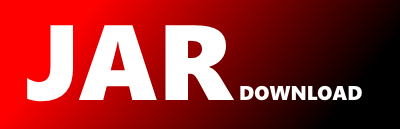
org.jcamp.units.OffsetUnit Maven / Gradle / Ivy
package org.jcamp.units;
/**
* unit created by adding an offset to another unit
* @author Thomas Weber
*/
public final class OffsetUnit extends Unit {
Unit unit;
double offset;
String symbol;
String name;
/**
* cloning.
*
* @return java.lang.Object
*/
public Object clone() {
OffsetUnit unit = (OffsetUnit) super.clone();
unit.unit = (Unit) this.unit.clone();
return unit;
}
/**
* ScaledUnit constructor comment.
* @param identifier java.lang.String
*/
public OffsetUnit(BaseUnit unit, double offset, String name, String symbol) {
this((Unit) unit, offset, name, symbol);
}
/**
* ScaledUnit constructor comment.
* @param identifier java.lang.String
*/
public OffsetUnit(DerivedUnit unit, double offset, String name, String symbol) {
this((Unit) unit, offset, name, symbol);
}
/**
* ScaledUnit constructor comment.
* @param identifier java.lang.String
*/
public OffsetUnit(ScaledUnit unit, double offset, String name, String symbol) {
this((Unit) unit, offset, name, symbol);
}
/**
* ScaledUnit constructor comment.
* @param identifier java.lang.String
*/
private OffsetUnit(Unit unit, double offset, String name, String symbol) {
super(name, symbol);
this.unit = unit;
this.offset = offset;
this.name = name;
this.symbol = symbol;
}
/**
* convert value from unit thatUnit
to this unit.
* @param value double
* @param thatUnit Unit
* @return double
*/
public double convertFrom(double value, Unit thatUnit) throws UnitException {
if (isConvertibleTo(thatUnit)) {
if ((thatUnit instanceof ScaledUnit)
|| (thatUnit instanceof BaseUnit)
|| (thatUnit instanceof DerivedUnit))
return value * thatUnit.getScaleFactor() / getScaleFactor() - offset;
else
return thatUnit.convertTo(value, this);
} else
throw new UnitException("units not convertible");
}
/**
* convert value to unit thatUnit
from this unit.
* @param value double
* @param thatUnit Unit
* @return double
*/
public double convertTo(double value, Unit thatUnit) throws UnitException {
if (isConvertibleTo(thatUnit)) {
if ((thatUnit instanceof ScaledUnit)
|| (thatUnit instanceof BaseUnit)
|| (thatUnit instanceof DerivedUnit))
return value * thatUnit.getScaleFactor() / getScaleFactor() + offset;
else
return thatUnit.convertFrom(value, this);
} else
throw new UnitException("units not convertible");
}
/**
* getDefinition method comment.
*/
public java.lang.String getName() {
return name;
}
/**
* gets quantity.
* @return String
*/
public java.lang.String getQuantity() {
return unit.getQuantity();
}
/**
* gets scale factor.
* @return double
*/
public double getScaleFactor() {
return unit.getScaleFactor();
}
/**
* getDefinition method comment.
*/
public java.lang.String getSymbol() {
return symbol;
}
/**
* checks if unit is convertible to unit thatUnit
.
* @param thatUnit Unit
* @return boolean
*/
public boolean isConvertibleTo(Unit thatUnit) {
if (thatUnit instanceof OffsetUnit)
return unit.isConvertibleTo(((OffsetUnit) thatUnit).unit);
else if (thatUnit instanceof ScaledUnit)
return unit.isConvertibleTo(((ScaledUnit) thatUnit).unit);
else if (thatUnit instanceof BaseUnit)
return unit.isConvertibleTo((BaseUnit) thatUnit);
else if (thatUnit instanceof DerivedUnit)
return unit.isConvertibleTo((DerivedUnit) thatUnit);
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy