
*/
@Override
public void setSizeInBitsWithinLastWord(int bits) {
}
static class NonEmptyException extends RuntimeException {
private static final long serialVersionUID = 1L;
/**
* Do not fill in the stack trace for this exception for
* performance reasons.
*
* @return this instance
* @see java.lang.Throwable#fillInStackTrace()
*/
@Override
public synchronized Throwable fillInStackTrace() {
return this;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy