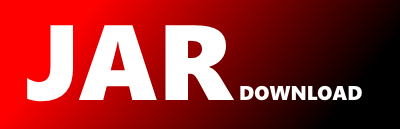
com.googlecode.javaewah32.BitCounter32 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JavaEWAH Show documentation
Show all versions of JavaEWAH Show documentation
The bit array data structure is implemented in Java as the BitSet class. Unfortunately, this fails to scale without compression.
JavaEWAH is a word-aligned compressed variant of the Java bitset class. It uses a 64-bit run-length encoding (RLE) compression scheme.
The goal of word-aligned compression is not to achieve the best compression, but rather to improve query processing time. Hence, we try to save CPU cycles, maybe at the expense of storage. However, the EWAH scheme we implemented is always more efficient storage-wise than an uncompressed bitmap (implemented in Java as the BitSet class). Unlike some alternatives, javaewah does not rely on a patented scheme.
package com.googlecode.javaewah32;
/*
* Copyright 2009-2016, Daniel Lemire, Cliff Moon, David McIntosh, Robert Becho, Google Inc., Veronika Zenz, Owen Kaser, Gregory Ssi-Yan-Kai, Rory Graves
* Licensed under the Apache License, Version 2.0.
*/
/**
* BitCounter is a fake bitset data structure. Instead of storing the actual
* data, it only records the number of set bits.
*
* @author Daniel Lemire and David McIntosh
* @since 0.5.0
*/
public final class BitCounter32 implements BitmapStorage32 {
/**
* Virtually add words directly to the bitmap
*
* @param newData the word
*/
@Override
public void addWord(final int newData) {
this.oneBits += Integer.bitCount(newData);
}
/**
* Virtually add literal words directly to the bitmap
*
* @param newData the word
*/
@Override
public void addLiteralWord(final int newData) {
this.oneBits += Integer.bitCount(newData);
}
/**
* virtually add several literal words.
*
* @param buffer the buffer wrapping the literal words
* @param start the starting point in the array
* @param number the number of literal words to add
*/
@Override
public void addStreamOfLiteralWords(Buffer32 buffer, int start, int number) {
for (int i = start; i < start + number; i++) {
addLiteralWord(buffer.getWord(i));
}
}
/**
* virtually add many zeroes or ones.
*
* @param v zeros or ones
* @param number how many to words add
*/
@Override
public void addStreamOfEmptyWords(boolean v, int number) {
if (v) {
this.oneBits += number
* EWAHCompressedBitmap32.WORD_IN_BITS;
}
}
/**
* virtually add several negated literal words.
*
* @param buffer the buffer wrapping the literal words
* @param start the starting point in the array
* @param number the number of literal words to add
*/
@Override
public void addStreamOfNegatedLiteralWords(Buffer32 buffer, int start,
int number) {
for (int i = start; i < start + number; i++) {
addLiteralWord(~buffer.getWord(i));
}
}
@Override
public void clear() {
this.oneBits = 0;
}
/**
* As you act on this class, it records the number of set (true) bits.
*
* @return number of set bits
*/
public int getCount() {
return this.oneBits;
}
@Override
public void setSizeInBitsWithinLastWord(int bits) {
// no action
}
private int oneBits;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy