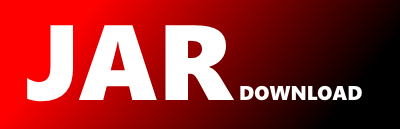
org.ocap.application.OcapIxcPermission.html Maven / Gradle / Ivy
OcapIxcPermission
Overview
Package
Class
Tree
Deprecated
Index
Help
PREV CLASS
NEXT CLASS
FRAMES
NO FRAMES
SUMMARY: NESTED | FIELD | CONSTR | METHOD
DETAIL: FIELD | CONSTR | METHOD
org.ocap.application
Class OcapIxcPermission
java.lang.Object
java.security.Permission
java.security.BasicPermission
org.ocap.application.OcapIxcPermission
- All Implemented Interfaces:
- java.io.Serializable, java.security.Guard
public final class OcapIxcPermission
- extends java.security.BasicPermission
This class represents access to the inter-xlet communication registry. An OcapIxcPermission consists of a name specification and a action specifying what can be done with those names.
The name specification is a superset of the name passed into the IxcRegistry
methods such as IxcRegistry.bind(javax.tv.xlet.XletContext, java.lang.String, java.rmi.Remote)
and IxcRegistry.lookup(javax.tv.xlet.XletContext, java.lang.String)
.
Valid names are composed of fields delimited by "/" characters, with each field
specifying a particular value (e.g., OID). The following grammar defines the name
format:
NAME = "*" | "/" SCOPE "/" SIGNED "/" OID "/" AID "/" BINDNAME SCOPE = "*" | "global" | "ixc" | "service-" CONTEXT CONTEXT = "*" | context-id SIGNED = "*" | "signed" | "unsigned" OID = "*" | oid AID = "*" | aid BINDNAME = "*" | bindname | bindname "*"Where
"*"
specifies a wildcard character.
Where context-id is a platform-specific unique identifier for a service
context;
oid and aid are the organization
and
application
identifiers of the binding application as
converted by Integer.toHexString(int)
;
and bindname is the application-defined name given at bind-time.
- "*" as the entire name string will match any other name
- "/*/*/*/*/* is equivalent to "*"
- "/*/*/1a/4abc/*" will match names in any scope,
published by an application with an OID of
1a
and AID of4abc
. - "/*/signed/*/VODApi" will match any object bound by a signed application with an ixcname of "VODApi".
bind
and
lookup
methods of IxcRegistry
.
The actions string is converted to lowercase before processing.
- See Also:
- Serialized Form
Constructor Summary | |
---|---|
OcapIxcPermission(java.lang.String name,
java.lang.String actions)
Creates a new OcapIxcPermission object with the specified name and actions. |
Method Summary | |
---|---|
boolean |
equals(java.lang.Object obj)
Checks two OcapIxcPermission objects for equality. |
java.lang.String |
getActions()
Returns the "canonical string representation" of the actions. |
int |
hashCode()
Returns the hash code value for this object. |
boolean |
implies(java.security.Permission p)
Checks if this OcapIxcPermission "implies" the specified permission. |
java.security.PermissionCollection |
newPermissionCollection()
Returns a new PermissionCollection object for storing OcapIxcPermission objects. |
Methods inherited from class java.security.Permission |
---|
checkGuard, getName, toString |
Methods inherited from class java.lang.Object |
---|
clone, finalize, getClass, notify, notifyAll, wait, wait, wait |
Constructor Detail |
---|
OcapIxcPermission
public OcapIxcPermission(java.lang.String name, java.lang.String actions)
- Creates a new OcapIxcPermission object with the specified name and actions.
The name specification is a superset of the name passed into the
IxcRegistry
methods such asIxcRegistry.bind(javax.tv.xlet.XletContext, java.lang.String, java.rmi.Remote)
andIxcRegistry.lookup(javax.tv.xlet.XletContext, java.lang.String)
. See theclass description
for the specification of the name string.The actions specification is comprised of a single action specified by one of two keywords: "bind" or "lookup". These correspond to the
bind
andlookup
methods ofIxcRegistry
. The actions string is converted to lowercase before processing.- Parameters:
name
- The name specification for exported/imported objectsactions
- The action string
Method Detail |
---|
equals
public boolean equals(java.lang.Object obj)
- Checks two OcapIxcPermission objects for equality.
Check that other is an OcapIxcPermission, and has the same name and actions as this object.
- Overrides:
equals
in classjava.security.BasicPermission
- Parameters:
obj
- the object we are testing for equality with this object- Returns:
- true if obj is an OcapIxcPermission, and has the same name and actions as this OcapIxcPermission object.
getActions
public java.lang.String getActions()
- Returns the "canonical string representation" of the actions.
That is, this method always returns present actions in the following order: bind, lookup.
For example, if this OcapIxcPermission object allows both bind and lookup actions, a call
to getActions will return the string "bind,lookup".
- Overrides:
getActions
in classjava.security.BasicPermission
- Returns:
- the canonical string representation of the actions
hashCode
public int hashCode()
- Returns the hash code value for this object.
- Overrides:
hashCode
in classjava.security.BasicPermission
- Returns:
- a hash code value for this object.
implies
public boolean implies(java.security.Permission p)
- Checks if this OcapIxcPermission "implies" the specified permission.
More specifically, this method returns true if:
- p is an instanceof OcapIxcPermission
- p's actions are a proper subset of this object's actions, and
- p's name is implied by this object's name.
The rules for determining if this object's name implies p's name are as follows:
- Where p's name is exactly the same as this object's name, then it is implied.
- The name
"*"
and"/*/*/*/*/*"
both imply all possible names. - Where this object's name includes a wildcard for a field (
"*"
), then all possible values for that field are implied. - Where this object's name includes a field that ends in a wildcard (e.g.,
service-*
) then all possible values for that field starting with the non-wildcard portion are implied.
For example,
"/service-*/signed/abc/4001/*"
implies"/service-1234/signed/abc/4001/VODObject"
.An
OcapIxcPermission
may also imply anIxcPermission
. That is, this method will also return true if:- p is an instanceof IxcPermission
- p's actions are a proper subset of this object's actions, and
- p's name is implied by this object's name.
The rules for determining if this object's name implies an
IxcPermission
name are the same as detailed above except that a translation of theIxcPermission
name to theOcapIxcPermission
is applied first. The following table shows how such a mapping SHALL be applied:IxcPermission name OcapIxcPermission name "*" "*" "dvb:/*" "*" "dvb:/signed/*" "/global/signed/*/*/* "dvb:/service/id/signed/*" "/service-id/signed/*/*/* "dvb:/ixc/*" "/ixc/*/*/*/* "dvb:/signed/OID/*" "/global/signed/OID/*/* "dvb:/service/id/signed/OID/*" "/service-id/signed/OID/*/* "dvb:/ixc/OID/*" "/ixc/*/OID/*/* "dvb:/signed/OID/AID/*" "/global/signed/OID/AID/* "dvb:/service/id/signed/OID/AID/*" "/service-id/signed/OID/AID/* "dvb:/ixc/OID/AID/*" "/ixc/*/OID/AID/* "dvb:/signed/OID/AID/name*" "/global/signed/OID/AID/name* "dvb:/service/id/signed/OID/AID/name*" "/service-id/signed/OID/AID/name* "dvb:/ixc/OID/AID/name*" "/ixc/*/OID/AID/name* Any
IxcPermission
name that cannot be mapped cannot be implied.- Overrides:
implies
in classjava.security.BasicPermission
- Parameters:
p
- the permission to check against- Returns:
- true if the specified permission is implied by this object, false if not.
newPermissionCollection
public java.security.PermissionCollection newPermissionCollection()
- Returns a new PermissionCollection object for storing OcapIxcPermission objects.
OcapIxcPermission objects must be stored in a manner that allows them to be inserted into the collection in any order, but that also enables the PermissionCollection implies method to be implemented in an efficient (and consistent) manner.
- Overrides:
newPermissionCollection
in classjava.security.BasicPermission
- Returns:
- a new PermissionCollection object suitable for storing OcapIxcPermissions.
|
|||||||||
PREV CLASS NEXT CLASS | FRAMES NO FRAMES | ||||||||
SUMMARY: NESTED | FIELD | CONSTR | METHOD | DETAIL: FIELD | CONSTR | METHOD |